BENEFACT - The Benefactor
Another chapter of the municipal chronicles of a well known unbelievable lordly major town (if this town is not well known to you, you might want to solve problem CSTREET first) tells us the following story:
Once upon a time the citizens of the unbelievable lordly major town decided to elect a major. At that time this was a very new idea and election campaigns were completely unknown. But of course several citizens wanted to become major and it didn't took long for them to find out, that promising nice things that never will become real tends to be useful in such a situation. One candidate to be elected as a major was Ivo sometimes called the benefactor because of his valuable gifts to the unbelievably lordly major towns citizens.
One day before the election day Ivo the benefactor made a promise to the citizens of the town. In case of his victory in the elections he would ensure that on one of the paved streets of the town street lamps would be erected and that he would pay that with his own money. As thrifty as the citizens of the unbelievable lordly major town were, they elected him and one day after the elections they presented him their decision which street should have street lamps. Of course they chose not only the longest of all streets but renamed several streets so that a very long street in the town existed.
Can you find how long this street was? To be more specific, the situation is as follows. You are presented a list of all paved streets in the unbelievable lordly major town. As you might remember from problem CSTREET in the town the streets are paved in a way that between every two points of interest in the town exactly one paved connection exists. Your task is to find the longest distance that exists between any two places of interest in the city.
Input
The first line of input contains the number of testcases t.
The first line of each testcase contains the number of places (2 <= n <= 50000) in the town. Each street is given at one line by two places (1 <= a, b <= n) and the length of the street (0 <= l < 20000).
Output
For each testcase output one line which contains the maximum length of the longest street in the city.
Example
Input: 1 6 1 2 3 2 3 4 2 6 2 6 4 6 6 5 5 Output: 12
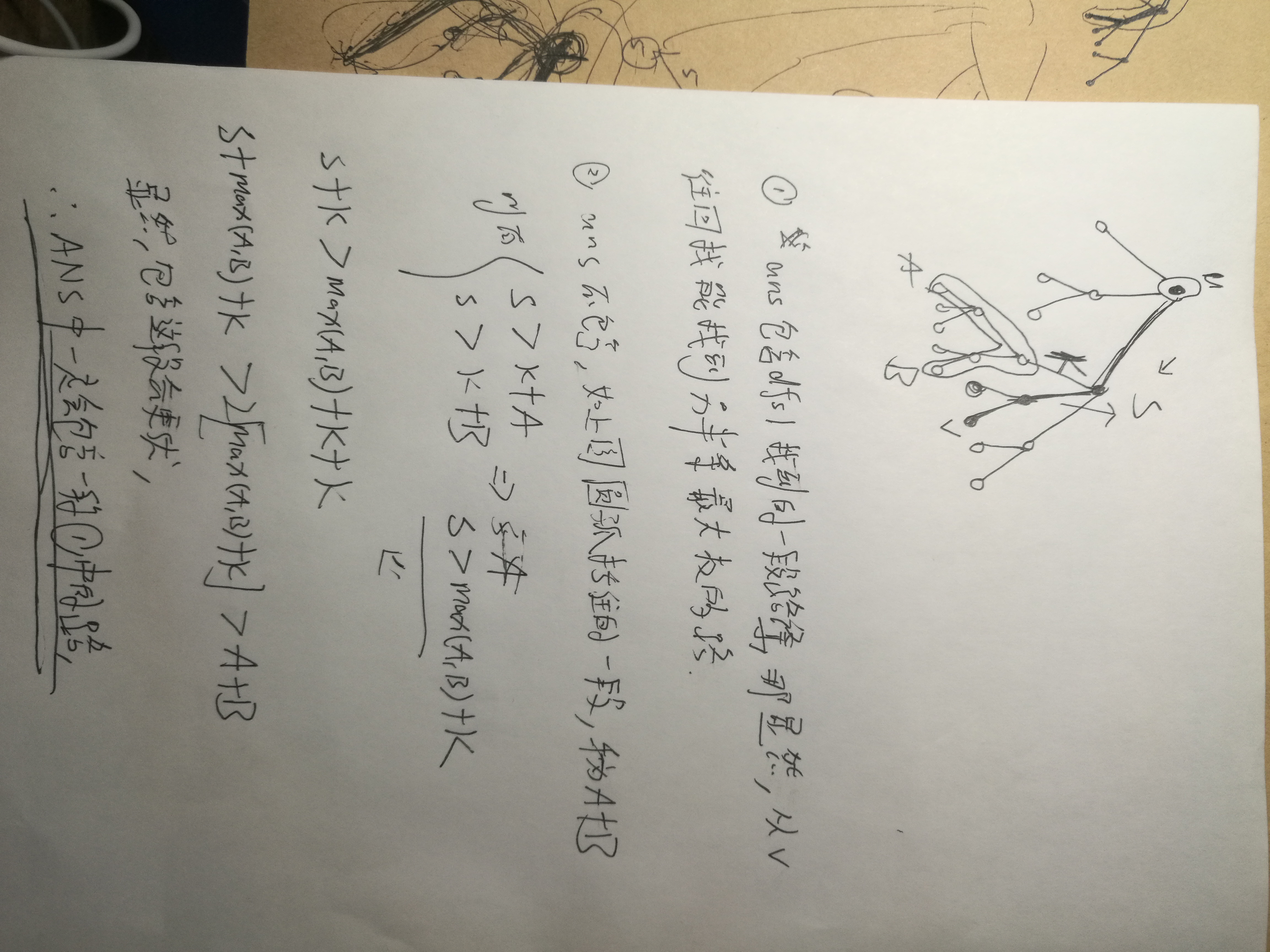
1 #include<bits/stdc++.h> 2 using namespace std; 3 #define LL long long 4 #define pii pair<int,int> 5 vector<pair<int,int> >g[50005]; 6 bool vis[50005]; 7 pii dfs(int n){ 8 vis[n]=1; 9 pii r=make_pair(n,0); 10 for(int i=0;i<g[n].size();++i){ 11 pii p=g[n][i]; 12 if(vis[p.first]) continue; 13 pii t=dfs(p.first); 14 if(p.second+t.second>r.second){ 15 r=t; 16 r.second+=p.second; 17 } 18 } 19 return r; 20 } 21 int main() 22 { 23 int i,j,t,n; 24 int u,v,w; 25 cin>>t; 26 while(t--){ 27 scanf("%d",&n); 28 for(i=1;i<=n;++i) g[i].clear(); 29 for(i=1;i<n;++i){ 30 scanf("%d%d%d",&u,&v,&w); 31 g[u].push_back(make_pair(v,w)); 32 g[v].push_back(make_pair(u,w)); 33 } 34 int ans=-1; 35 memset(vis,0,sizeof(vis)); 36 pii t1=dfs(1); 37 memset(vis,0,sizeof(vis)); 38 cout<<dfs(t1.first).second<<endl; 39 } 40 return 0; 41 }
