查看POST multipart/form-data协议格式
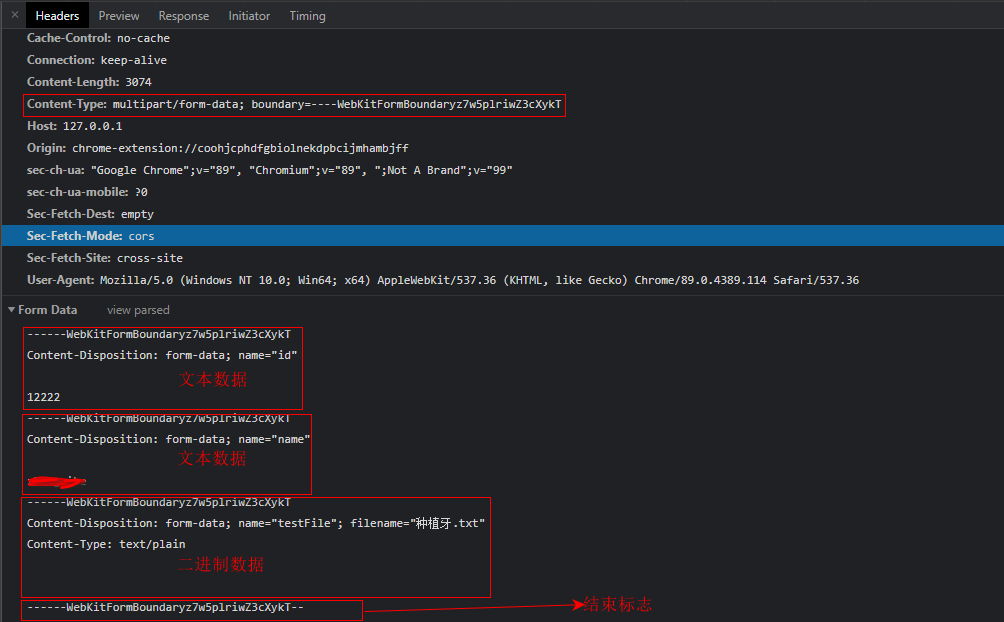
下载文件存到到字节数组并且POST multipart/form-data接口
package com.http;
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.Closeable;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class HttpDownload {
/**
*
* @param urlPath 下载路径
* @param downloadDir 下载存放目录
* @return 返回下载文件
*/
public static byte[] downloadFile(String urlPath, String downloadDir, String fileName) {
BufferedInputStream in = null;
OutputStream out = null;
HttpURLConnection connection = null;
try {
URL url = new URL(urlPath);
connection = (HttpURLConnection)url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("Content-Type", "application/octet-stream;charset=utf-8");
connection.setRequestProperty("Connection", "close");
connection.setConnectTimeout(5000);
connection.setDoOutput(true);
connection.setDoInput(true);
connection.connect();
// 文件大小
int fileLength = connection.getContentLength();
System.out.println("file length-------------" + fileLength + " (byte)");
in = new BufferedInputStream(connection.getInputStream());
String path = downloadDir + File.separatorChar + fileName;
// 下载文件到本地
File file = new File(path);
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
out = new FileOutputStream(file);
int size = 0;
int len = 0;
byte[] buf = new byte[1024 * 1024];
byte[] data = new byte[fileLength];
int dataPos = 0;
while ((size = in.read(buf)) != -1) {
len += size;
out.write(buf, 0, size);
for (int i = 0; i < size; i++) {
data[dataPos] = buf[i];
dataPos++;
}
}
System.out.println("download finish--" + fileLength + "," + len + "," + dataPos + " (byte)");
return data;
} catch (Exception e) {
e.printStackTrace();
} finally {
close(in, out);
closeConnection(connection);
}
return new byte[0];
}
public static String uploadFile(String actionUrl, Map<String, String> textMap, String filekey, byte[] data,
String fileName) {
String end = "
";
String boundary = "----WebKitFormBoundaryLBFuobxXx0AmQVFR";
DataOutputStream out = null;
BufferedReader reader = null;
HttpURLConnection connection = null;
try {
URL url = new URL(actionUrl);
connection = (HttpURLConnection)url.openConnection();
connection.setDoInput(true);
connection.setDoOutput(true);
connection.setUseCaches(false);
connection.setRequestMethod("POST");
connection.setRequestProperty("Connection", "close");
connection.setRequestProperty("Charset", "utf-8");
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + boundary);
out = new DataOutputStream(connection.getOutputStream());
// 处理文本==============================
String strData = "";
if (textMap != null) {
Iterator<Map.Entry<String, String>> iter = textMap.entrySet().iterator();
while (iter.hasNext()) {
Map.Entry<String, String> entry = iter.next();
String name = (String)entry.getKey();
String value = (String)entry.getValue();
if (value == null) {
continue;
}
value = new String(value.getBytes("utf-8"));
strData = end + "--" + boundary + end;
System.out.println(strData);
out.writeBytes(strData);
strData = "Content-Disposition: form-data; name="" + name + """ + end + end + value;
System.out.println(strData);
out.writeBytes(strData);
}
}
// 处理文本结束==============================
// 开始上传文件========================================
strData = end + "--" + boundary + end;
System.out.println(strData);
out.writeBytes(strData);
strData =
"Content-Disposition: form-data;" + " name="" + filekey + ""; filename="" + fileName + """ + end;
System.out.println(strData);
out.writeBytes(strData);
strData = "Content-Type:" + " text/plain" + end + end;
System.out.println(strData);
out.writeBytes(strData);
System.out.println("二进制...");
out.write(data);
System.out.println(end);
out.writeBytes(end);
strData = "--" + boundary + "--" + end;
System.out.println(strData);
// 开上传文件结束========================================
// 请求结束标志
out.writeBytes(strData);
out.flush();
// 获取响应
if (connection.getResponseCode() >= 300) {
throw new Exception("HTTP Request fail,response code " + connection.getResponseCode());
}
StringBuilder sb = new StringBuilder();
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) {
reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String strLine = null;
while ((strLine = reader.readLine()) != null) {
sb.append(strLine);
}
}
return sb.toString();
} catch (Exception e) {
e.printStackTrace();
} finally {
close(out, reader);
closeConnection(connection);
}
return "";
}
public static void close(Closeable... args) {
for (Closeable closeable : args) {
if (closeable != null) {
try {
closeable.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private static void closeConnection(HttpURLConnection connection) {
if (connection != null) {
connection.disconnect();
}
}
public static void main(String[] args) {
byte[] data = downloadFile(
"https://4f7fce38e855e98a0d722672fc0a4ef4.dlied1.cdntips.net/dl.softmgr.qq.com/original/Picture/FocusOnIV64_1.23.exe?mkey=607322a66f1278d3&f=9870&cip=111.18.94.38&proto=https",
"C:\Users\admin\Desktop", "FocusOnIV64_1.23.exe");
Map<String, String> textMap = new HashMap<String, String>();
textMap.put("id", "123");
textMap.put("name", "zinredible");
String responseStr =
uploadFile("http://127.0.0.1/api/his/upload", textMap, "testFile", data, "FocusOnIV64_1.23.exe");
System.out.println(responseStr);
}
}
// springboot接口
@PostMapping(value = "/api/his/upload", produces = "multipart/form-data;charset=UTF-8")
@ApiImplicitParams({@ApiImplicitParam(name = "id", paramType = "form"),
@ApiImplicitParam(name = "name", paramType = "form")})
public String uploadFile(TestParam param, MultipartFile testFile,HttpServletRequest request) throws Exception