上例图:
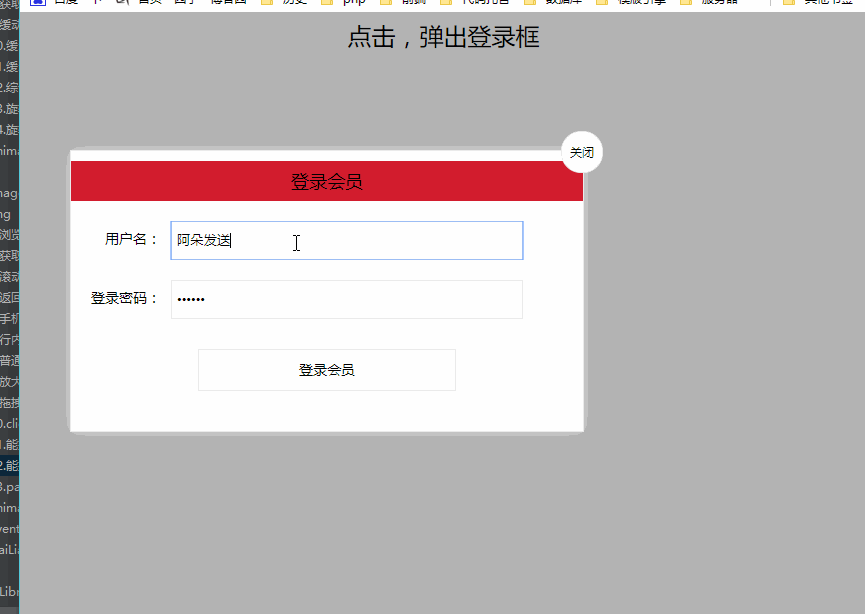
代码块:
1 <!DOCTYPE html>
2 <html>
3 <head lang="en">
4 <meta charset="UTF-8">
5 <title></title>
6 <style>
7 .login-header {
8 100%;
9 text-align: center;
10 height: 30px;
11 font-size: 24px;
12 line-height: 30px;
13 }
14 ul, li, ol, dl, dt, dd, div, p, span, h1, h2, h3, h4, h5, h6, a {
15 padding: 0px;
16 margin: 0px;
17 }
18 .login {
19 512px;
20 position: absolute;
21 border: #ebebeb solid 1px;
22 height: 280px;
23 left: 50%;
24 right: 50%;
25 background: #ffffff;
26 box-shadow: 0px 0px 20px #ddd;
27 z-index: 9999;
28 margin-left: -250px;
29 margin-top: 140px;
30 transform: translateY(-500px) scale(0);
31 transition: transform .4s cubic-bezier(0.98, 0.09, 0.4, 1.3);
32 }
33 .login-title {
34 100%;
35 margin: 10px 0px 0px 0px;
36 text-align: center;
37 line-height: 40px;
38 height: 40px;
39 font-size: 18px;
40 position: relative;
41 cursor: move;
42 -moz-user-select:none;/*火狐*/
43 -webkit-user-select:none;/*webkit浏览器*/
44 -ms-user-select:none;/*IE10*/
45 -khtml-user-select:none;/*早期浏览器*/
46 user-select:none;
47 background: #d21c2e;
48 }
49 .login-input-content {
50 margin-top: 20px;
51 }
52 .login-button {
53 50%;
54 margin: 30px auto 0px auto;
55 line-height: 40px;
56 font-size: 14px;
57 border: #ebebeb 1px solid;
58 text-align: center;
59 }
60 .login-bg {
61 100%;
62 height: 100%;
63 position: fixed;
64 top: 0px;
65 left: 0px;
66 background: #000000;
67 filter: alpha(opacity=30);
68 -moz-opacity: 0.3;
69 -khtml-opacity: 0.3;
70 opacity: 0.3;
71 display: none;
72
73 }
74 a {
75 text-decoration: none;
76 color: #000000;
77 }
78 .login-button a {
79 display: block;
80 }
81 .login-input input.list-input {
82 float: left;
83 line-height: 35px;
84 height: 35px;
85 350px;
86 border: #ebebeb 1px solid;
87 text-indent: 5px;
88 }
89 .login-input {
90 overflow: hidden;
91 margin: 0px 0px 20px 0px;
92 }
93 .login-input label {
94 float: left;
95 90px;
96 padding-right: 10px;
97 text-align: right;
98 line-height: 35px;
99 height: 35px;
100 font-size: 14px;
101 }
102 .login-title span {
103 position: absolute;
104 font-size: 12px;
105 right: -20px;
106 top: -30px;
107 background: #ffffff;
108 border: #ebebeb solid 1px;
109 40px;
110 height: 40px;
111 border-radius: 20px;
112 }
113 body.show-login .login{
114 display:block;
115 transform: translateY(0) scale(1);
116 }
117
118 body.show-login .login-bg {
119 display: block;
120 }
121 .login.on {
122 margin-left: 0;
123 margin-top: 0;
124 }
125
126 </style>
127 </head>
128 <body>
129 <div class="login-header"><a id="link" href="javascript:void(0);">点击,弹出登录框</a></div>