GCPC11J - Time to live
As you might know, most computer networks are organized in a tree-like fashion, i.e. each computer is reachable by each other computer but only over one, unique path.
The so-called Time to live (TTL) specifies after how many hops a network packet is dropped if it has not reached its destination yet. The purpose of the TTL is to avoid situations in which a packet circulates through the network caused by errors in the routing tables.
The placement of a router that connects the network to another network is optimal when the maximal needed TTL for packets that are sent from this router to any other computer within the same network is minimal. Given a network as specified above, you should calculate the maximal needed TTL in this network if you can select the computer that should be used as router.
Input
The first line of the input consists of the number of test cases c that follow (1 ≤ c ≤ 100). Each test case starts with a line specifying N, the number of computers in this network (1 < N ≤ 105). Computers are numbered from 0 to N-1. Then follow N-1 lines, each specifying a network connection by two numbers a and b which means that computer a is connected to computer b and vice versa, of course (0 ≤ a,b < N).
Output
For each test case in the input, print one line containing the optimal TTL as specified above.
Example
Input: 3 2 1 0 5 3 2 2 1 0 2 2 4 9 3 1 6 5 3 4 0 3 8 1 1 7 1 6 2 3 Output: 1 1 2
这道题和上一题类似,相当于每条边的权值都是1了而已,算出最大权值后/2就是答案了。
1 #include<bits/stdc++.h> 2 using namespace std; 3 #define LL long long 4 #define pii pair<int,int> 5 vector<pair<int,int> >g[100005]; 6 bool vis[100005]; 7 pii dfs(int n){ 8 vis[n]=1; 9 pii r=make_pair(n,0); 10 for(int i=0;i<g[n].size();++i){ 11 pii p=g[n][i]; 12 if(vis[p.first]) continue; 13 pii t=dfs(p.first); 14 if(p.second+t.second>r.second){ 15 r=t; 16 r.second+=p.second; 17 } 18 } 19 return r; 20 } 21 int main() 22 { 23 int i,j,t,n; 24 int u,v,w; 25 cin>>t; 26 while(t--){ 27 scanf("%d",&n); 28 for(i=0;i<=n;++i) g[i].clear(); 29 for(i=1;i<n;++i){ 30 scanf("%d%d",&u,&v); 31 g[u].push_back(make_pair(v,1)); 32 g[v].push_back(make_pair(u,1)); 33 } 34 memset(vis,0,sizeof(vis)); 35 pii t1=dfs(1); 36 memset(vis,0,sizeof(vis)); 37 int ans=dfs(t1.first).second; 38 if(ans%2==1) ans++; 39 cout<<ans/2<<endl; 40 } 41 return 0; 42 }