为什么需要babel?
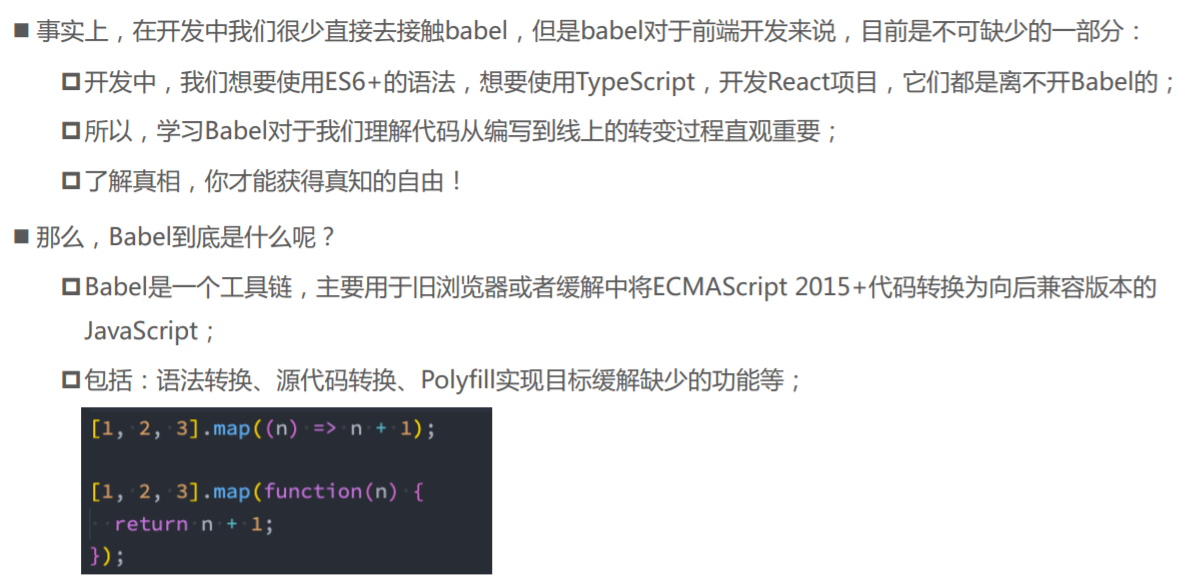
Babel命令行使用
- npm install @babel/cli @babel/core
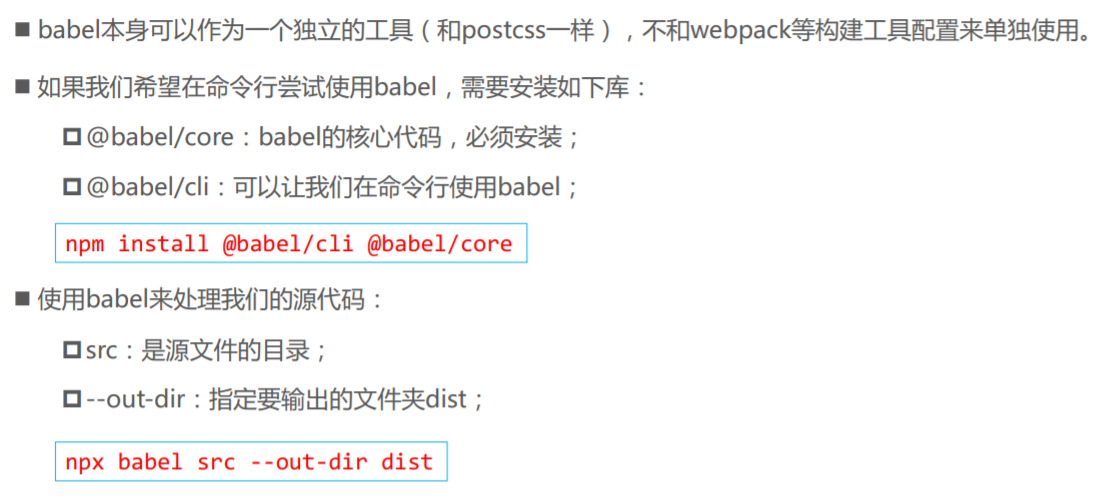
插件的使用
