一、集合概述
1. Java中的集合是工具类,可以存储任意数量的具有共同属性的对象。
与数组不同,集合的长度可以动态改变,所以数组适合去存储固定长度的数据,集合适合去存储不固定长度的数据。
2. 集合的应用场景:
1)无法预测存储数据的数量。
2)存储具有一对一关系的数据(比如某件商品,其商品编号只对应这件商品)。
3)需要进行数据的增删。
4)数据重复问题。
二、集合框架的体系结构
1、collection:存储类的对象
Collection三个子接口:
1)List(序列)
存放的数据有序、可以重复
List常用实现类ArrayList(长度动态增长的数组)
2)Queue(队列)
存放的数据有序、可以重复
Queue实现类LinkedList(同时实现了List接口表示的是链表的内容)
3)Set(集)
无序、不可以重复
Set实现类HashSet(哈希集)
2、Map
键值对 Map实现类HashMap(哈希表,存储为键值对<key,value>)
三、List
1、List概述
1. List中元素有序且可以重复,称为序列。
2. List可以精确地控制每个元素的插入位置,或删除某个位置的元素。
3. List的两个主要实现类:ArrayList和LinkedList。
4. ArrayList底层是由数组实现的,长度动态增长。ArrayList中的元素可以为null且可以有多个。
在列表尾部插入或删除数据非常有效,但是在中间则需要耗费较多资源。所以更适合查找和更新元素。
2、list的常用方法
List list = new ArrayList();
list.contains();用来判断列表中是否包含要查找的对象,括号中写对象名
list.add();添加元素
list.remove();移除元素/移除元素有两种方法1.根据下标移除2.根据要移除的元素内容移除
list.get();获取列表中指定位置处的元素
list.size();获取列表长度
举例:

import java.util.ArrayList; import java.util.List; public class ListDemo1 { public static void main(String[] args) { // 用ArrayList存储编程语言的名称,并输出 List list=new ArrayList(); list.add("Java"); list.add("C"); list.add("C++"); list.add("Go"); list.add("swift"); //输出列表中元素的个数 System.out.println("列表中元素的个数为:"+list.size()); //遍历输出所有编程语言 System.out.println("**************************************"); for(int i=0;i<list.size();i++){ System.out.print(list.get(i)+","); } //移除列表中的C++ System.out.println(); //list.remove(2); list.remove("C++"); System.out.println("**************************************"); System.out.println("移除C++以后的列表元素为:"); for(int i=0;i<list.size();i++){ System.out.print(list.get(i)+","); } } }
3、List代码实例:添加公告
1、 需求
--公告的添加和显示
--在指定位置处插入公告
--删除公告 --修改公告
2、分析
1)公告类属性: --编号 id --标题 title -- 创建人 creator --创建时间 createTime
2)公告类方法 --构造方法 --获取和设置属性值的方法

import java.util.Date; public class Notice { private int id;//ID private String title;//标题 private String creator;//创建人 private Date createTime;//创建时间 public Notice(int id, String title, String creator, Date createTime) { super(); this.id = id; this.title = title; this.creator = creator; this.createTime = createTime; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getCreator() { return creator; } public void setCreator(String creator) { this.creator = creator; } public Date getCreateTime() { return createTime; } public void setCreateTime(Date createTime) { this.createTime = createTime; } }

