1 Spring Cloud简述及简单入门实例
1.1 概述
Spring Cloud基于Spring Boot,提供了多个组件来帮助企业进行微服务系统建设;它提供的主要功能有:服务注册中心/服务注册/服务调用/负载均衡/断路器等;一般情况下它都是使用已有的开源软件,在其基础上按Spring Boot的理念来进行封装,简化各种个组件的调用以及各个组件之间的交互。
1.2 常用组件
Spring Cloud主要包含以下常用组件:Eureka或nacos、Ribbon、Feign
1.2.1 Eureka
分成两类:
(1)一是注册中心及EurekaServer,用于提供服务注册/服务申请等功能;
(2)一是被注册者及服务提供者EurekaClient,用于向EurekaServer注册服务并可从EurekaServer获取需要调用的服务地址信息;
需要向外提供服务的应用,需要使用EurekaClient来向Server注册服务。
1.2.2 Ribbon
负责进行客户端负载均衡的组件;一般与RestTemplate结合,在访问EurekaClient提供的服务时进行负载均衡处理。
也就是说,Ribbon用于服务调用者向被调用者进行服务调用,并且如果服务者有多个节点时,会进行客户端的负载均衡处理;
1.2.3 Feign
与Ribbon功能类型,用于调用方与被调用方的服务调用,同时进行客户端负载均衡的处理;不过它能提供类似本地调用的方式调用远程的EurekaClient提供的服务;它实际上是在Ribbon基础上进行了进一步的封装来提高调用服务的简便性。
1.3 使用示例
假设现在有springcloud-eurekaClient和springcloud-eurekaClient2向外提供服务,该服务同时部署两个节点;Client通过Feign或者是Ribbon调用其提供的服务,其部署关系及数据流向图如下所示:
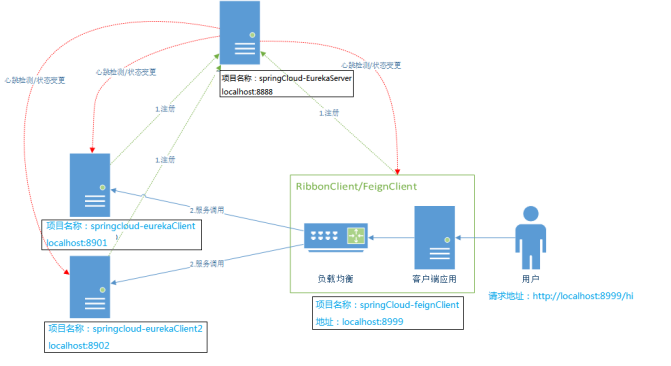
第一步:启动注册中心(启动springCloud-EurekaServer项目);服务提供者及调用者向服务中心注册;
第二步:服务调用者向服务中心申请服务,根据服务中心返回的地址信息调用服务提供者提供的服务;
第三步:注册中心通过心跳检测方式检测服务提供者的状态,当某个提供者连接不上时,发送消息通知所有注册者;
下面详细说明每个节点的实现; 具体代码见
1.4 Eureka服务端(springCloud-EurekaServer)
新建springCloud-EurekaServer Maven工程,具体目录结构如下图
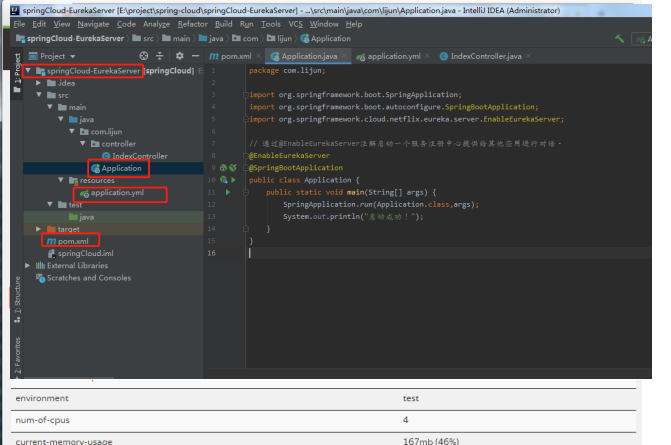
1.4.1 pom.xml配置
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId> <artifactId>springCloud</artifactId> <version>1.0-SNAPSHOT</version> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.4.RELEASE</version> <relativePath /> </parent>
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties>
<dependencies> <!-- eureka --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka-server</artifactId> </dependency> </dependencies>
<!-- Spring Cloud --> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Dalston.SR4</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> </plugins> </build>
</project>
|
1.4.2 Application.yml配置
server: port: 8888
#应用的名字 spring: application: name: springCloud-EurekaServer eureka: instance: hostname: localhost client: registerWithEureka: false # 由于该应用为注册中心,所以设置为false,代表不向注册中心注册自己 fetchRegistry: false # 由于注册中心的职责就是维护服务实例,他并不需要去检索服务,所以也设置为false serviceUrl: defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/
|
1.4.3 Application.cs文件
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
// 通过@EnableEurekaServer注解启动一个服务注册中心提供给其他应用进行对话。 @EnableEurekaServer @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class,args); System.out.println("启动成功!"); } }
|
1.4.4 启动结果
启动完后通过http://localhost:8888/可以看到EurekaServer的监控页面; 此时还没有节点注册,如下图;
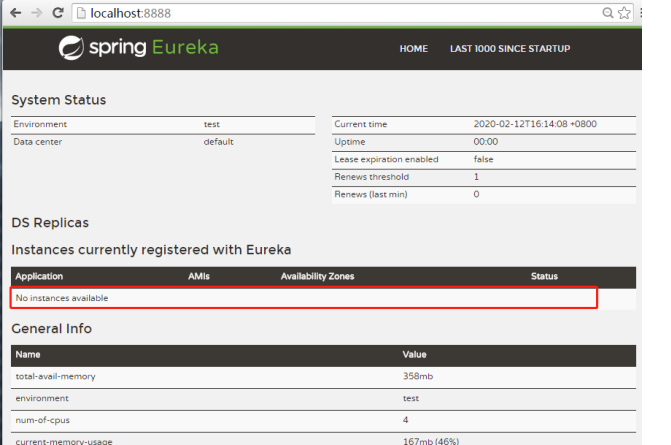
1.5 Eureka服务提供方(springcloud-eurekaClient)
新建springcloud-eurekaClient Maven工程,具体目录结构如下图
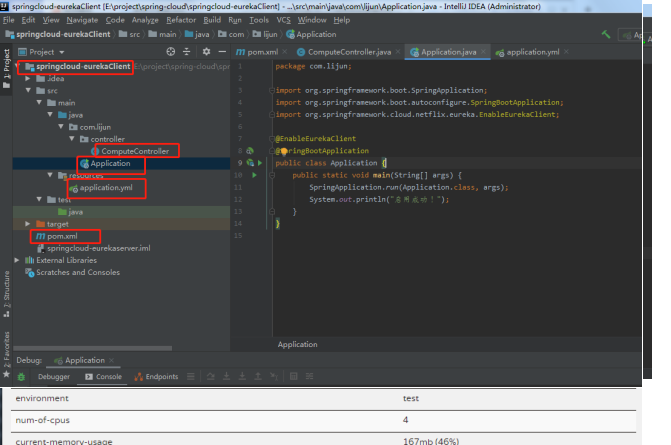
1.5.1 pom.xml配置
