简单选择排序
找到数组中的最大值或最小值时再将值进行交换

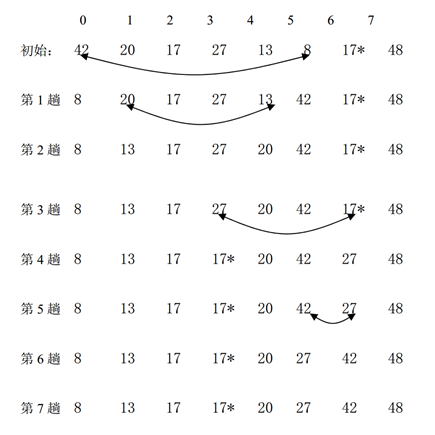
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 简单选择排序
{
class Program
{
static void Main(string[] args)
{
int[] array = new int[] { 42, 20, 99, 12, 44, 4, 66, 88, 24, 93, 1, 22 };//需要排序的数组
Stopwatch watch = new Stopwatch();//创建StopWatch类的对象
watch.Start();//调用类中的start函数开始计时
Bubbing(array);//用冒泡排序算法对数组进行排序
watch.Stop();//调用StopWatch类中的stop函数,终止计时
Console.WriteLine(watch.Elapsed);//输出直接插入排序的用时
foreach (var item in array)//输出已近排序好的数组
{
Console.WriteLine(item);
}
Console.ReadKey();
}
/// <summary>
/// 简单选择排序,找到数组中的最大值或最小值时在交换
/// </summary>
/// <param name="array"></param>
private static void Bubbing(int[] array)
{
for (int i = 0; i < array.Length-1; i++)
{
int index = i;
int temp = array[i];
for (int j = i+1; j < array.Length; j++)
{
if(temp>array[j])
{
temp = array[j];
index = j;//记录要交换值的下标,因为暂时还不确定这个值是否为最小值,所以记录下标
}
}
if(temp!=i)//找到数组的最小值,把最小值放在array[i]位置
{
array[index] = array[i];
array[i] = temp;
}
}
}
}
}
堆排序:将完全二叉树构造成大顶堆或小顶堆。
实现大顶堆:在每个顶推的父节点和子节点中找到最大值,放入父节点中(实质是大于父节点的子节点中拿出最大的子节点与父节点交换)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 简单选择排序
{
class Program
{
static void Main(string[] args)
{
int[] array = new int[] { 49,38,65,97,76,13,27,49};
HeapSort(array);
foreach (var item in array)
{
Console.WriteLine(item);
}
Console.ReadKey();
}
private static void HeapSort(int[] array)
{
for (int i = array.Length/2; i >0; i--)//对每个分支节点进行堆排序
{
int temp = i;
int index = i;
while(true)
{
int leftChild = 2 * temp;
int rightChild = 2 * temp + 1;
if(rightChild<=array.Length&& array[rightChild - 1] > array[temp - 1])
{
temp = rightChild;
}
if(leftChild<=array.Length&& array[leftChild - 1] > array[temp - 1])
{
temp = leftChild;
}
if(temp!=index)//子节点大于父节点,所以交换子节点与父节点
{
int value = array[index-1];
array[index-1] = array[temp-1];
array[temp-1] = value;
index = temp;
}
else
{
break;
}
}
}
}
}
}