知识点:
OSI参考模型和TCP/IP模型
TCP三次握手
TCP、UDP、URL编程
浏览器输入网址到显示页面的整个过程
参考:https://www.cnblogs.com/midiyu/p/7875574.html
一 :OSI参考模型和TCP/IP模型
网络协议
二 :TCP三次握手
三 :TCP、UDP、URL编程
(1)TCP编程
实例1
客户端发送消息到服务端,服务端接收消息后,发送消息返回给客户端
//客户端给服务端发送消息,服务端接收到消息,并且发消息返回给客户端
public class TCPTest1 {
//客户端
@Test
public void Client (){
Socket socket=null;
OutputStream os=null;
InputStream is=null;
try {
//1.创建一个Socket对象,通过构造器指明服务端IP地址,以及接收程序的端口号
socket=new Socket(InetAddress.getByName("169.254.77.87"),8082);
//2.getOutputStream():发送数据,方法返回OutputStream对象
os=socket.getOutputStream();
//3.输出内容
os.write("我是客户端!".getBytes());
//shutdownOutput():显示告诉服务器端发送完毕
socket.shutdownOutput();
//收到来自服务端的消息
is=socket.getInputStream();
byte[] b=new byte[40];
int len;
while ((len=is.read(b))!=-1){
String str=new String(b,0,len);
System.out.println(str);//输出 "我是服务端,已收到消息!"
}
} catch (IOException e) {
e.printStackTrace();
}finally {
//4.关闭流和socket对象
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
//服务端
@Test
public void Server(){
ServerSocket ss=null;
Socket socket=null;
InputStream is=null;
OutputStream os=null;
try {
//1.创建一个ServerSocket对象,构造器指明自身端口
ss=new ServerSocket(8082);
//2.调用accept()方法,返回一个Socket对象
socket=ss.accept();
//3.调用socket对象的getInputStream()获取一个从客户端发送过来的输入流
is=socket.getInputStream();
//4.对获取的输入流操作
byte[] b=new byte[20];
int len;
while ((len=is.read(b))!=-1){
String str=new String(b,0,len);
System.out.println(str);//输出 "我是客户端!"
}
//获得客户端IP地址
System.out.println("消息来自于:"+socket.getInetAddress().getHostAddress());
//给客户端发送消息
os=socket.getOutputStream();
os.write("我是服务端,已收到消息!".getBytes());
} catch (IOException e) {
e.printStackTrace();
}finally {
//5.关闭相应的流和ServerSocket和Socket对象
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(ss!=null){
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
控制台结果
server端输出:
client端输出 :
实例2
客户端发送文件到服务端,服务端接收文件保存到本地,并发送“文件接收成功”消息到客户端
/客户端发送文件到服务端,服务端接收文件保存到本地,并发送“成功消息”到客户端
public class TCPTest2 {
@Test
public void Client(){
Socket socket=null;
FileInputStream fis=null;
BufferedInputStream br=null;
OutputStream os=null;
InputStream is=null;
try {
//发送文件到服务端
socket=new Socket(InetAddress.getByName("169.254.77.87"),8087);
fis=new FileInputStream(new File("E:\test\io\1.png"));
br=new BufferedInputStream(fis);
os=socket.getOutputStream();
byte[] b=new byte[1024];
int len;
while ((len=br.read(b))!=-1){
os.write(b,0,len);
}
os.flush();/清空缓冲区内的数据,最后一次
socket.shutdownOutput(); //显示告诉服务器端发送完毕
//接收服务端返回的消息
is=socket.getInputStream();
byte[] b1=new byte[1024];
int len1;
while ((len1=is.read(b1))!=-1){
String str=new String(b1,0,len1);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (br!=null){
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fis!=null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fis!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void Server(){
ServerSocket serverSocket=null;
Socket socket=null;
InputStream is=null;
OutputStream os=null;
FileOutputStream fos=null;
BufferedOutputStream bos=null;
try {
//读取客户端发送的流
serverSocket=new ServerSocket(8087);
socket=serverSocket.accept();
is=socket.getInputStream();
//文件写入本地
fos=new FileOutputStream(new File("E:\test\io\5.png"));
bos=new BufferedOutputStream(fos);
byte[] b=new byte[1024];
int len;
while ((len=is.read(b))!=-1){
bos.write(b,0,len);
}
bos.flush();
//输出客户端信息
System.out.println("来自客户端"+socket.getInetAddress().getHostAddress()+"的文件!");
//返回客户端发送成功消息
os=socket.getOutputStream();
os.write("服务端文件接收成功!".getBytes());
} catch (IOException e) {
e.printStackTrace();
}finally {
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bos!=null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos!=null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(serverSocket!=null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
