The figure shown on the left is left-right symmetric as it is possible to fold the sheet of paper along a vertical line, drawn as a dashed line, and to cut the figure into two identical halves. The figure on the right is not left-right symmetric as it is impossible to find such a vertical line.
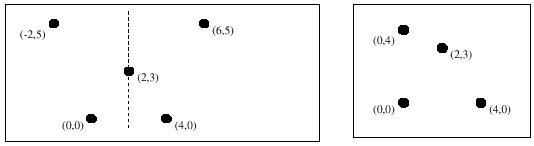
Write a program that determines whether a figure, drawn with dots, is left-right symmetric or not. The dots are all distinct.
Input
The input consists of T test cases. The number of test cases T is given in the first line of the input file. The first line of each test case contains an integer N ,
where N ( 1N
1,
000) is the number of dots in a figure. Each of the following N lines contains the x-coordinate and y-coordinate of a dot. Both x-coordinates
and y-coordinates are integers between -10,000 and 10,000, both inclusive.
Output
Print exactly one line for each test case. The line should contain `YES' if the figure is left-right symmetric. and `NO', otherwise.
The following shows sample input and output for three test cases.
Sample Input
3 5 -2 5 0 0 6 5 4 0 2 3 4 2 3 0 4 4 0 0 0 4 5 14 6 10 5 10 6 14
Sample Output
YES NO YES
#include <iostream> #include <algorithm> #include <vector> using namespace std; struct point { int x; int y; bool operator < (const point &b) const { int x1 = this->x; int y1 = this->y; int x2 = b.x; int y2 = b.y; if(y1 != y2) return y1 < y2; else return x1 < x2; } bool operator == (const point &b) const { if(x == b.x && y==b.y) return true; else return false; } }; int main() { int T; cin >> T; while(T--) { int N; cin >> N; vector<point> box; for(int i = 0; i<N; i++) { point temp; cin >> temp.x >> temp.y; box.push_back(temp); } sort(box.begin(), box.end()); double center; bool flag; for(auto i = box.begin(); i!=box.end() && (*i).y==box[0].y; i++) { center = ( (*i).x + box[0].x ) / 2.0; flag = true; for(auto i = box.begin(); i!=box.end(); i++) { point M; M.x = (2.0 * center) - (*i).x; M.y = (*i).y; if(find(box.begin(), box.end(), M)==box.end()) { flag = false; break; } } if(flag==true) { cout << "YES" << endl; break; } } if(flag == false) cout << "NO" << endl; } return 0; }