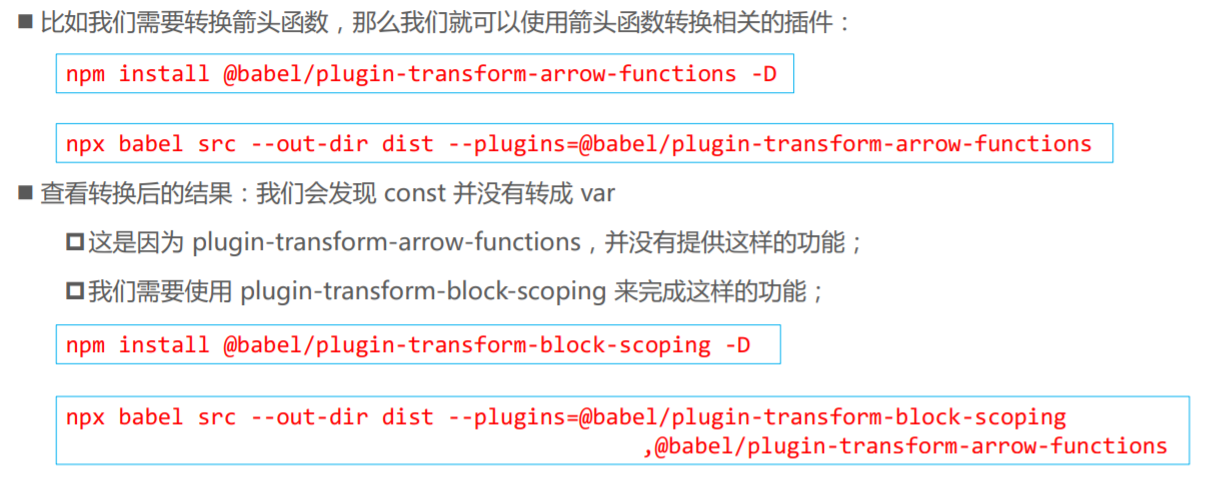
Babel的预设preset
- cnpm install @babel/preset-env -D
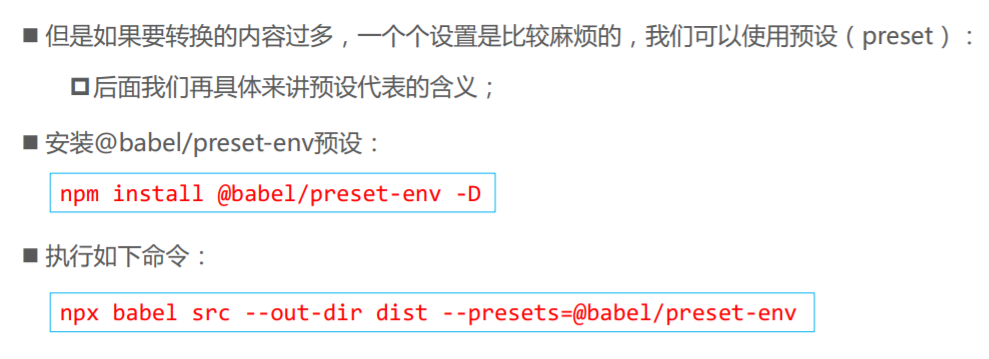
Babel的底层原理