130 <div id="login" class="login" >
131 <div id="title" class="login-title">登录会员
132 <span id="closeBtn"><a href="javascript:void(0);" class="close-login">关闭</a></span></div>
133 <div class="login-input-content">
134 <div class="login-input">
135 <label>用户名:</label>
136 <input type="text" placeholder="请输入用户名" name="info[username]" id="username" class="list-input">
137 </div>
138 <div class="login-input">
139 <label>登录密码:</label>
140 <input type="password" placeholder="请输入登录密码" name="info[password]" id="password" class="list-input">
141 </div>
142 </div>
143 <div id="loginBtn" class="login-button"><a href="javascript:void(0);" id="login-button-submit">登录会员</a></div>
144 </div>
145 <div id="bg" class="login-bg" ></div>
146 <script>
147 /**
148 * 获取元素样式函数
149 * @param element 要获取的样式的对象
150 * return 目标css样式对象
151 * */
152 function getStyle(element) {
153 if(window.getComputedStyle) {
154 return window.getComputedStyle(element,null);
155 }else if(element.currentStyle){
156 return element.currentStyle;
157 }
158 }
159
160 /**
161 * 鼠标按下
162 * 标记可以被拖动
163 * 鼠标移动
164 * 让盒子跟着鼠标移动
165 * 鼠标弹起
166 * 标记不能被拖动
167 */
168 var link = document.getElementById('link');
169 var closeBtn = document.getElementById('closeBtn');
170 var bg = document.getElementById('bg');
171 var title = document.getElementById('title');
172 var login = document.getElementById('login');
173 link.onclick = function () {
174 document.body.className = 'show-login';
175 // login.style.left = window.innerWidth/ 2;
176 // login.style.top = window.innerHeight / 2;
177 }
178
179 closeBtn.onclick = function () {
180 document.body.className = '';
181 }
182 bg.onclick = function () {
183 document.body.className = '';
184 }
185
186 // 开关思想
187 var flag = false;
188 document.onmouseup = function () {
189 flag = false;
190 }
191 title.onmousedown = function () {
192 flag = true;
193
194 }
195
196 var boxWidth = parseInt(getStyle(login).width);
197 var boxHeight = parseInt(getStyle(login).height);
198 document.onmousemove = function (event) {
199 if(flag) {
200 login.className = "login on";
201 var pageX = event.pageX - boxWidth/2 ;
202 var pageY = event.pageY - 30;
203
204 if(pageX < 50) {
205 pageX = 50;
206 }
207 if(pageY < 50) {
208 pageY = 50;
209 }
210 if(pageX > window.innerWidth - boxWidth) {
211 pageX = window.innerWidth - boxWidth;
212 }
213 if(pageY > window.innerHeight - boxHeight) {
214 pageY = window.innerHeight - boxHeight;
215 }
216 login.style.left = pageX + "px";
217 login.style.top = pageY + "px";
218
219
220 }
221 }
222 </script>
223
224 </body>
225 </html>