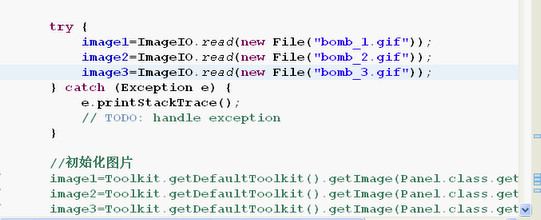
image1=ImageIO.read(new File("bomb_1.gif"));
图片拷贝:
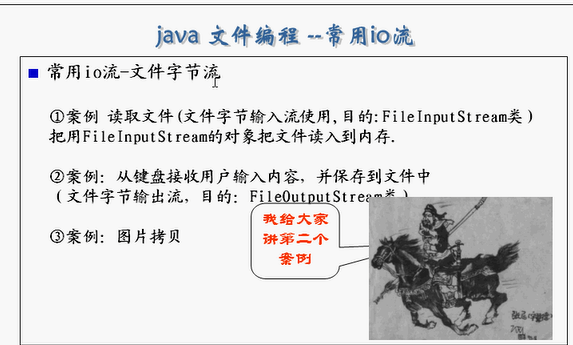
输入流和输出流同时要用的问题:
图片读入到内存;
写入,输出到某个文件;
二进制文件,字节流完成;
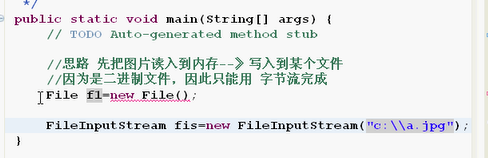
FileInputStream fis=new FileInputStream("xxxx.jpg");
输出到指定文件:FileOutputStream fos=null;
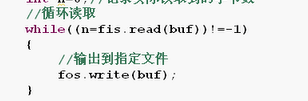
关闭打开的文件流:
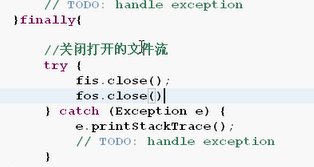
------------------------------------------
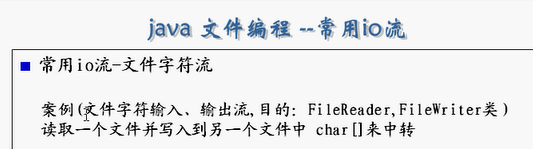
FileReader,FileWriter类,文件字符流的对象;
Reader读进来,Writer写出去;
byte 是一位,不足以表示汉字;
char 是两位,可以表示一个汉字;
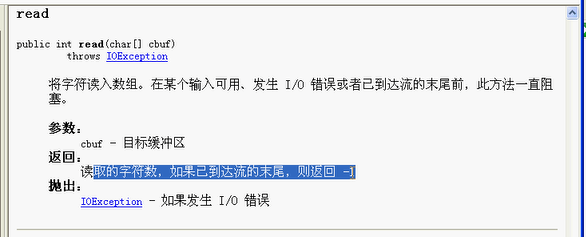
达到文件的末尾,返回-1;
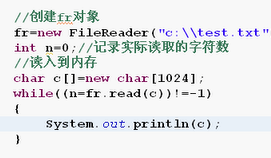
char是1024个字符;
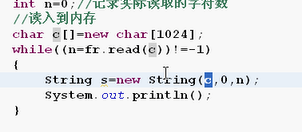
String s=new String(c,0,n);
这样,就没有乱码;
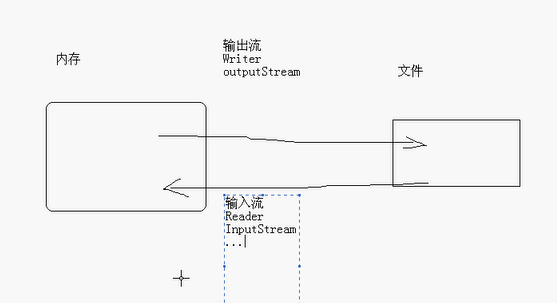
关闭文件:finally---fr.close---fw.close
fw.write(c,0,n); 读取文件,防止乱码;
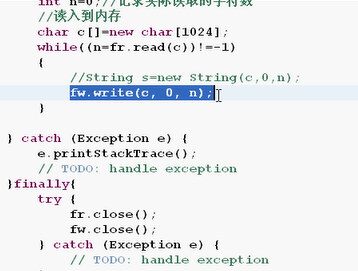
因为,发现JAVA开发者效率还是不够高,
提出缓冲字符流:提高效率;
一个一个字节/字符读取,速度太慢;
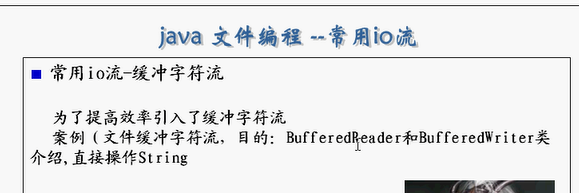
是一种编译异常,
BufferedReader缓存为对象;
先通过FileReader找到一个文件,
然后通过BufferReader进行缓存;
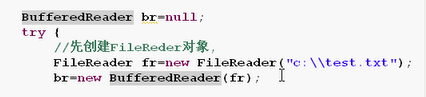
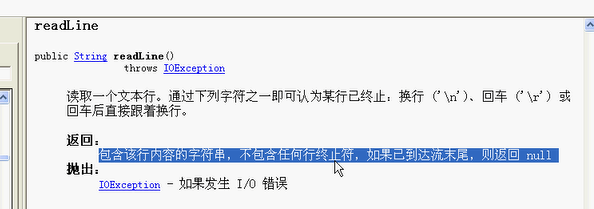
readLine不读取换行的;末尾,返回null;
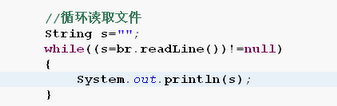
一行一行的读取,速度比较快;
BufferedWriter输出到磁盘;
FileWriter找到对象;
BufferedWriter输出到缓存;
文件的关闭:文件不关闭,后果很严重:
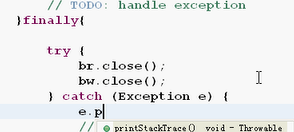
换行:
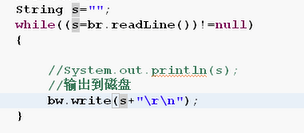
坦克存盘退出:
记录坦克的坐标
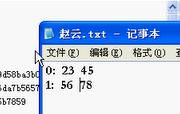
一行行读进来,让坦克暂停;
记事本功能的实现:
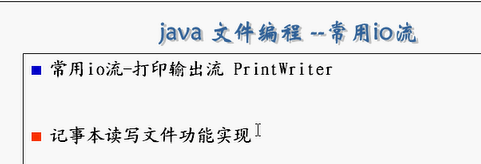
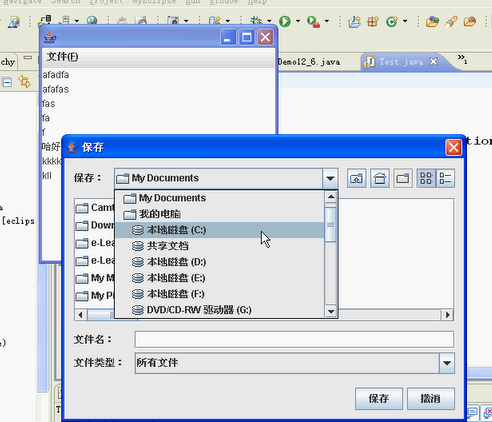
---------------------------------------------
x
39
1
java文件编程--常用io流
2
常用io流--文件字节流
3
1、案例[Io02.java]:读取文件(文件字节输入流使用,目的:FileInputStream类)把用FileInputStream的对象把文件读入到内存
4
/**
5
* File类的基本用法
6
* io流--文件字节流
7
* FileInputStream类的使用
8
*/
9
import java.io.*;
10
public class Io02 {
11
public static void main(String[] args) {
12
//得到一个文件对象,f指向e:ffhsp.txt文件
13
File f=new File("e:\ff\hsp.txt");