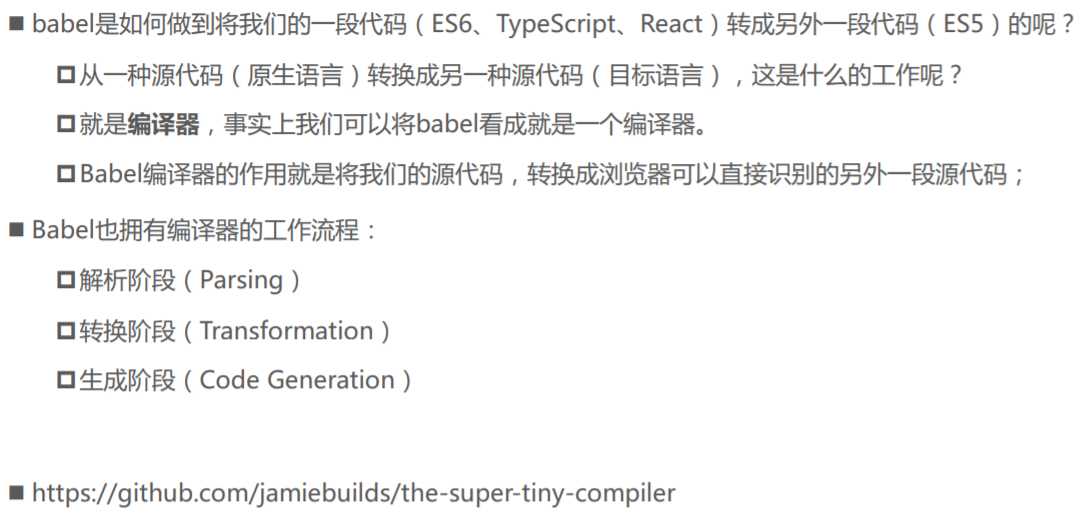
babel编译器执行原理
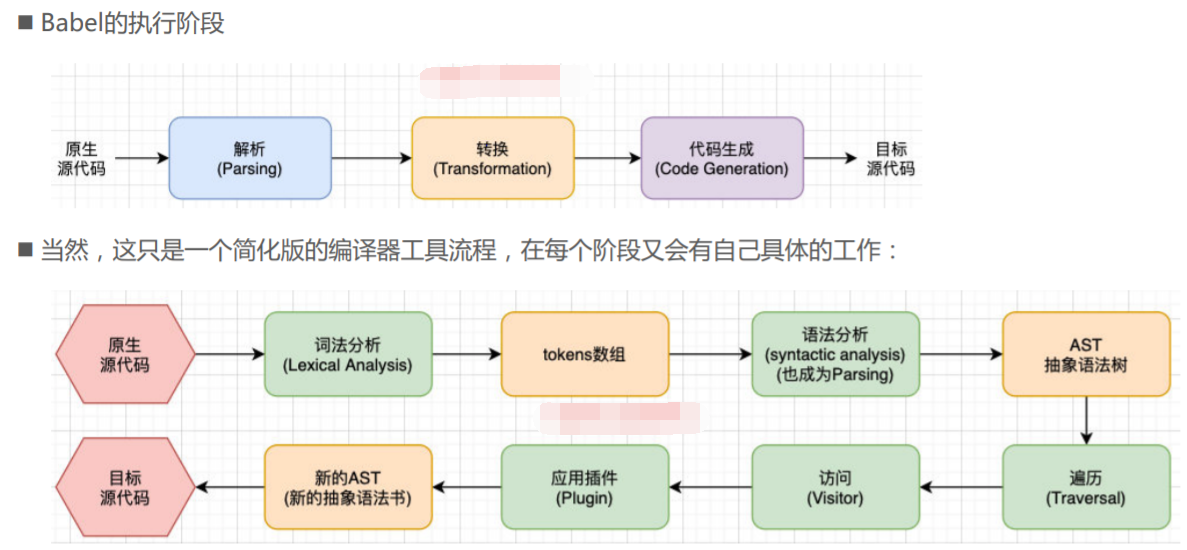
中间产生的代码
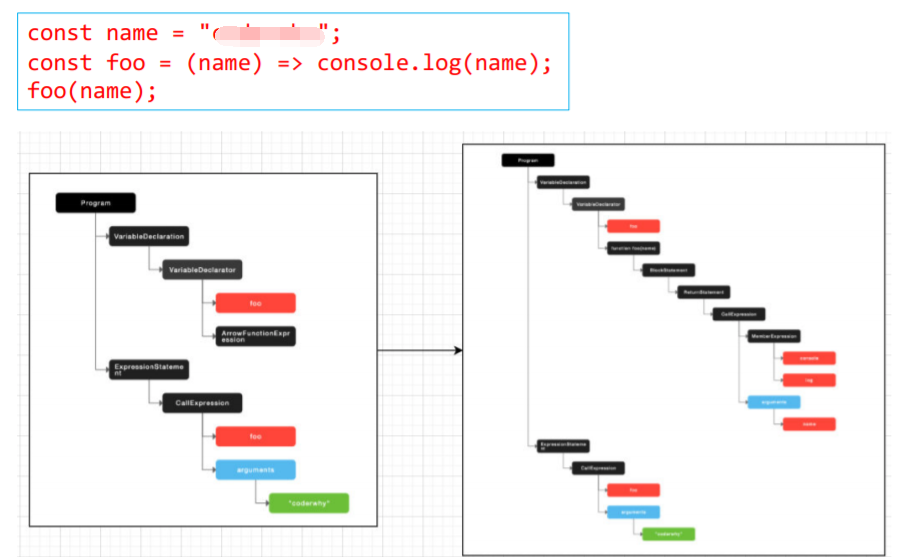
babel-loader