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId> <artifactId>springcloud-eurekaserver</artifactId> <version>1.0-SNAPSHOT</version>
<!-- Spring Boot --> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.4.RELEASE</version> <relativePath /> </parent>
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies>
<!-- Spring Cloud --> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Dalston.SR4</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> </project>
|
1.5.2 Application.yml配置
server: port: 8901 #应用的名字 spring: application: name: springcloud-eurekaClient eureka: client: serviceUrl: defaultZone: http://localhost:8888/eureka/
|
1.5.3 Application.cs文件
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@EnableEurekaClient @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); System.out.println("启用成功!"); } }
|
1.5.4 ComputeController.cs文件
package com.lijun.controller;
import org.apache.log4j.Logger; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController;
@RestController public class ComputeController { private final Logger logger = Logger.getLogger(getClass());
@RequestMapping(value = "/add" ,method = RequestMethod.GET) public Integer add(@RequestParam Integer a, @RequestParam Integer b) { Integer r = a + b; logger.info("/add, result:" + r); return r; } @GetMapping("/hello") public String sayHi(@RequestParam String name){ return " Welcome-: "+name; } }
|
1.5.5 启动结果
启动main函数后,通过http://localhost:8888可以看到已经有节点注册;
如果要启动多个节点做负载均衡,可以修改application.yml中的端口信息后再次启动main函数就可以了。
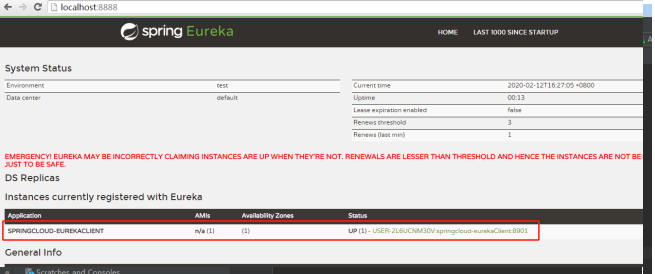
1.6 调用方(springCloud-feignClient)
新建springCloud-feignClient Maven工程,具体目录结构如下图
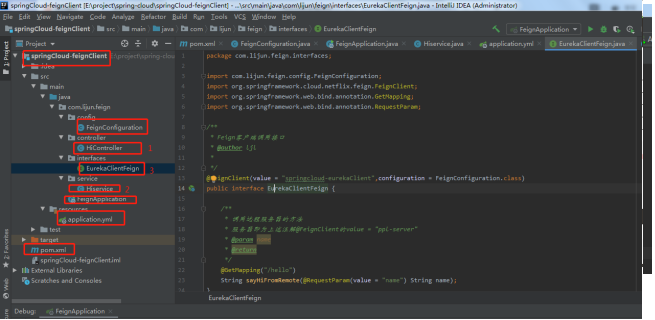
1.6.1 pom.xml配置
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId> <artifactId>springCloud-feignClient</artifactId> <version>1.0-SNAPSHOT</version>
<!-- Spring Boot --> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.4.RELEASE</version> <relativePath /> </parent>
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties>
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency>
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-feign</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies>
<!-- Spring Cloud --> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Dalston.SR4</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
</project>
|
1.6.2 Application.yml配置
server: port: 8999 #应用的名字 spring: application: name: springCloud-feignClient eureka: client: serviceUrl: defaultZone: http://localhost:8888/eureka/
|
1.6.3 FeignApplication.cs文件
package com.lijun.feign;
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; import org.springframework.cloud.netflix.feign.EnableFeignClients;
