函数调用
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> </head> <body> </body> <script> //函数调用 // 函数内部的this指向window var age=18; var p={ age:15, say:function(){ console.log(this.age); } } var f1=p.say; //f1是函数 f1(); //函数调用-->this:window -->this.age=18 </script> </html>
this 的含义:函数内部的this指向window
上下文调用bind:
this 的含义:执行了bind方法之后,产生了一个新函数,这个新函数里面的逻辑和原来还是一样的,唯一的不同是this指向
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>调用</title> </head> <body> <script> function speed() { console.log(this.seconds); } var speedBind=speed.bind({seconds:100}); speedBind(); </script> </body> </html>
this.seconds 为100,this指向{ seconds:100 }
上下文调用:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> </head> <body> </body> <script> //上下文调用方式,有3种,call、apply、bind function f1(){ console.log(this); } //call方法的第一个参数决定了函数内部的this的值 f1.call([1,3,5]) f1.call({age:20,height:1000}) f1.call(1) f1.call("abc") f1.call(true); f1.call(null) f1.call(undefined); //上述代码可以用apply完全替换 //总结: //call方法的第一个参数: //1、如果是一个对象类型,那么函数内部的this指向该对象 //2、如果是undefined、null,那么函数内部的this指向window //3、如果是数字-->this:对应的Number构造函数的实例 // --> 1 --> new Number(1) // 如果是字符串-->this:String构造函数的实例 // --> "abc" --> new String("abc") // 如果是布尔值-->this:Boolean构造函数的实例 // --> false --> new Boolean(false) </script> </html>
总结: //call方法的第一个参数: //1、如果是一个对象类型,那么函数内部的this指向该对象 //2、如果是undefined、null,那么函数内部的this指向window //3、如果是数字-->this:对应的Number构造函数的实例 // --> 1 --> new Number(1) // 如果是字符串-->this:String构造函数的实例 // --> "abc" --> new String("abc") // 如果是布尔值-->this:Boolean构造函数的实例 // --> false --> new Boolean(false)
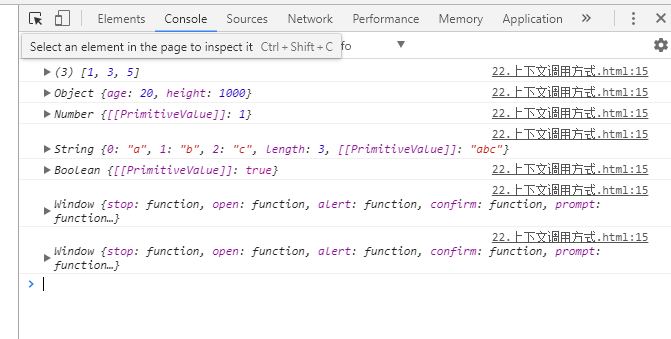
new调用(构造函数)
var age=18; var p={ age:15 say:function(){ //this:say构造函数的实例,实例中并没有age属性,值为:undefined console.log(this.age); } } new p.say()//构造函数调用 //相当于: var s1=p.say; new s1();
方法调用
var age=18;
var p={
age:15
say:function(){
console.log(this.age);//this:p
//this.age->p.age:15
}
}
p.say()//方法调用