/**
图片的两种加载方式:
1> imageNamed:
a. 就算指向它的指针被销毁,该资源也不会被从内存中干掉,
b. 放到Assets.xcassets的图片,默认就有缓存,
c. 图片经常被使用,用这种方式加载,
2> imageWithContentsOfFile:
a. 指向它的指针被销毁,该资源会被从内存中干掉,
b. 放到项目中的图片就不带缓存,
c. 不经常用,大批量的图片,使用这种方式,
*/
/**
* 游戏结束
*/
- (IBAction)gameOver { //没有强指针,就把图片释放了,即使是图片数组,只要把图片数组的首地址置位nil,数组就释放了,所以只要首地址置位nil就可以
self.standImages = nil;
self.smallImages = nil;
self.bigImages = nil;
self.imageView.animationImages = nil;
}
//
// ViewController.m
// 07-拳皇动画(加载图片)
//
#import "ViewController.h"
@interface ViewController ()
@property (weak, nonatomic) IBOutlet UIImageView *imageView;
/** 站立 */
@property (nonatomic, strong) NSArray *standImages; //不是故事板上的东西,没有强引用指向,所以这里用strong,
/** 小招 */
@property (nonatomic, strong) NSArray *smallImages;
/** 大招 */
@property (nonatomic, strong) NSArray *bigImages;
@end
@implementation ViewController
// 初始化一些数据
- (void)viewDidLoad {
[super viewDidLoad];
// 1.加载所有的站立图片
self.standImages = [self loadAllImagesWithimagePrefix:@"stand" count:10];
// 2.加载所有的小招图片
self.smallImages = [self loadAllImagesWithimagePrefix:@"xiaozhao3" count:39];
// 3.加载所有的大招图片
self.bigImages = [self loadAllImagesWithimagePrefix:@"dazhao" count:87];
// 4.站立
[self stand];
}
/**
* 加载所有的图片
*
* @param imagePrefix 名称前缀
* @param count 图片的总个数
*/
- (NSArray *)loadAllImagesWithimagePrefix:(NSString *)imagePrefix count:(int)count{
NSMutableArray<UIImage *> *images = [NSMutableArray array];
for (int i=0; i<count; i++) {
// 获取所有图片的名称
NSString *imageName = [NSString stringWithFormat:@"%@_%d",imagePrefix, i+1];
// 创建UIImage
UIImage *image = [UIImage imageNamed:imageName];
// 装入数组
[images addObject:image];
}
return images;
}
/**
* 站立
*/
- (IBAction)stand {
// 2.设置动画图片
self.imageView.animationImages = self.standImages;
// 3.设置播放次数
self.imageView.animationRepeatCount = 0;
// 4.设置播放的时长
self.imageView.animationDuration = 0.6;
// 5.播放
[self.imageView startAnimating];
}
/**
* 小招
*/
- (IBAction)smallZhao {
// 2.设置动画图片
self.imageView.animationImages = self.smallImages;
// 3.设置动画次数
self.imageView.animationRepeatCount = 1;
// 4.设置播放时长
self.imageView.animationDuration = 1.5;
// 5.播放
[self.imageView startAnimating];
// 6.站立(延迟),执行一个方法,传入参数,延迟多长时间,
// Selector 方法
// Object 参数
// afterDelay 时间
// NSSelectorFromString(NSString * _Nonnull aSelectorName) 效果一样