import java.util.ArrayList; import java.util.Date; public class NoticeTest { public static void main(String[] args) { // 创建Notice类的对象,生成三条公告 Notice notice1 = new Notice(1, "欢迎来到学校!", "管理员", new Date()); Notice notice2 = new Notice(2, "请同学们按时提交作业!", "老师", new Date()); Notice notice3 = new Notice(3, "考勤通知!", "老师", new Date()); // 添加公告 ArrayList noticeList = new ArrayList(); noticeList.add(notice1); noticeList.add(notice2); noticeList.add(notice3); // 显示公告 System.out.println("公告的内容为:"); for (int i = 0; i < noticeList.size(); i++) { System.out.println(i + 1 + ":" + ((Notice) (noticeList.get(i))).getTitle());//这里需要强制转换,加上Notice } System.out.println("**************************************"); // 在第一条公告后面添加一条新公告 Notice notice4 = new Notice(4, "在线编辑器可以使用啦!", "管理员", new Date()); noticeList.add(1, notice4); // 显示公告 System.out.println("公告的内容为:"); for (int i = 0; i < noticeList.size(); i++) { System.out.println(i + 1 + ":" + ((Notice) (noticeList.get(i))).getTitle()); } System.out.println("**************************************"); // 删除按时提交作业的公告 noticeList.remove(2); // 显示公告 System.out.println("删除公告后的内容为:"); for (int i = 0; i < noticeList.size(); i++) { System.out.println(i + 1 + ":" + ((Notice) (noticeList.get(i))).getTitle()); } //将第二条公告改为:Java在线编辑器可以使用啦! System.out.println("**************************************"); //修改第二条公告中title的值 notice4.setTitle("Java在线编辑器可以使用啦!"); noticeList.set(1, notice4); System.out.println("修改后公告的内容为:"); for (int i = 0; i < noticeList.size(); i++) { System.out.println(i + 1 + ":" + ((Notice) (noticeList.get(i))).getTitle()); } } }
四、set
1、set概述
Set是元素无序并且不可以重复的集合,被称为集,Set是接口。
HashSet是Set的一个重要实现类,称为哈希集
HashSet中的元素无序并且不可以重复
HashSet中只允许一个null元素 具有良好的存取和查找性能
HashSet的底层其实是HashMap。 HashSet默认容量是16,默认的加载因子为0.75
2、补充知识:迭代器 ITerator
迭代器:Iterator接口可以以统一的方式对各种集合元素进行遍历 hasNext():检测集合中是否还有下一个元素 next():返回集合中的下一个元素
迭代器使用完会释放空间,再次使用需要给到新的引用 把集合添加到迭代器中:(导入包)Iterator 迭代器名=集合名.iterator()方法 遍历迭代器并输出:
代码实例:

import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class WordDemo { public static void main(String[] args) { // 将英文单词添加到HashSet中 Set set = new HashSet(); // 向集合中添加元素 set.add("blue"); set.add("red"); set.add("black"); set.add("yellow"); set.add("white"); // 显示集合的内容 System.out.println("集合中的元素为:"); Iterator it = set.iterator(); // 遍历迭代器并输出元素 while (it.hasNext()) { System.out.print(it.next() + " "); } System.out.println(); // 在集合中插入一个新的单词 // set.add("green"); set.add("white"); it = set.iterator(); // 遍历迭代器并输出元素 System.out.println("**************************"); System.out.println("插入重复元素后的输出结果为:"); while (it.hasNext()) { System.out.print(it.next() + " "); } //插入失败,但是不会报错 } }
3、HashSet实例:宠物猫信息管理
1)需求与提示

*需求 --添加和显示宠物猫信息 --查找某只宠物猫的信息并输出 --修改宠物猫的信息 --删除宠物猫信息 宠物猫类的定义: *属性 --名字 name --年龄 month --品种 species *类中方法的定义具体如截图所示 方法: 构造方法 获取和设置属性值的方法 其他方法:tostring方法
2)代码

public class Cat { private String name; //名字 private int month; //年龄 private String species;//品种 //构造方法 public Cat(String name, int month, String species) { super(); this.name = name; this.month = month; this.species = species; } //getter与setter方法 public String getName() { return name; } public void setName(String name) { this.name = name; } public int getMonth() { return month; } public void setMonth(int month) { this.month = month; } public String getSpecies() { return species; } public void setSpecies(String species) { this.species = species; } /** * toString()方法是Object类的方法,我们定义的所有类都是Object类的子类; 如果输出该类的对象,就会自动调用toString()方法,这样会输出该对象的地址; 如果想输出对象的内容,可以在该类中重写toString()方法 *改写toString方法 * 1重写Object类的toString()方法,输出对象时,就可以自动调用toString()方法。 * 这个方法只是为了方便输出,比如System.out.println(xx),括号里面的“xx”如果不是String类型的话,就自动调用xx的toString()方法。 我们在Cat中重写toString方法,输出Cat对象的各个属性值。 总而言之,它只是sun公司开发java的时候为了方便所有类的字符串操作而特意加入的一个方法。 * @return */ @Override public String toString() { return "[姓名:" + name + ", 年龄:" + month + ", 品种:" + species + "]"; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + month; result = prime * result + ((name == null) ? 0 : name.hashCode()); result = prime * result + ((species == null) ? 0 : species.hashCode()); return result; } @Override public boolean equals(Object obj) { //判断对象是否相等,相等则返回true,不用继续比较属性了 if(this==obj) return true; //判断obj是否是Cat类的对象 if(obj.getClass()==Cat.class){ Cat cat=(Cat)obj; return cat.getName().equals(name)&&(cat.getMonth()==month)&&(cat.getSpecies().equals(species)); } return false; } }