- npm install babel-loader @babel/core
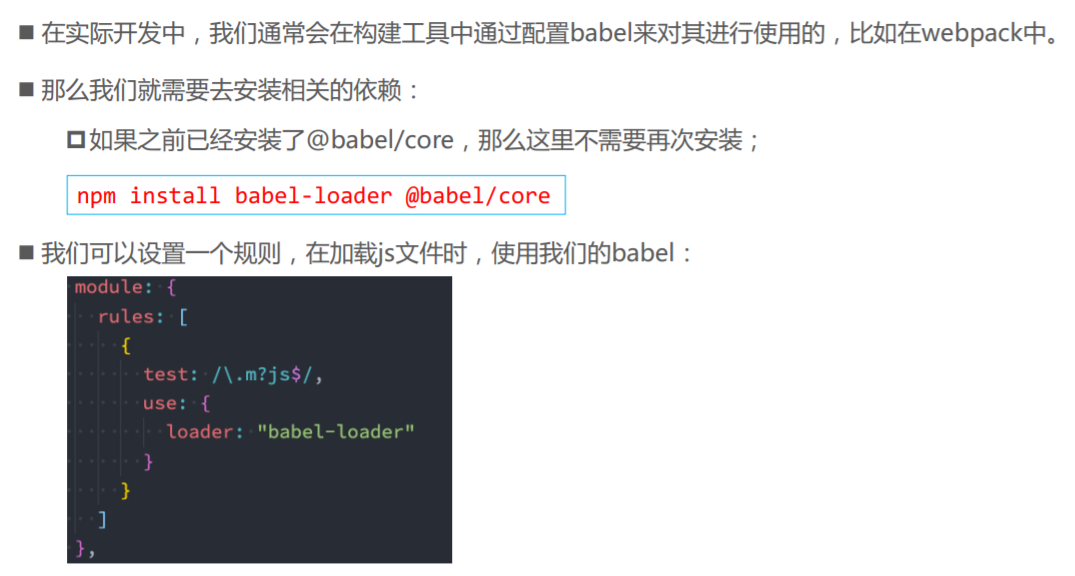
指定使用的插件
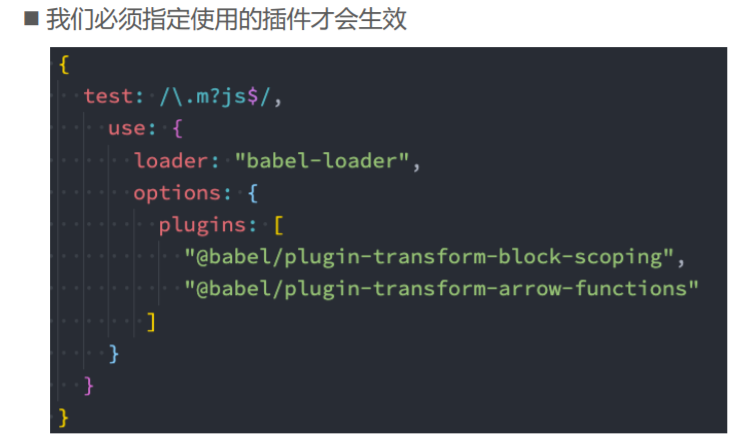
babel-preset
- npm install @babel/preset-env
