1 <?
2 //节点
3 class Node
4 {
5 private $Data;//节点数据
6
7 private $Next;//下一节点
8
9 public function setData($value)
10 {
11 $this->Data=$value;
12 }
13
14 public function setNext($value)
15 {
16 $this->Next = $value;
17 }
18
19 public function getData()
20 {
21 return $this->Data;
22 }
23
24 public function getNext()
25 {
26 return $this->Next;
27 }
28
29 public function __construct($data, $next)
30 {
31 $this->setData($data);
32 $this->setNext($next);
33 }
34
35 }
36
37 //链表数据
38 class LinkList
39 {
40 private $header;//头节点
41
42 private $size;//长度
43
44 public function getSize()
45 {
46 $i = 0;
47 $node = $this->header;
48 while ($node->getNext() != null) {
49 $i++;
50 $node = $node->getNext();
51 }
52
53 return $i;
54 }
55
56 public function setHeader($value)
57 {
58 $this->header = $value;
59 }
60
61 public function getHeader()
62 {
63 return $this->header;
64 }
65
66 public function __construct()
67 {
68 header("content-type:text/html; charset=utf-8");
69 $this->setHeader(new Node(null,null));
70 }
71
72 /**
73 *@author Marx
74 *@param $data--要添加节点的数据
75 *
76 */
77 public function add($data)
78 {
79 $node = $this->header;
80
81 //var_dump($this->header);
82
83 while($node->getNext() != null) {
84 $node = $node->getNext();
85 }
86
87 $node->setNext(new Node($data,null));
88 }
89
90 /**
91 *@author Marx
92 *@param $data--要移除节点的数据
93 *
94 */
95 public function removeAt($data)
96 {
97 $node = $this->header;
98
99 if ($node->getNext() == null) {
100 return;
101 }
102
103 while ($node->getData() != $data)
104 {
105 $node = $node->getNext();
106 }
107
108 $node->setData($node->getNext()->getData());
109
110
111 $node->setNext($node->getNext()->getNext());
112 }
113
114 /**
115 *@author Marx
116 *@param 遍历
117 *
118 */
119 public function get()
120 {
121 $node = $this->header;
122 if ($node->getNext() == null) {
123 print("数据集为空!");
124 return;
125 }
126
127 while ($node->getNext() != null) {
128 print('['.$node->getNext()->getData().'] -> m');
129 if ($node->getNext()->getNext() == null){break;}
130 $node = $node->getNext();
131 }
132 }
133
134 /**
135 *@author Marx
136 *@param $data--要访问的节点的数据
137 * @param 此方法只是演示不具有实际意义
138 *
139 */
140
141 public function getAt($data)
142 {
143 $node = $this->header->getNext();
144
145 if ($node->getNext() == null) {
146 print("数据集为空!
");
147 return;
148 }
149
150 while ($node->getData() != $data) {
151 if ($node->getNext() == null) {break;}
152
153 $node = $node->getNext();
154 }
155
156 return $node->getData();
157 }
158
159 /**
160 *@author Marx
161 *@param $value--需要更新的节点的原数据 --$initial---更新后的数据
162 *
163 */
164 public function update($initial,$value)
165 {
166 $node = $this->header->getNext();
167
168 if($node->getNext() == null) {
169 print("数据集为空!
