本文讲解如何在spring-boot中使用swagger文档自动生成工具
目录结构
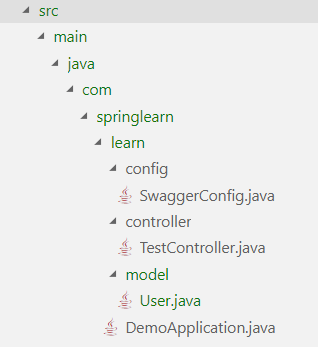
说明
swagger的使用本身是非常简单的,因为所有的一切swagger都帮你做好了,你所要做的就是专注写你的api信息
swagger自动生成html文档并且打包到jar包中
本文不仅用了swagger,JSR 303注释信息也支持使用
依赖
// springfox-bean-validators 用来支持 JSR 303
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-bean-validators</artifactId>
<version>2.9.2</version>
</dependency>
SwaggerConfig
package com.springlearn.learn.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport;
import springfox.bean.validators.configuration.BeanValidatorPluginsConfiguration;
import springfox.documentation.builders.ApiInfoBuilder;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.Contact;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
import static springfox.documentation.builders.PathSelectors.regex;
@Configuration
@EnableSwagger2
@Import(BeanValidatorPluginsConfiguration.class)
public class SwaggerConfig extends WebMvcConfigurationSupport {
private ApiInfo metaData() {
return new ApiInfoBuilder()
.title("Spring Boot REST API")
.description(""REST API 测试"")
.version("1.0.0")
.license("无")
.licenseUrl("https://www.baidu.com")
.contact(new Contact("yejiawei", "https://www.cnblogs.com/ye-hcj/", "2640199637@qq.com"))
.build();
}
@Bean
public Docket productApi() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.springlearn.learn.controller"))
.paths(regex("/.*"))
.build()
.apiInfo(metaData());
}
@Override
protected void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("swagger-ui.html")
.addResourceLocations("classpath:/META-INF/resources/");
registry.addResourceHandler("/webjars/**")
.addResourceLocations("classpath:/META-INF/resources/webjars/");
}
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("*").allowedMethods("GET", "POST", "PUT", "DELETE").allowedOrigins("*")
.allowedHeaders("*");
}
}
开启api界面
在你做好了上述的步骤以后,运行项目
直接访问 yourapiurl/v2/api-docs 查看json数据
直接访问 yourapiurl/swagger-ui.html 查看文档界面
JSR 303注释信息
@NotNull(message = "...")
@NotBlank(message = "...")
@Pattern(regexp = "[a-z-A-Z]*", message = "...")
@Past(message = "过去的日期...")
@Min(value = 1, message = "必须大于或等于1")
@Max(value = 10, message = "必须小于或等于10")
@Email(message = "Email Address")
Swagger核心注释
@ApiModel 返回json对象描述
@ApiModelProperty 返回json对象属性描述
@ApiResponses 设置状态码含义
@Api 控制器描述
@ApiOperation api描述
@ApiParam api参数描述
User
package com.springlearn.learn.model;
import javax.validation.constraints.Max;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotBlank;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
@ApiModel(description = "用户基本信息")
public class User {
// @NotBlank
// @Size(min = 1, max = 10)
@ApiModelProperty(notes = "用户姓名", example = "叶家伟", required = true, position = 0)
String name;
// @NotBlank
@Pattern(regexp ="[m|f]")
@ApiModelProperty(notes = "用户性别", example = "m", required = true, position = 1)
Character sex;
// @NotNull
// @Min(0)
// @Max(100)
@ApiModelProperty(notes = "用户年龄", example = "19", position = 3)
Integer age;
public String getname() {
return name;
}
public void setname(String name) {
this.name = name;
}
public Character getsex() {
return sex;
}
public void setsex(Character sex) {
this.sex = sex;
}
public Integer getage() {
return age;
}
public void setage(Integer age) {
this.age = age;
}
}
TestController
package com.springlearn.learn.controller;
import javax.servlet.http.HttpServletRequest;
import com.springlearn.learn.model.User;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import io.swagger.annotations.ApiResponse;
import io.swagger.annotations.ApiResponses;
@RestController
@Api(value="测试控制器", description="测试控制器描述")
@ApiResponses(value = {
@ApiResponse(code = 200, message = "Successfully retrieved list"),
@ApiResponse(code = 401, message = "You are not authorized to view the resource"),
@ApiResponse(code = 403, message = "Accessing the resource you were trying to reach is forbidden"),
@ApiResponse(code = 404, message = "The resource you were trying to reach is not found")
})
public class TestController {
@ResponseBody
@RequestMapping(value = "/test1/{id}", method = RequestMethod.GET, produces = "application/json")
@ApiOperation(value = "测试api", response = User.class)
public User Test1(HttpServletRequest request, @ApiParam("Id of the person to be obtained. Cannot be empty.") @PathVariable int id){
User user = new User();
user.setname("叶家伟");
user.setage(18);
user.setsex('m');
return user;
}
}