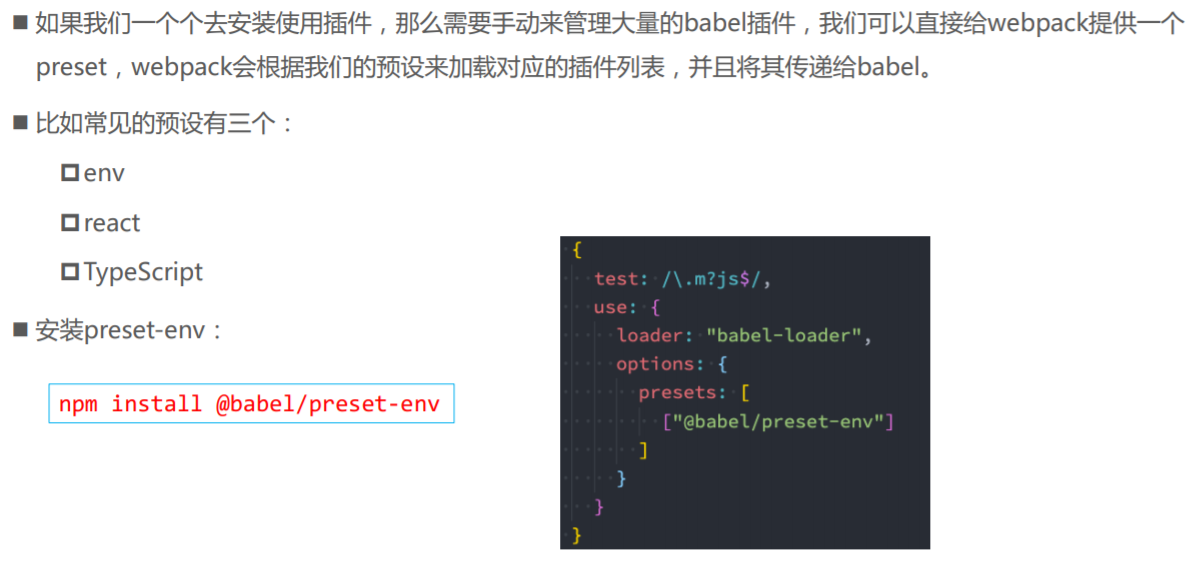
设置目标浏览器 browserslist
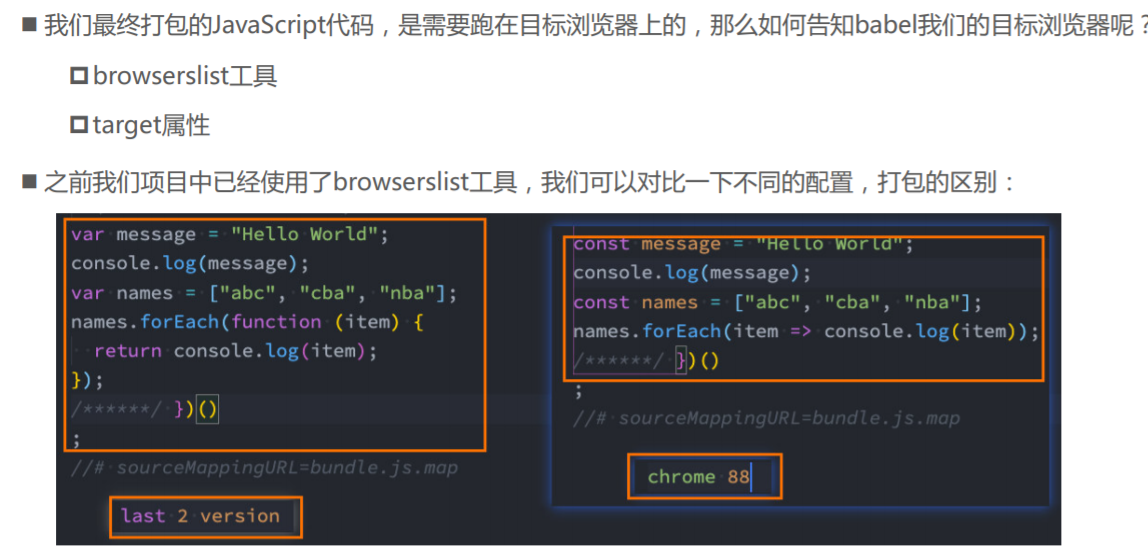
设置目标浏览器 targets
