相关概念 栈(stack)和队列(queue)都是动态集合。 在其上进行delete操作所移除的元素是预先设定的. 在stack中,被删除的是最近插入的元素: stack实现的是一种LIFO(last-in,first-out)策略。 在queue中,被删除的是在集合中存在时间最长的那个元素: queue实现的是一种FIFO(first-in,first-out)策略。 Stack上的insert操作被称为PUSH,无参数的delete操作被称为POP queue上的insert操作称为enqueue;delete操作称为dequeue Q[0...n]用来实现一个最多容纳n个元素的队列的一种方式. 该队列有一个属性Q.head指向对头元素.属性Q.tail则指向下一个新元素要插入的位置. 队列中的元素存放在位置Q.head,Q.head+1,...,Q.tail-1,并在最后的位置'环绕'. 感觉好像位子0紧邻位置n的后面,形成一个'环'. 当Q.head=Q.tail时队列为空.初始有Q.head=Q.tail=0. 如果试图从空队列中删除一个元素, 则队列发生下溢.当Q.head=Q.tail+1的时候,队列是满的. 此时若尝试插入,则队列发生上溢.
Python programming
Stack
class stack(list): # [0,0,0,0,...,depth] 通过继承 list 结构, 初始化一个深度为 depth 的空stack def __init__(self, depth): self.top = 0 def __new__(self, depth, **kwargs): # return [0 for x in range(depth)] # If __new__() is invoked during object construction and it returns an instance or subclass of cls, then the new instance’s __init__() method will be invoked like __init__(self[, ...]), where self is the new instance and the remaining arguments are the same as were passed to the object constructor. # # If __new__() does not return an instance of cls, then the new instance’s __init__() method will not be invoked. # # __new__() is intended mainly to allow subclasses of immutable types (like int, str, or tuple) to customize instance creation. It is also commonly overridden in custom metaclasses in order to customize class creation. # self.__init__(self, depth) #print("owwoow", depth) res = super(stack, self).__new__(self) while depth: res.append(0) depth -= 1 return res def stack_empty(S): # 当 S.top = 0 时, stack 中不含有任何元素, 即为 空栈. if S.top == 0: return True else: return False def push(S, x): S[S.top] = x S.top += 1 def pop(S): if stack_empty(S): print('Stack underflow') else: S.top -= 1 print('Poped', S[S.top]) S[S.top] = 0 return S[S.top] if __name__ == '__main__': print('Build a empty stack with a depth of 8') S = stack(8) print(S, S.top) #print(S.__dict__) #print(S, vars(S)) #print(vars(S)) #print(S.top) print('Push 15 in') push(S, 15) print(S, S.top) print('Push 6 in') push(S, 6) print(S, S.top) print('Push 2 in') push(S, 2) print(S, S.top) print('Push 9 in') push(S, 9) print(S, S.top) print('Push 17 in') push(S, 17) print(S, S.top) print('Push 3 in') push(S, 3) print(S, S.top) pop(S) print(S, S.top) 结果打印: Build a empty stack with a depth of 8 [0, 0, 0, 0, 0, 0, 0, 0] 0 Push 15 in [15, 0, 0, 0, 0, 0, 0, 0] 1 Push 6 in [15, 6, 0, 0, 0, 0, 0, 0] 2 Push 2 in [15, 6, 2, 0, 0, 0, 0, 0] 3 Push 9 in [15, 6, 2, 9, 0, 0, 0, 0] 4 Push 17 in [15, 6, 2, 9, 17, 0, 0, 0] 5 Push 3 in [15, 6, 2, 9, 17, 3, 0, 0] 6 Poped 3 [15, 6, 2, 9, 17, 0, 0, 0] 5
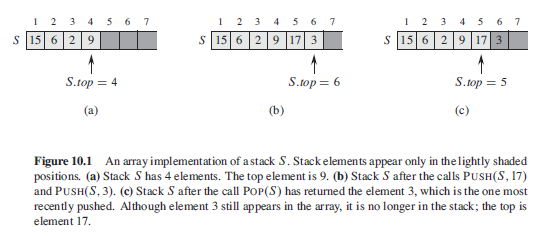
Queue class queue(list): # [0,0,0,0,...,depth] 通过继承 list 结构, 初始化一个深度为 depth 的空 Queue def __init__(self, depth): self.head = 0 self.tail = 0 self.length = depth def __new__(cls, depth, **kwargs): res = super(queue, cls).__new__(cls) while depth: res.append(0) depth -= 1 return res def enqueue(Q, x): if Q.head == Q.tail + 1: print('Queue overflow') if Q.tail == len(Q): Q.tail = 1 Q[Q.tail-1] = x if Q.tail == Q.length: Q.tail = 1 else: Q.tail += 1 def dequeue(Q): x = Q[Q.head-1] Q[Q.head-1] = 0 if Q.head == Q.length: Q.head = 0 else: Q.head += 1 print('dequeue', x) return x if __name__ == '__main__': print('BUild a empty with a depth of 12') Q = queue(12) print('Queue',Q) print('Initializing with date : 15, 6, 9, 8, 4; head: 7, tail: 12') a = [15, 6, 9, 8, 4] Q[6:11] = a Q.tail = 11 # 对齐 python list 的 index 是 0 base Q.head = 7 print('Queue', Q) print('enqueue', 17) enqueue(Q, 17) print('# tail head Q') print(Q.tail, Q.head, Q) print('enqueue', 3) enqueue(Q, 3) print('# tail head Q') print(Q.tail, Q.head, Q) print('enqueue', 5) enqueue(Q, 5) print('# tail head Q') print(Q.tail, Q.head, Q) print('# denqueue') dequeue(Q) print('# tail head Q') print(Q.tail, Q.head, Q) 结果打印: BUild a empty with a depth of 12 Queue [0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] Initializing with date : 15, 6, 9, 8, 4; head: 7, tail: 12 Queue [0, 0, 0, 0, 0, 0, 15, 6, 9, 8, 4, 0] enqueue 17 # tail head Q 12 7 [0, 0, 0, 0, 0, 0, 15, 6, 9, 8, 17, 0] enqueue 3 # tail head Q 2 7 [3, 0, 0, 0, 0, 0, 15, 6, 9, 8, 17, 0] enqueue 5 # tail head Q 3 7 [3, 5, 0, 0, 0, 0, 15, 6, 9, 8, 17, 0] # denqueue dequeue 15 # tail head Q 3 8 [3, 5, 0, 0, 0, 0, 0, 6, 9, 8, 17, 0]
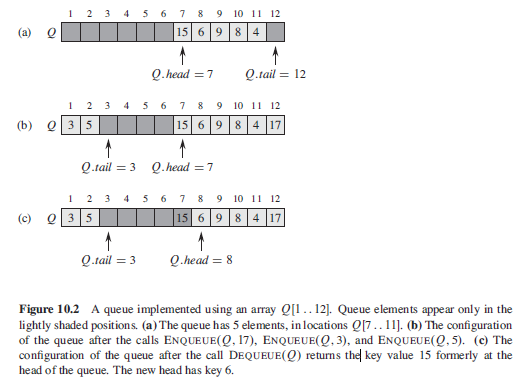
Reference
1. Introduction to algorithms