/** * 在启动类上加@EnableFeignClients注解, * 如果你的Feign接口定义跟你的启动类不在一个包名下,还需要制定扫描的包 * 名@EnableFeignClients(basePackages = "com.lijun.api.client") * @author ljl * */ @SpringBootApplication @EnableEurekaClient @EnableFeignClients public class FeignApplication {
public static void main(String[] args) {
SpringApplication.run(FeignApplication.class, args); System.out.println("启动成功"); } }
|
1.6.4 FeignConfiguration.cs文件
package com.lijun.feign.config;
import feign.Retryer; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration;
import java.util.concurrent.TimeUnit;
/** * 自定义配置类 * @author ljl * */ @Configuration public class FeignConfiguration { /** * 覆盖默认的Retryer的Bean,重试间隔为100毫秒,最大重试时间为1秒,重试次数5次 * @return */ @Bean public Retryer feignRetryer(){ return new Retryer.Default(100, TimeUnit.SECONDS.toMillis(1), 5); }
}
|
1.6.5 EurekaClientFeign.cs文件
package com.lijun.feign.interfaces;
import com.lijun.feign.config.FeignConfiguration; import org.springframework.cloud.netflix.feign.FeignClient; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam;
/** * Feign客户端调用接口 * @author ljl * */ @FeignClient(value = "springcloud-eurekaClient",configuration = FeignConfiguration.class) public interface EurekaClientFeign {
/** * 调用远程服务器的方法 * 服务器即为上述注解@FeignClient的value = "ppl-server" * @param name * @return */ @GetMapping("/hello") String sayHiFromRemote(@RequestParam(value = "name") String name); }
|
1.6.6 Hiservice.cs文件
package com.lijun.feign.service;
import com.lijun.feign.interfaces.EurekaClientFeign; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service;
@Service public class Hiservice {
@Autowired EurekaClientFeign eureClientFeign; public String sayHi(String name){ return eureClientFeign.sayHiFromRemote(name); } }
|
1.6.7 HiController.cs文件
package com.lijun.feign.controller;
import com.lijun.feign.service.Hiservice; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.RestController;
@RestController public class HiController {
@Autowired Hiservice hiService; /** * 在该接口调用了Hiservice的sayHi()方法,Hiservice通过接口EurekaClientFeign远程 * 调用 springcloud-eurekaClient 项目 ComputeController.cs中的 "/hello" * @param name * @return */ @GetMapping("/hi") public String sayHi(@RequestParam(defaultValue="Feifn-client-test",required=false)String name){ return hiService.sayHi(name); } }
|
1.6.8 启动结果
启动main方法后,通过EurekaServer的监控页面可以看到又多了一个名称为SPRINGCLOUD-FEIGNCLIENT的节点; 此时可以通过http://localhost:8999/hi来查看执行结果。
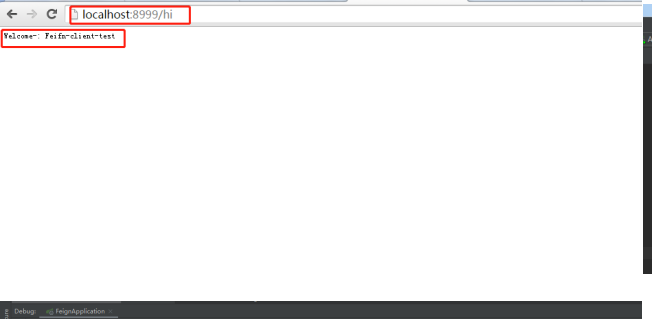
1.7 结束
这就是spring cloud 的简单示例,在此感谢 https://blog.csdn.net/icarusliu/article/details/79461012 博主,如果需要代码,请关注公众号,发送“springcloud”,获取下载地址
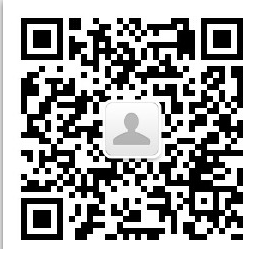