[self performSelector:@selector(stand) withObject:nil afterDelay:self.imageView.animationDuration];
}
/**
* 大招
*/
- (IBAction)bigZhao {
// 2.设置动画图片
self.imageView.animationImages = self.bigImages;
// 3.设置动画次数
self.imageView.animationRepeatCount = 1;
// 4.设置播放时长
self.imageView.animationDuration = 2.5;
// 5.播放
[self.imageView startAnimating];
// 6.站立
[self performSelector:@selector(stand) withObject:nil afterDelay:self.imageView.animationDuration];
}
@end
//
// ViewController.m
// 07-拳皇动画(加载图片)
//
#import "ViewController.h"
@interface ViewController ()
@property (weak, nonatomic) IBOutlet UIImageView *imageView;
/** 站立 */
@property (nonatomic, strong) NSArray *standImages;//不是故事板上的东西,没有强引用指向,所以这里用strong,
/** 小招 */
@property (nonatomic, strong) NSArray *smallImages;
/** 大招 */
@property (nonatomic, strong) NSArray *bigImages;
@end
@implementation ViewController
/**
图片的两种加载方式:
1> imageNamed:
a. 就算指向它的指针被销毁,该资源也不会被从内存中干掉,
b. 放到Assets.xcassets的图片,默认就有缓存,
c. 图片经常被使用,用这种方式加载,
2> imageWithContentsOfFile:
a. 指向它的指针被销毁,该资源会被从内存中干掉,
b. 放到项目中的图片就不带缓存,
c. 不经常用,大批量的图片,使用这种方式,
*/
// 初始化一些数据
- (void)viewDidLoad {
[super viewDidLoad];
// 1.加载所有的站立图片
self.standImages = [self loadAllImagesWithimagePrefix:@"stand" count:10];
// 2.加载所有的小招图片
self.smallImages = [self loadAllImagesWithimagePrefix:@"xiaozhao3" count:39]; //不需要写外层文件夹的名字,只需要写图片文件的名字即可。
// 3.加载所有的大招图片
self.bigImages = [self loadAllImagesWithimagePrefix:@"dazhao" count:87];
// 4.站立
[self stand];
}
/**
* 加载所有的图片
*
* @param imagePrefix 名称前缀
* @param count 图片的总个数
*/
- (NSArray *)loadAllImagesWithimagePrefix:(NSString *)imagePrefix count:(int)count{
NSMutableArray<UIImage *> *images = [NSMutableArray array];
for (int i=0; i<count; i++) {
// 获取所有图片的名称
NSString *imageName = [NSString stringWithFormat:@"%@_%d",imagePrefix, i+1];
// 创建UIImage
// UIImage *image = [UIImage imageNamed:imageName];
NSString *imagePath = [[NSBundle mainBundle] pathForResource:imageName ofType:@"png"];
UIImage *image = [UIImage imageWithContentsOfFile:imagePath];
// 装入数组
[images addObject:image];
}
return images;
}
/**
* 站立
*/
- (IBAction)stand {
/*
// 2.设置动画图片
self.imageView.animationImages = self.standImages;
// 3.设置播放次数
self.imageView.animationRepeatCount = 0;
// 4.设置播放的时长
self.imageView.animationDuration = 0.6;
