Dart中的库就是具有特定功能的模块,可能包含单个文件,也可能包含多个文件
按照库的作者进行划分,库可以分成三类
(1)、自定义库(开发人员自己写的)
(2)、系统库(Dart 中自带的)
(3)、第三方库(Dart 生态中的)
Dart生态
https://pub.dev/
pub 命令(D:\flutter\bin\cache\dart-sdk\bin)
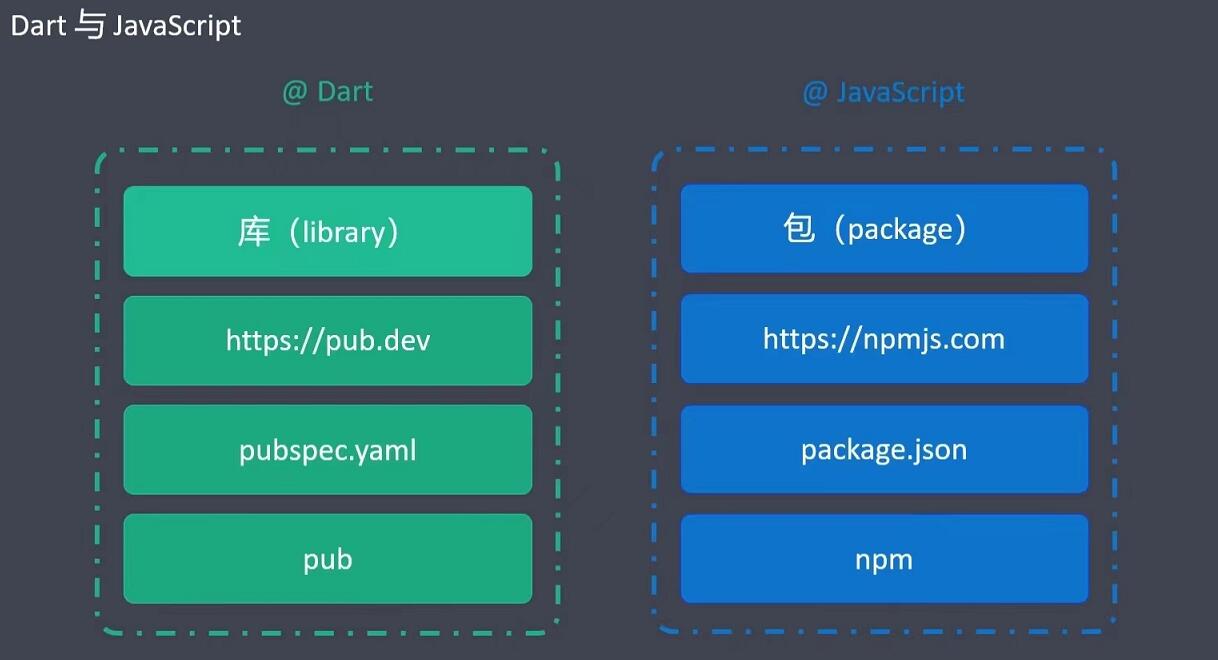
自定义库
通过 library 来声明库
每个 dart 文件默认都是一个库,只是没有使用 library 来显示声明
dart 使用 _(下划线)开头的标识符,表示库内访问可见(即私有属性,在库外访问不可见)
library 关键字声明的库名称建议使用:小写字母 + 下划线
通过 import 来引入库
不同类型的库,引入方式不同
自定义库(import '库的位置/库的名称.dart')
library my_custom; // 建议写成小写字母 + 下划线的形式 class MyCustom { String name = 'MyCustom'; static num version = 1.0; void info() { print('这是自定义库。'); } }
import 'lib/MyCustom.dart'; void main() { MyCustom mc = new MyCustom(); mc.info(); // 这是自定义库。 print(MyCustom.version); // 1.0 }
系统库(import 'dart:库的名称')
// import 'dart:core'; // core 库会被默认引入 import 'dart:math'; void main() { print(pi); // 3.141592653589793 print(max(3, 7)); // 7 print(pow(2, 10)); // 1024 }
引入部分库(仅引入需要的内容)
library common; void foo() { print('common foo is running'); } void bar() { print('common bar is running'); } void baz() { print('common baz is running'); }
包含引入(show)
// show 后面指定包含引入的内容 import 'lib/Common.dart' show bar, baz; void main() { bar(); // common bar is running baz(); // common baz is running // foo(); // Error: Method not found: 'foo'. }
排除引入(hide)
// hide 会隐藏后面的内容 import 'lib/Common.dart' hide bar, baz; void main() { // bar(); // Error: Method not found: 'bar'. // baz(); // Error: Method not found: 'baz'. foo(); // common foo is running }
指定库的前缀
当库名冲突时,可以通过 as 关键字,给库声明一个前缀
library function; void foo() { print('function foo is running'); } void hello() { print('Hello Dart.'); }
import 'lib/Common.dart'; import 'lib/Function.dart'; void main() { // 报错:两个库中包含了同名的 foo 方法 foo(); // The name 'foo' is defined in the libraries 'file:///e:/dart-study/6_Library/lib/Common.dart' // and 'file:///e:/dart-study/6_Library/lib/Function.dart'.Try using 'as prefix' for one of the // import directives, or hiding the name from all but one of the imports. }
// 给库添加前缀,解决命名冲突问题 import 'lib/Common.dart' as com; import 'lib/Function.dart' as fun; void main() { com.foo(); // common foo is running fun.foo(); // function foo is running }
延迟引入(懒加载)
使用 deferred as 关键字来标识需要延时加载的库
import 'lib/Function.dart' deferred as func; Future greet() async { await func.loadLibrary(); func.hello(); } void main() { // 使用 deferred 标识库以后直接使用库提供的方法报错了 // func.foo(); // Error: Deferred library func was not loaded. print('1'); greet(); print('2'); print('3'); // 1 // 2 // 3 // Hello Dart. }