控制台结果
server端输出:

cilent端输出:
实例3
服务端接收多个客户端的请求,并打印请求内容
//服务端接收多个客户端的请求
public class TCP_Thread_Test1 {
@Test
public void server(){ //服务端
int clientNo=1;
ServerSocket serverSocket=null;
try {
serverSocket=new ServerSocket(7071);
//创建线程池
ExecutorService exec= Executors.newCachedThreadPool(); //Executors创建可缓存的线程池 https://www.cnblogs.com/shuaifing/p/11580337.html
while(true){
Socket socket=serverSocket.accept();
exec.execute(new SingleServer(socket,clientNo));//线程池执行线程
clientNo++;
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if(serverSocket!=null){
try {
serverSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void client1(){ //客户端1
Socket socket=null;
OutputStream os=null;
try {
socket=new Socket(InetAddress.getByName("192.168.127.1"),7071);
BufferedOutputStream bos=new BufferedOutputStream(os);
os=socket.getOutputStream();
os.write("我是客户端:".getBytes());
} catch (IOException e) {
e.printStackTrace();
}finally {
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void client2(){ //客户端2
Socket socket=null;
OutputStream os=null;
try {
socket=new Socket(InetAddress.getByName("192.168.127.1"),7071);
BufferedOutputStream bos=new BufferedOutputStream(os);
os=socket.getOutputStream();
os.write("我是客户端:".getBytes());
} catch (IOException e) {
e.printStackTrace();
}finally {
if(os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket!=null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
class SingleServer implements Runnable{
private Socket socket;
private int clientNo;
public SingleServer(Socket socket,int clientNo){
this.socket=socket;
this.clientNo=clientNo;
}
@Override
public void run() {
BufferedInputStream bis=null;
try {
bis=new BufferedInputStream(socket.getInputStream());
byte[] b=new byte[1024];
int len;
while ((len=bis.read(b))!=-1){
String str=new String(b,0,len);
System.out.println(str+clientNo);//打印接收的客户端信息
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if(bis!=null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
控制台结果:
server端输出:
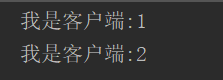
(2)UDP编程
实例1
发送端发送消息到接收端
//UDP编程
public class UDPTest {
@Test
public void send(){
DatagramSocket socket=null;//socket套接字
DatagramPacket packet=null;
try {
socket=new DatagramSocket();
byte[] b="发送端发送的数据!".getBytes();
packet=new DatagramPacket(b,0,b.length, InetAddress.getByName("169.254.77.87"),8084);//DatagramPacket数据包
socket.send(packet);
} catch (Exception e) {
e.printStackTrace();
}finally {
socket.close();
}
}
@Test
public void receive(){
DatagramSocket ds=null;
try {
ds=new DatagramSocket(8084);
byte[] b=new byte[40];
DatagramPacket dp=new DatagramPacket(b,0,b.length);
ds.receive(dp);
String str=new String(dp.getData(),0,dp.getLength());
System.out.println("接收端收到消息:"+str);
} catch (Exception e) {
e.printStackTrace();
}finally {
ds.close();
}
}
}
控制台结果
receive端输出:
(2)URL编程
实例1
获取服务端资源,控制输出,同时输出数据到文件中
public class URLTest {
public static void main(String[] args) throws IOException {
URL url=new URL("http://localhost:8087/examples/hello.txt");
//获取服务端资源
InputStream is=null;
try {
is=url.openStream();//网上读取数据
byte [] b=new byte[20];
int len;
while ((len=is.read(b))!=-1){
String str=new String(b,0,len);
System.out.println(str);
}
} catch (IOException e) {
e.printStackTrace();
}finally {
if(is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//输出数据 URLConnection
URLConnection urlcon=url.openConnection();
InputStream is1=urlcon.getInputStream();
FileOutputStream fos=new FileOutputStream(new File("E:/test/io/11.txt"));
byte [] b1=new byte[20];
int len1;
while ((len1=is1.read(b1))!=-1){
fos.write(b1,0,len1);
}
fos.close();
is1.close();
}
}