import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class CatTest { public static void main(String[] args) { // 定义宠物猫对象 Cat huahua = new Cat("花花", 12, "英国短毛猫"); Cat fanfan = new Cat("凡凡", 3, "中华田园猫"); // 将宠物猫对象放入HashSet中 Set<Cat> set = new HashSet<Cat>(); set.add(huahua); set.add(fanfan); // 显示宠物猫信息 Iterator<Cat> it = set.iterator();//使用迭代器 while (it.hasNext()) { System.out.println(it.next()); } // 再添加一个与花花属性一样的猫 Cat huahua01 = new Cat("花花", 12, "英国短毛猫"); set.add(huahua01); System.out.println("**********************************"); System.out.println("添加重复数据后的宠物猫信息:"); it = set.iterator(); while (it.hasNext()) { System.out.println(it.next()); } System.out.println("**********************************"); // 重新插入一个新宠物猫 Cat huahua02 = new Cat("花花二代", 2, "英国短毛猫"); set.add(huahua02); System.out.println("添加花花二代后的宠物猫信息:"); it = set.iterator(); while (it.hasNext()) { System.out.println(it.next()); } System.out.println("**********************************"); // 在集合中查找花花的信息并输出 if (set.contains(huahua)) { System.out.println("花花找到了!"); System.out.println(huahua); } else { System.out.println("花花没找到!"); } // 在集合中使用名字查找花花的信息 System.out.println("**********************************"); System.out.println("通过名字查找花花信息"); boolean flag = false; Cat c = null; it = set.iterator(); while (it.hasNext()) { c = it.next(); if (c.getName().equals("花花")) { flag = true;// 找到了 break; } } if (flag) { System.out.println("花花找到了"); System.out.println(c); } else { System.out.println("花花没找到"); } // 删除花花二代的信息并重新输出 for (Cat cat : set) { if ("花花二代".equals(cat.getName())) { set.remove(cat); break; } } System.out.println("**********************************"); System.out.println("删除花花二代后的数据"); for(Cat cat:set){ System.out.println(cat); } //删除集合中的所有宠物猫信息 System.out.println("**********************************"); boolean flag1=set.removeAll(set); if(set.isEmpty()){ System.out.println("猫都不见了。。。"); }else{ System.out.println("猫还在。。。"); } } }
4、toString方法+重写equals和hashcode方法
1)为什么要在Cat类中重写toString方法呢?
这个方法只是为了方便输出,比如System.out.println(xx),括号里面的“xx”如果不是String类型的话,就自动调用xx的toString()方法。
我们在Cat中重写toString方法,输出Cat对象的各个属性值。
总而言之,它只是sun公司开发java的时候为了方便所有类的字符串操作而特意加入的一个方法。
2)HashSet中,是无法添加相同的对象的。那么什么是相同的对象?
问:用相同的属性对对象进行初始化,它们是相同的对象吗?
如:
Cat cat1 = new Cat("花花", 12, "英国短毛猫");
Cat cat2 = new Cat("花花", 12, "英国短毛猫");
答:不是。
所以这样的两个对象,是可以加入的HashSet中的。这往往与我们的期望是不符的,我们认为他们应该是"相同的",不应该重复加入。
程序是通过调用hashCode和equals两个方法来判断对象是否相等的。
所以,对于上面提到的问题,我们可以通过重写这两个方法来实现我们需求。
equals方法思路:
1)先判断对象是否相等,相等返回true,不用继续比较属性;
2)判断obj是否为目标类的对象,若是则通过强制类型转换为目标类对象后继续比较属性值是否相同;
3)以上都不满足则返回false:
@Override public boolean equals(Object obj) { //判断对象是否相等,相等则返回true,不用继续比较属性了 if(this==obj) return true; //判断obj是否是Cat类的对象 if(obj.getClass()==Cat.class){ Cat cat=(Cat)obj; return cat.getName().equals(name)&&(cat.getMonth()==month)&&(cat.getSpecies().equals(species)); } return false; }
3)自动生成toString、hashCode和equals
右键Generate
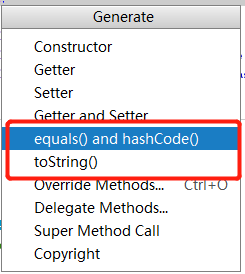
一般情况下hashcode直接用equals需要手动修改下
4)其他
hashCode方法是对类中的数据再分类,例如:分成了3份, 不重写也没关系。
如果hashCode()值相同,还需要根据equals()方法判断是否为同一个对象。
hashCode的值不同时,则两个对象必定不同
五、Map
1、Map概述
1)Map中的数据是以键值对(key-value)的形式存储的;
2)key-value以Entry类型的对象实例存在;Entry是一个接口
3)可以通过key值快速地查找value;
4)一个映射不能包含重复的键;
5)每个键最对只能映射到一个值。
2. HashMap
1)基于哈希表的Map接口的实现;
2)允许使用null值和null键;
3)key值不允许重复;
4)HashMap中的Entry对象是无序排列的。
3、 HashMap打印输出
Map 类型,Map 是一个接口,无法进行实例化操作,可以定义一个引用指向HashMap()的方法构造一个Map类,
向Map中添加元素的时,使用 对象名.put ( key,value) 的方法添加一个键值对。
打印输出有两种方式:
1)打印输出value的值(直接使用迭代器)
2)打印输输出key和value的值:通过entrySet方法
通过keySet()方法将map中所有key值的取出,返回值是个只存放key值的Set集合
通过entrySet()方法将map集合中的映射关系取出,返回映射所包含的映射关系的Set集合
虽然使用keyset及entryset来进行遍历能取得相同的结果,但两者的遍历速度是有差别的。
keySet():迭代后只能通过get()取key;再根据key值取value。
entrySet():迭代后可以e.getKey(),e.getValue()取key和value。
4、HashMap代码实例