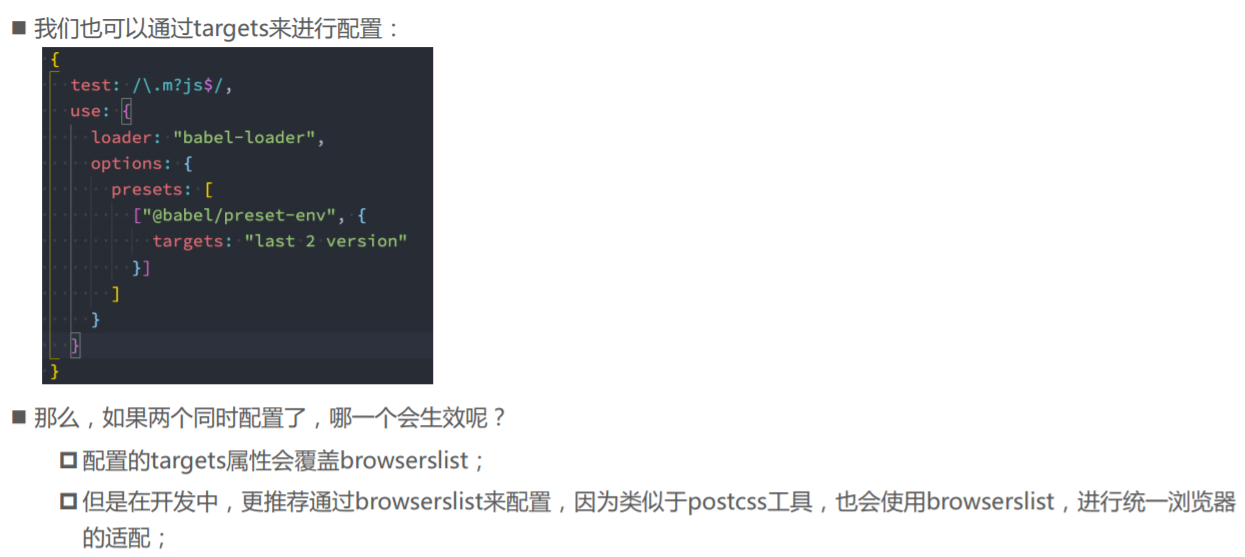
Stage-X的preset
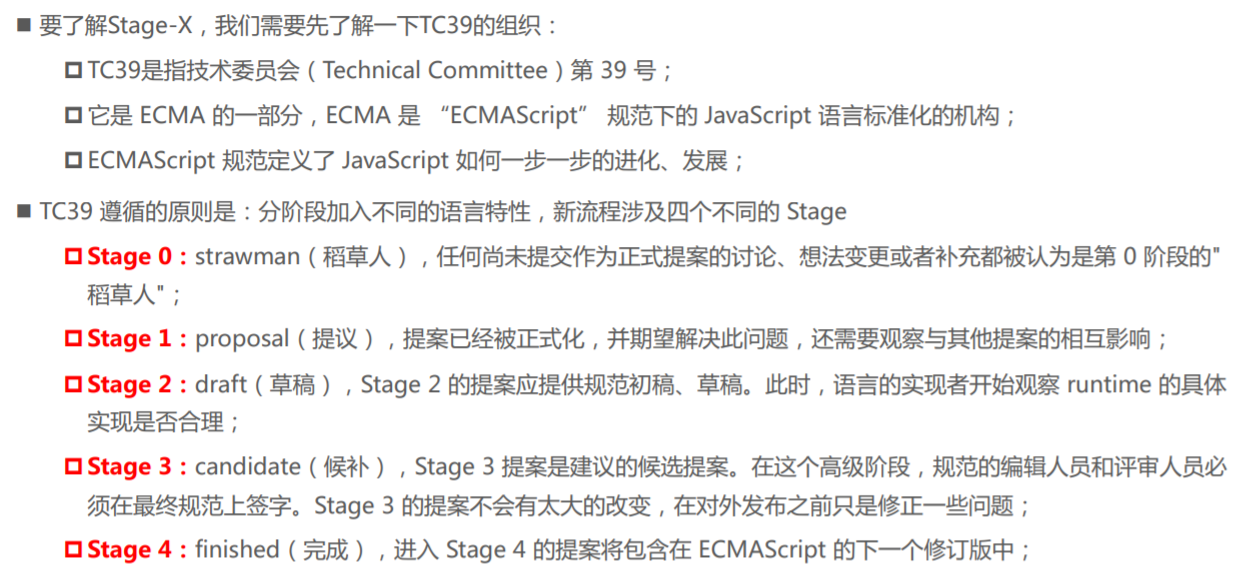
Babel的Stage-X设置
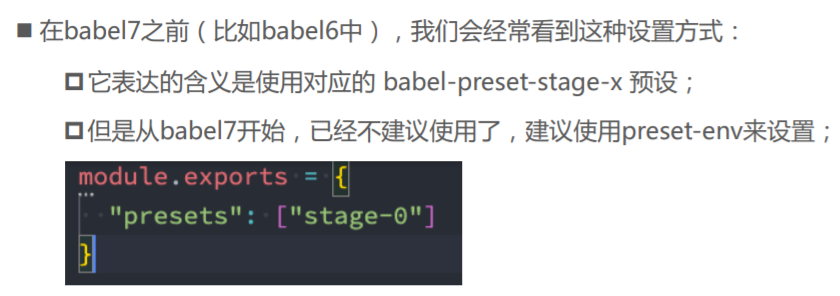
Babel的配置文件
