2020-04-07
设计哈希集合
不使用任何内建的哈希表库设计一个哈希集合 具体地说,你的设计应该包含以下的功能
- add(value):向哈希集合中插入一个值。
- contains(value) :返回哈希集合中是否存在这个值。
- remove(value):将给定值从哈希集合中删除。如果哈希集合中没有这个值,什么也不做。
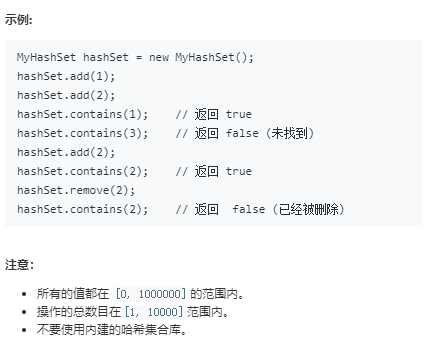
题解:
思路1: 使用数组设计哈希集合 (不建议)
之所以不建议是因为性能差 每次add,contains或者delete都要遍历看看这个值是不是存在
// 使用数组做hash /** * Initialize your data structure here. */ var MyHashSet = function () { this.hashContent = []; }; /** * @param {number} key * @return {void} */ MyHashSet.prototype.add = function (key) { const data = this.hashContent; for (let i = 0; i < data.length; i++) { if (data[i] === key) return null; } data[data.length] = key; }; /** * @param {number} key * @return {void} */ MyHashSet.prototype.remove = function (key) { const data = this.hashContent; for (let i = 0; i < data.length; i++) { if (data[i] === key) data.splice(i, 1); } }; /** * Returns true if this set contains the specified element * @param {number} key * @return {boolean} */ MyHashSet.prototype.contains = function (key) { const data = this.hashContent; for (let i = 0; i < data.length; i++) { if (data[i] === key) return true; } return false; }; /** * Your MyHashSet object will be instantiated and called as such: * var obj = new MyHashSet() * obj.add(key) * obj.remove(key) * var param_3 = obj.contains(key) */
2: 使用对象设计哈希集合
对象免去了遍历的烦恼 性能更高 代码更简单易读
/** * Initialize your data structure here. */ var MyHashSet = function () { this.hashContent = {}; }; /** * @param {number} key * @return {void} */ MyHashSet.prototype.add = function (key) { this.hashContent[key] = true; }; /** * @param {number} key * @return {void} */ MyHashSet.prototype.remove = function (key) { this.hashContent[key] && delete this.hashContent[key]; }; /** * Returns true if this set contains the specified element * @param {number} key * @return {boolean} */ MyHashSet.prototype.contains = function (key) { if (this.hashContent[key]) return true; return false; }; /** * Your MyHashSet object will be instantiated and called as such: * var obj = new MyHashSet() * obj.add(key) * obj.remove(key) * var param_3 = obj.contains(key) */