import java.util.*; import java.util.Map.Entry; public class DictionaryDemo { public static void main(String[] args) { Map<String,String> animal=new HashMap<String,String>(); System.out.println("请输入三组单词对应的注释,并存放到HashMap中"); Scanner console=new Scanner(System.in); //添加数据 int i=0; while(i<3){ System.out.println("请输入key值(单词):"); String key=console.next(); System.out.println("请输入value值(注释):"); String value=console.next(); animal.put(key, value); i++; } //打印输出value的值(直接使用迭代器) System.out.println("*****************************************"); System.out.println("使用迭代器输出所有的value:"); Iterator<String> it=animal.values().iterator(); while(it.hasNext()){ System.out.print(it.next()+" "); } System.out.println(); System.out.println("*****************************************"); //打印输出key和value的值 //通过entrySet方法 System.out.println("通过entrySet方法得到key-value:"); Set<Entry<String, String>> entrySet=animal.entrySet(); for(Entry<String, String> entry:entrySet){ System.out.print(entry.getKey()+"-");; System.out.println(entry.getValue()); } System.out.println(); System.out.println("*****************************************"); //通过单词找到注释并输出 //使用keySet方法 System.out.println("请输入要查找的单词:"); String strSearch=console.next(); //1.取得keySet Set<String> keySet=animal.keySet(); //2.遍历keySet for(String key:keySet){ if(strSearch.equals(key)){ System.out.println("找到了!"+"键值对为:"+key+"-"+animal.get(key)); break; } } } }