通过 part 与 part of 来组装库
// library camera; // 子库不能使用 library 关键字 // 与主库建立联系 part of phone; class Camera { String name = '摄像头'; void info() { print('打印摄像头信息'); } }
// 与主库建立联系 part of phone; class Processor { String name = '处理器'; void info() { print('打印处理器信息'); } }
library phone; // 与分库建立联系 part 'Camera.dart'; part 'Processor.dart';
import 'lib/phone/main.dart'; void main() { Camera c = new Camera(); Processor p = new Processor(); c.info(); // 打印摄像头信息 p.info(); // 打印处理器信息 }
系统库
系统库(也叫核心库)是 Dart 提供的常用内置库,无需单独下载,可以直接使用
https://dart.cn/guides/libraries
void main() { // 创建当前时间 DateTime now = new DateTime.now(); print(now); // 2022-04-28 14:29:44.776655 // 通过普通构造函数创建时间 DateTime d = new DateTime(2020, 8, 20, 23, 30); print(d); // 2020-08-20 23:30:00.000 // 创建标准时间 // DateTime d1 = DateTime.parse('2019-12-12 8:10:30'); // Error: FormatException: Invalid date format DateTime d1 = DateTime.parse('2019-12-12 08:10:30'); print(d1); // 2019-12-12 08:10:30.000 DateTime d2 = DateTime.parse('2021-01-01 24:00:00+0800'); print(d2); // 2021-01-01 16:00:00.000Z // 时间增量 DateTime now2 = now.add(new Duration(hours: 2)); print(now2); // 2022-04-28 16:42:28.160090 DateTime now3 = now.add(new Duration(days: -1)); print(now3); // 2022-04-27 14:44:23.453962 // 时间比较 bool result1 = d1.isAfter(d2); // d1 是否在 d2之后 print(result1); // false bool result2 = d1.isBefore(d2); // d1 是否在 d2之前 print(result2); // true bool result3 = d1.isAtSameMomentAs(d2); // d1 与 d2 是否相同 print(result3); // false // 时间差 DateTime d3 = new DateTime(2020, 8, 1); DateTime d4 = new DateTime(2022, 8, 1); Duration diff = d3.difference(d4); print(diff); // -17520:00:00.000000 // d3 与 d4相差的天数和小时 print([diff.inDays, diff.inHours]); // [-730, -17520] // 时间戳 int s1 = now.millisecondsSinceEpoch; // 单位:毫秒,13位时间戳 print(s1); // 1651129485493 int s2 = now.microsecondsSinceEpoch; // 单位:毫秒,16位时间戳 print(s2); // 1651129555247676 // 格式化 String month = now.month.toString().padLeft(2, '0'); print(month); // 04 String timestamp = '${now.year.toString()}-${now.month.toString()}-${now.day.toString().padLeft(2, "0")} ${now.hour.toString().padLeft(2, "0")}:${now.minute.toString().padLeft(2, "0")}'; print(timestamp); // 2022-4-28 15:15 }
第三方库
来源
https://pub.dev/
https://pub.flutter-io.cn/packages
使用
在项目目录下创建 pubspec.yaml
在 pubspec.yaml 中声明第三方库(依赖)
命令行中进入 pubspec.yaml 所在目录,执行 pub get 进行安装
项目中引入已按照的第三方库(import 'package:xxx/xxx.dart';)