何为引用...给已有的变量取别名
int num = 10; int &a = num;//此处 &不是取地址 而是标明 a是引用变量(a 是 num的别名)
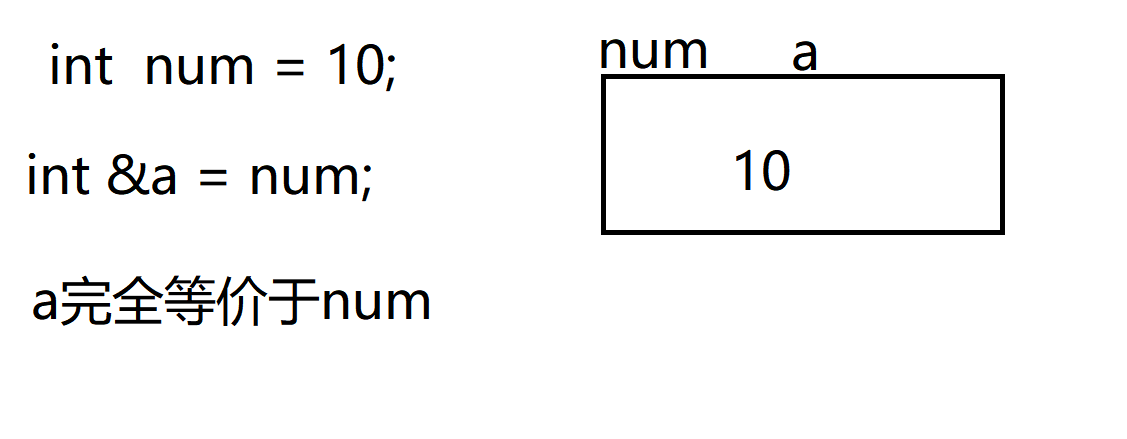
注意:
1、引用必须初始化
2、引用一旦初始化 就不能再次修改别名
int num = 10; int &a = num; int data = 20; a = data;//不是data别名为a 而是将data值赋值a(num)
案例:
int num = 10; int &a = num;//a就是num的别名 a==num cout<<"num = "<<num<<endl;//10 //对a赋值 == 对num赋值 a=100; cout<<"num = "<<num<<endl;//100 //a是num的别名 所以num和a具有相同的地址空间 cout<<"a 的地址:"<<&a<<endl; cout<<"num 的地址:"<<&num<<endl;
了解完如何简单应用引用,接着来深挖【引用--给数组取个别名】
1 void test02() 2 { 3 int arr[5] = {10,20,30,40,50}; 4 //需求:给arr起个别名 5 int (&my_arr)[5] = arr;//my_arr就是数组arr的别名 6 7 int i=0; 8 for(i=0;i<5;i++) 9 { 10 cout<<my_arr[i]<<" "; 11 } 12 cout<<endl; 13 }
还有,引用作为函数的参数
void my_swap1(int a,int b) { int tmp = a; a = b; b=tmp; } void my_swap2(int *a,int *b)//a=&data1,b =data2; { int tmp = *a; *a = *b; *b = tmp; } void my_swap3(int &a, int &b)//a=data1,b=data2 { int tmp = a; a = b; b= tmp; } void test04() { int data1 = 10,data2=20; cout<<"data1 = "<<data1<<", data2 = "<<data2<<endl; //my_swap1(data1,data2);//交换失败 //my_swap2(&data1,&data2);//交换成功 my_swap3(data1,data2);//交换成功(推荐) cout<<"data1 = "<<data1<<", data2 = "<<data2<<endl; }
指针的引用
#include<stdlib.h> #include<string.h> void my_str1(char **p_str)//p_str = &str { //*p_str == *&str == str *p_str = (char *)calloc(1,32); strcpy(*p_str, "hello world"); return; } void my_str2(char* &my_str)//char* &my_str = str;my_str等价str { my_str = (char *)calloc(1,32); strcpy(my_str, "hello world"); return; } void test07() { char *str = NULL; //需求:封装一个函数 从堆区 给str申请一个空间 并赋值为"hello world" //my_str1(&str); my_str2(str); cout<<"str = "<<str<<endl; free(str); }
引用的本质在c++内部实现是一个指针常量. Type& ref = val; // Type* const ref = &val;
c++编译器在编译过程中使用常指针作为引用的内部实现,因此引用所占用的空间大小与指针相同,只是这个过程是编译器内部实现,用户不可见
int data = 10; int &a = data;//a就是data的别名 //编译器内存转换:int * const a = &data; a=100;//等价于data=100 //*a = 100;//*a == data