div切换
<template>
<div id="app">
<div class="tab-tit">
<!--点击设置curId的值 如果curId等于0,第一个a添加cur类名,如果curId等于1,第二个a添加cur类名,以此类推。添加了cur类名,a就会改变样式 @click,:class ,v-show这三个是vue常用的指令或添加事件的方式-->
<a href="javascript:;" @click="curId=0" :class="{'cur':curId===0}">html</a>
<a href="javascript:;" @click="curId=1" :class="{'cur':curId===1}">css</a>
<a href="javascript:;" @click="curId=2" :class="{'cur':curId===2}">javascript</a>
<a href="javascript:;" @click="curId=3" :class="{'cur':curId===3}">vue</a>
</div>
<div class="tab-con">
<!--根据curId的值显示div,如果curId等于0,第一个div显示,其它三个div不显示。如果curId等于1,第二个div显示,其它三个div不显示。以此类推-->
<div v-show="curId===0">
html<br/>
</div>
<div v-show="curId===1">
css
</div>
<div v-show="curId===2">
javascript
</div>
<div v-show="curId===3">
vue
</div>
</div>
</div>
</template>
<script>
export default {
data(){
return{
curId:1,
}
},
}
</script>
<style scoped>
body{
font-family:"Microsoft YaHei";
}
#app{
600px;
margin: 0 auto;
}
.tab-tit{
font-size: 0;
600px;
}
.tab-tit a{
display: inline-block;
height: 40px;
line-height: 40px;
font-size: 16px;
25%;
text-align: center;
background: #ccc;
color: #333;
text-decoration: none;
}
.tab-tit .cur{
background: #09f;
color: #fff;
}
.tab-con div{
border: 1px solid #ccc;
height: 400px;
padding-top: 20px;
}
</style>
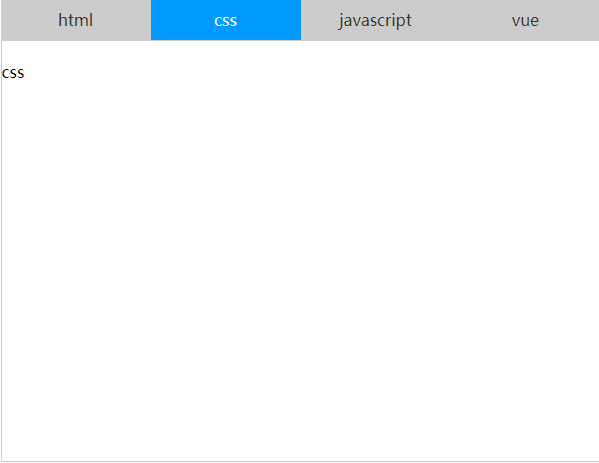
购物车
<template>
<div id="app">
<div v-if="books.length==0">
购物车为空
</div>
<div v-else>
<table>
<thead>
<tr>
<th></th>
<th>书籍</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for='(value,index) in books'>
<td >{{value.id}}</td>
<td >{{value.name}}</td>
<td >{{value.date}}</td>
<td >{{value.price | showPrice}}</td> <!--使用过滤器改变金额格式-->
<td >
<button @click="decrement(index)" v-bind:disabled="value.count <=1">-</button> <!--按钮失效-->
{{value.count}}
<button @click="increment(index)">+</button>
</td>
<td >
<button @click='remove(index)'>移除</button>
</td>
</tr>
</tbody>
</table>
<h2 >总价格为{{totalPrice | showPrice}}</h2>
</div>
</div>
</template>
<script>
export default {
data(){
return{
books:[
{
id:1,
name:'小黄书',
date:'2020-09',
price:80.00,
count:2
},
{
id:2,
name:'博人传',
date:'2020-09',
price:51.00,
count:2
},
{
id:3,
name:'王书',
date:'2020-09',
price:80.00,
count:2
},
{
id:4,
name:'牛逼书',
date:'2020-09',
price:80.00,
count:2
}
]
}
},
computed:{
totalPrice(){
let total = 0;
for(let i = 0; i < this.books.length; i++){
total += this.books[i].price * this.books[i].count;
}
return total;
}
},
methods:{
increment(index){
console.log('hello'+index);
this.books[index].count++;
},
decrement(index){
if(this.books[index].count>=2){
console.log('world'+index);
this.books[index].count--;
}
},
remove(index){
this.books.splice(index,1);/*移除*/
}
},
filters:{
showPrice(price){
return '¥'+price.toFixed(2);/*保留两位小数*/
}
}
}
</script>
<style scoped>
table{
border:1px solid #e9e9e9;
border-collapse: collapse;
border-spacing: 0;
}
th,td{
padding:8px 16px;
border:1px solid #E9E9E9;
text-align: left;
}
th{
background-color: #f7f7f7;
color:#5c6b77;
font-weight: 600;
}
</style>
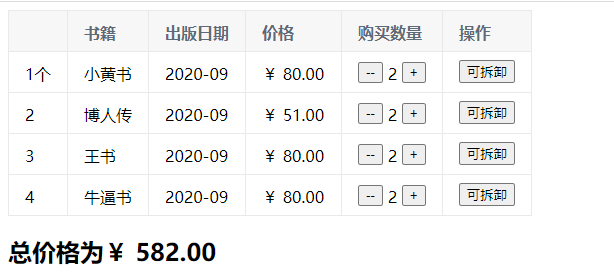