");
170
171 return;
172 }
173
174 while ($node->getData() != $initial) {
175 //var_dump($node->getData());
176 //var_dump($initial);
177 echo "
";
178
179 if ($node->getNext() == null) {break;}
180
181 $node = $node->getNext();
182 }
183 //var_dump($node->getData());
184 //var_dump($value);
185
186 $node->setData($value);
187
188 }
189 }
190
191 //迭代反转
192 function ReverseList1(Node $pHeader)
193 {
194 $q = $pHeader->getNext();//首节点没值 只能获取下一个节点 称为【当前节点】
195
196 $p = null;//负责设置【当前】的下一个 next值
197
198 while($q)//【当前】
199 {
200 $r = $q->getNext();//负责拿下一个
201
202 $q->setNext($p);//负责设置【当前】
203
204 $p = $q;//将【更改后的当前】的存下来
205
206 $q = $r;//将一下节点赋给【当前】节点
207 }
208
209 $newNode = new Node(null, $p);
210
211 return $newNode;
212 }
213
214 $lists = new LinkList();//创建链表数据
215
216 $lists->add(1);//增加节点数据 顺序按push来
217
218 $lists->add(2);
219
220 $lists->add(3);
221
222 $haedNode = $lists->getHeader();//获取全量节点
223
224 var_dump($haedNode);//当前节点数据
225
226 $haedNode2 = ReverseList1($haedNode);
227 var_dump($haedNode2);//反转节点数据
反转链表流程图
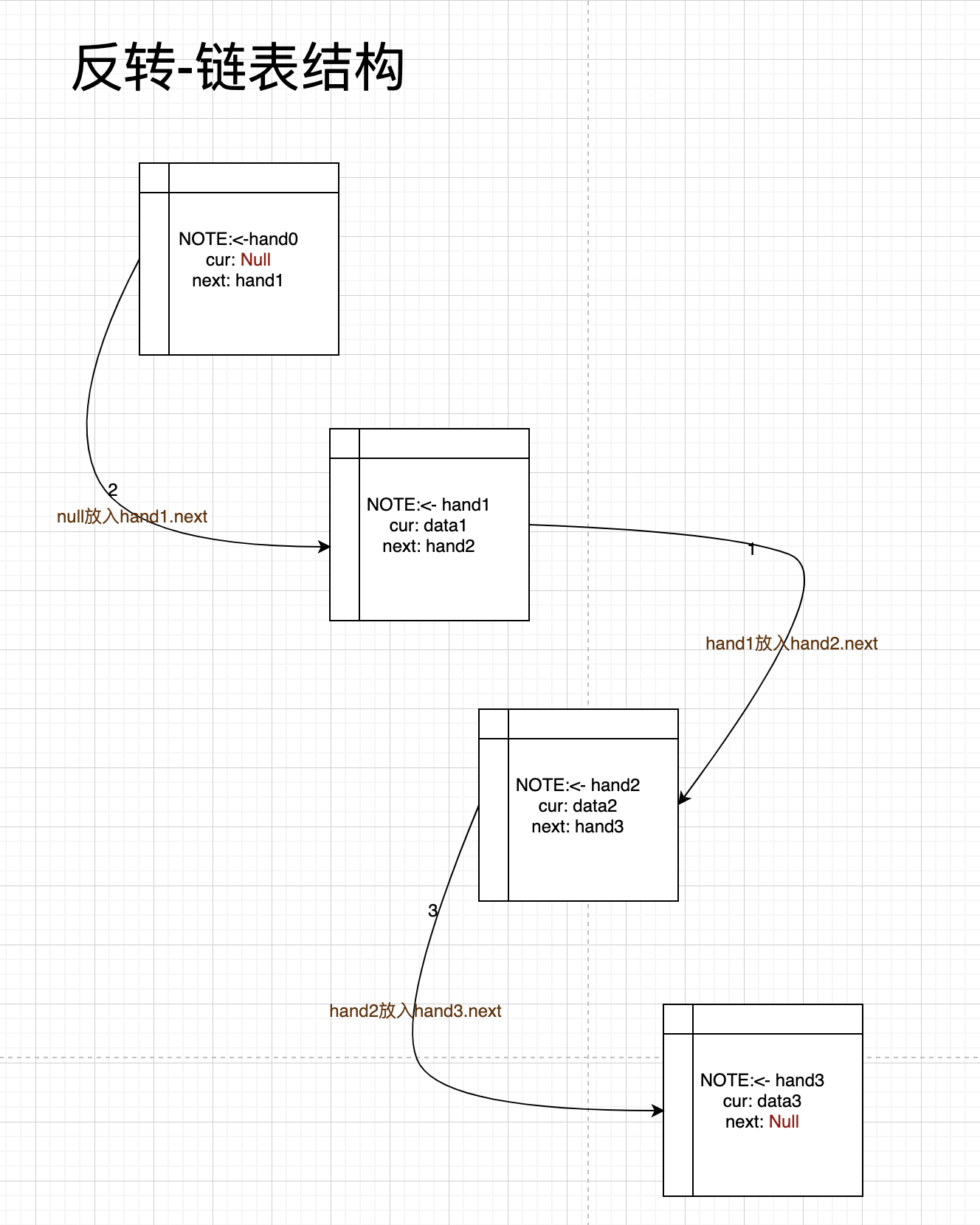