14
FileInputStream fis=null;
15
try {
16
//因为File没有读写的能力,所以需要使用InputStream类
17
fis=new FileInputStream(f);
18
//定义一个字节数组,相当于缓存
19
byte []bytes=new byte[1024];
20
int n=0;//得到实际读取到的字节数
21
//循环读取
22
while((n=fis.read(bytes))!=-1){
23
//把字节转成String
24
String s=new String(bytes,0,n);
25
System.out.println(s);
26
}
27
} catch (Exception e) {
28
e.printStackTrace();
29
}finally{
30
//关闭文件流必需放在finally语句块中
31
try {
32
fis.close();
33
} catch (Exception e) {
34
e.printStackTrace();
35
}
36
}
37
}
38
}
39
2、案例[Io03.java]:从键盘接收用户输入内容,并保存到文件中(文件字节输出流,目的:FileOutputStream类)
1
33
1
/**
2
* File类的基本用法
3
* io流--文件字节流
4
* FileOutputStream类的使用
5
*/
6
import java.io.*;
7
public class Io03 {
8
public static void main(String[] args) {
9
File f=new File("e:\ff\ss.txt");//直接覆盖写同一个文件
10
//字节输出流
11
FileOutputStream fos=null;
12
if(f.exists()){
13
System.out.println("文件已存在");
14
}else{
15
try {
16
fos=new FileOutputStream(f);
17
String s="hello,world!
";
18
String s1="中国人";
19
fos.write(s.getBytes());
20
fos.write(s1.getBytes());
21
} catch (Exception e) {
22
e.printStackTrace();
23
}finally{
24
try {
25
fos.close();
26
} catch (Exception e2) {
27
e2.printStackTrace();
28
}
29
}
30
}
31
}
32
}
33
3、案例[Io04.java]:图片拷贝
1
40
1
/**
2
* File类的基本用法
3
* io流--文件字节流
4
* 图片拷贝--FileInputStream类与 FileOutputStream类
5
*/
6
import java.io.*;
7
public class Io04 {
8
public static void main(String[] args) {
9
//先将图片读入到内存,再将内存中的图片写入到某个文件
10
//因为二进制文件只能拿使用字节流来处理
11
//输入流
12
FileInputStream fis=null;
13
//输出流
14
FileOutputStream fos=null;
15
16
try {
17
fis=new FileInputStream("e:\ff\a.jpg");
18
fos=new FileOutputStream("e:\a.jpg");
19
byte buf[]=new byte[1024];
20
int n=0;//记录实际读取到的字节数
21
//循环读取图片
22
while((n=fis.read(buf))!=-1){
23
//输出到指定文件
24
fos.write(buf);
25
}
26
27
} catch (Exception e) {
28
e.printStackTrace();
29
}finally{
30
//一定要关闭打开的文件流
31
try {
32
fis.close();
33
fos.close();
34
} catch (Exception e) {
35
e.printStackTrace();
36
}
37
}
38
}
39
}
40