// 5.播放
[self.imageView startAnimating];
*/
[self palyZhaoWithImages:self.standImages count:0 duration:0.6 isStand:YES];
}
/**
* 小招
*/
- (IBAction)smallZhao {
/*
// 2.设置动画图片
self.imageView.animationImages = self.smallImages;
// 3.设置动画次数
self.imageView.animationRepeatCount = 1;
// 4.设置播放时长
self.imageView.animationDuration = 1.5;
// 5.播放
[self.imageView startAnimating];
// 6.站立(延迟)
[self performSelector:@selector(stand) withObject:nil afterDelay:self.imageView.animationDuration];
*/
[self palyZhaoWithImages:self.smallImages count:1 duration:1.5 isStand:NO];
}
/**
* 大招
*/
- (IBAction)bigZhao {
/*
// 2.设置动画图片
self.imageView.animationImages = self.bigImages;
// 3.设置动画次数
self.imageView.animationRepeatCount = 1;
// 4.设置播放时长
self.imageView.animationDuration = 2.5;
// 5.播放
[self.imageView startAnimating];
// 6.站立
[self performSelector:@selector(stand) withObject:nil afterDelay:self.imageView.animationDuration];
*/
[self palyZhaoWithImages:self.bigImages count:1 duration:2.5 isStand:NO];
}
/**
* 游戏结束
*/
- (IBAction)gameOver { //没有强指针,就把图片释放了,即使是图片数组,只要把图片数组的首地址置位nil,数组就释放了,所以只要首地址置位nil就可以
self.standImages = nil;
self.smallImages = nil;
self.bigImages = nil;
self.imageView.animationImages = nil;
}
/**
* 放招
*
* @param images 图片数组
* @param count 播放次数
* @param duration 播放时间
* @param isStand 是否站立
*/
- (void)palyZhaoWithImages:(NSArray *)images count: (NSInteger)count duration:(NSTimeInterval)duration isStand:(BOOL)isStand{
// 1.设置动画图片
self.imageView.animationImages = images;
// 2.设置动画次数
self.imageView.animationRepeatCount = count;
// 3.设置播放时长
self.imageView.animationDuration = duration;
// 4.播放
[self.imageView startAnimating];
// 5.站立
if (!isStand) {
[self performSelector:@selector(stand) withObject:nil afterDelay:self.imageView.animationDuration];
}
}
@end
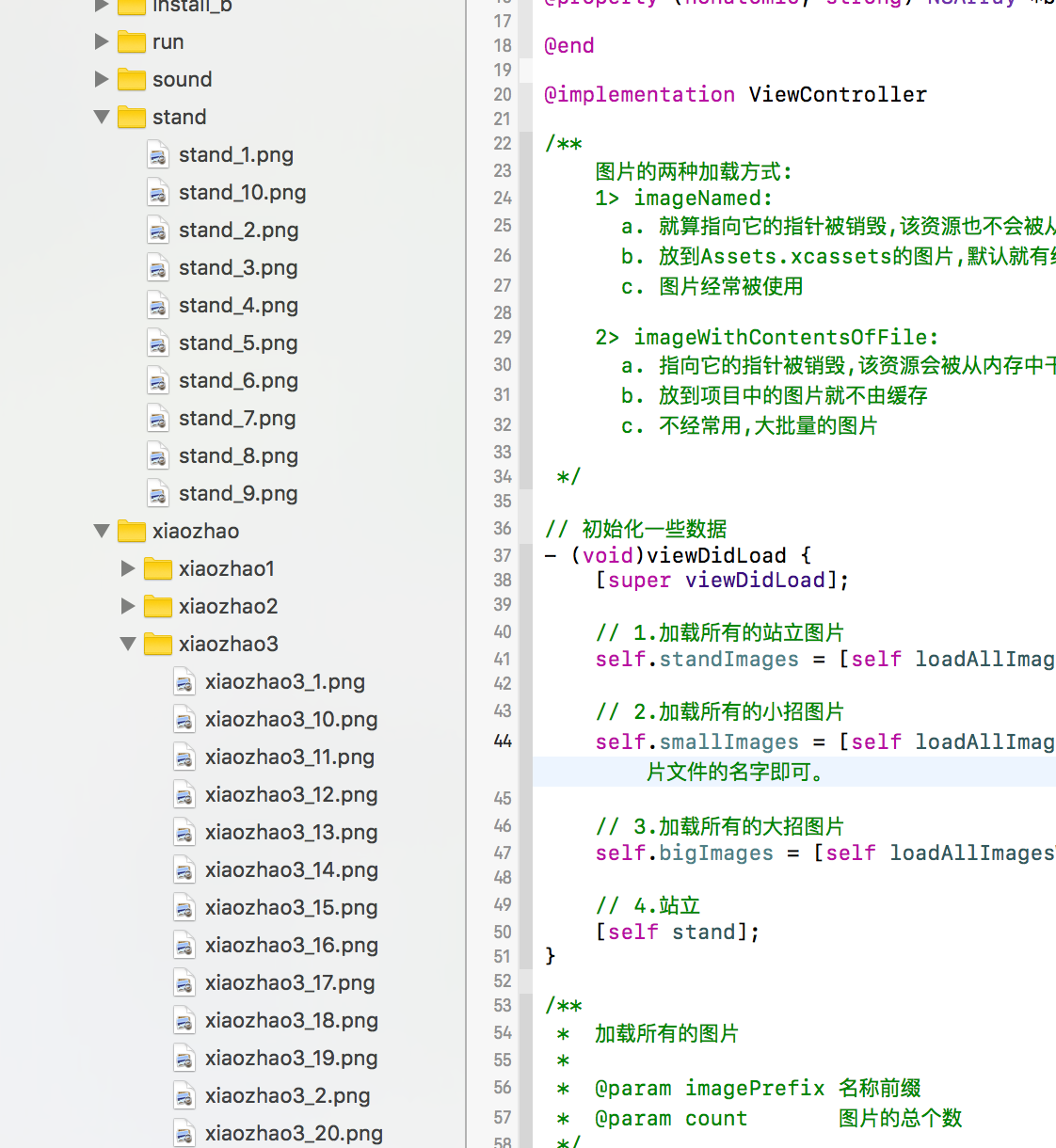
/**
图片的两种加载方式:
1> imageNamed:
a. 就算指向它的指针被销毁,该资源也不会被从内存中干掉,
b. 放到Assets.xcassets的图片,默认就有缓存,
c. 图片经常被使用,用这种方式加载,
2> imageWithContentsOfFile:
a. 指向它的指针被销毁,该资源会被从内存中干掉,
b. 放到项目中的图片就不带缓存,
c. 不经常用,大批量的图片,使用这种方式,
*/
/**
* 游戏结束
*/
- (IBAction)gameOver { //没有强指针,就把图片释放了,即使是图片数组,只要把图片数组的首地址置位nil,数组就释放了,所以只要首地址置位nil就可以
self.standImages = nil;
self.smallImages = nil;
self.bigImages = nil;
self.imageView.animationImages = nil;
}