继承映射
对象模型(Java类结构)

一个类继承体系一张表(subclass)(表结构)
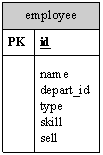
1、一个类继承体系一张表(subclass)(映射文件)
<class name="Employee" table="employee" discriminator-value="0"> <id name="id"> <generator class="native"/> </id> <discriminator column="type" type="int"/> <property name="name"/> <many-to-one name=”depart” column=”depart_id”/> <subclass name="Skiller" discriminator-value="1"> <property name=”skill”/> </subclass> <subclass name="Sales" discriminator-value="2"> <property name="sell"/> </subclass> </class>
实例代码:
package com.dzq.domain; import java.io.Serializable; public class Employee implements Serializable{ private int id; private String name; private Department depart; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Department getDepart() { return depart; } public void setDepart(Department depart) { this.depart = depart; } }
子类:
package com.dzq.domain; public class Sales extends Employee { private int sell; public int getSell() { return sell; } public void setSell(int sell) { this.sell = sell; } }
package com.dzq.domain; public class Skiller extends Employee { private String skiller; public String getSkiller() { return skiller; } public void setSkiller(String skiller) { this.skiller = skiller; } }
映射关系:
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="com.dzq.domain"> <class name="Employee" table="employee" discriminator-value="0"> <id name="id" column="id"> <generator class="native" /> </id> <discriminator column="type"/> <property name="name" column="name" /> <many-to-one name="depart" column="depart_id" /> <subclass name="Skiller" discriminator-value="1"> <property name="skiller"/> </subclass> <subclass name="Sales" discriminator-value="2"> <property name="sell"/> </subclass> </class> </hibernate-mapping>
测试代码:
package com.dzq.test; import java.util.HashSet; import java.util.Set; import org.hibernate.Hibernate; import org.hibernate.Session; import org.hibernate.Transaction; import com.dzq.domain.Department; import com.dzq.domain.Employee; import com.dzq.domain.Sales; import com.dzq.domain.Skiller; import com.dzq.utils.HibernateUntils; public class ManyToOne { public static void main(String[] args) { add(); query(2); } public static void addEmAndDe(){ Employee em=new Employee(); Department depart=new Department(); depart.setName("FBI"); em.setDepart(depart); em.setName("AK47"); HibernateUntils.add(depart); HibernateUntils.add(em); } public static void add(){ Session s=null; Transaction ts=null; try { Department dep=new Department(); dep.setName("FBI"); Employee e1=new Employee(); Skiller e2=new Skiller(); Sales e3=new Sales(); e1.setName("hi"); e1.setDepart(dep); e2.setName("hello"); e2.setDepart(dep); e2.setSkiller("skill"); e3.setName("fuck"); e3.setDepart(dep); e3.setSell(100); Set<Employee> empls=new HashSet<Employee>(); empls.add(e1); empls.add(e2); empls.add(e3); dep.setEmpls(empls); s=HibernateUntils.getSession(); ts=s.beginTransaction(); s.save(dep); s.save(e1); s.save(e2); s.save(e3); ts.commit(); } catch (Exception e) { ts.rollback(); throw new RuntimeException(e); }finally{ if(s!=null){ s.close(); } } } public static Employee query(int id){ Session s=null; try{ s=HibernateUntils.getSession(); Employee emp=(Employee) s.get(Employee.class, id); //Hibernate.initialize(emp.getDepart()); System.out.println(emp.getClass()); return emp; }finally{ if(s!=null){ s.close(); } } } }
表结构
2、每个子类一张表(joined-subclass) (表结构)

每个子类一张表(joined-subclass) (映射文件)
<class name="Employee" table="employee"> <id name="id"> <generator class="native"/> </id> <property name="name"/> <joined-subclass name="Skiller" table="skiller"> <key column="employee_id"/> <property name="skill"/> </joined-subclass> <joined-subclass name="Sales" table="sales"> <key column="employee_id"/> <property name="sell"/> </joined-subclass> </class>
3、混合使用“一个类继承体系一张表”和“每个子类一张表” (表结构)

混合使用“一个类继承体系一张表”和“每个子类一张表” (映射文件)
<class name="Employee" table="employee"> <id name="id"> <generator class="native"/> </id> <discriminator column="type"/> <property name="name"/> <subclass name="Skiller"> <property name="net"/> </subclass> <subclass name=”Sales”"> <join table="sales"> <key column="employee_id"/> <property name="sell"/> </join> </subclass> </class>
数据库结构:
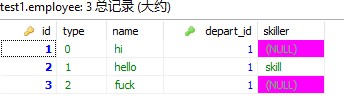
4、每个具体类一张表(union-subclass) (表结构)

每个具体类一张表(union-subclass) (映射文件)
<class name="Employee" abstract="true"> <id name="id"> <generator class="hilo"/> </id> <property name="name"/> <union-subclass name="Skiller" table="skiller"> <property name="skill"/> </union-subclass> <union-subclass name="Sales" table="sales"> <property name="sell"/> </union-subclass> </class>
主健不能是identity类型,如果父类是abstract=”true”就不会有表与之对应。
隐式多态,映射文件没有联系,限制比较多很少使用。