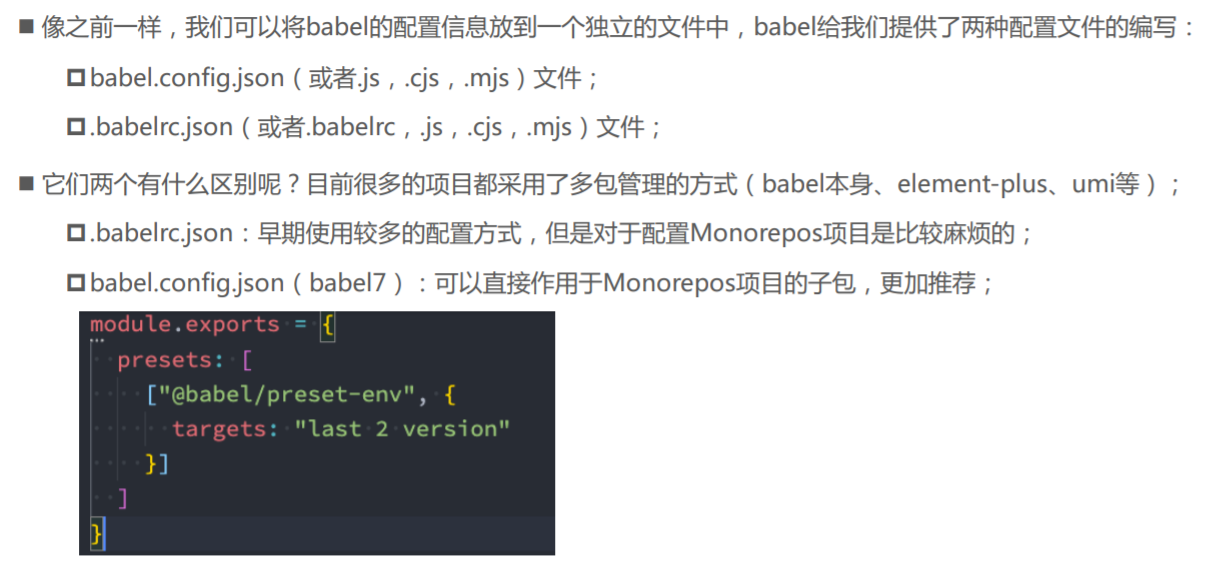
认识polyfill
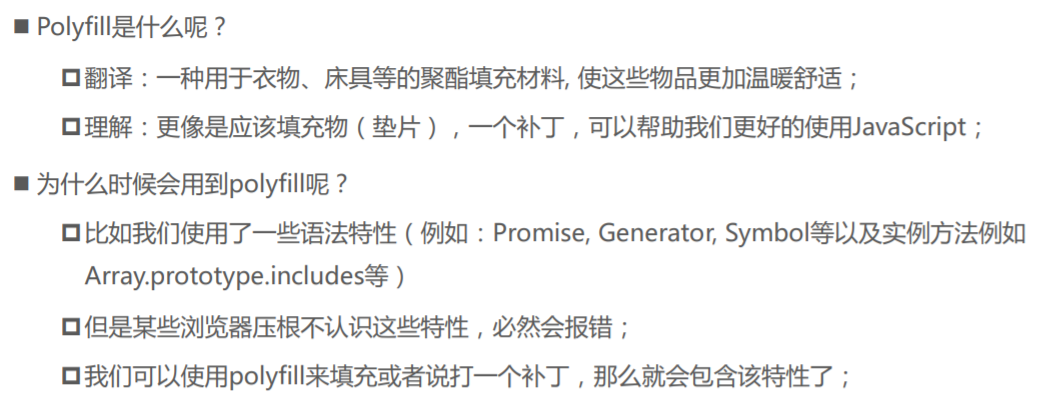
如何使用polyfill?
- cnpm install core-js regenerator-runtime --save
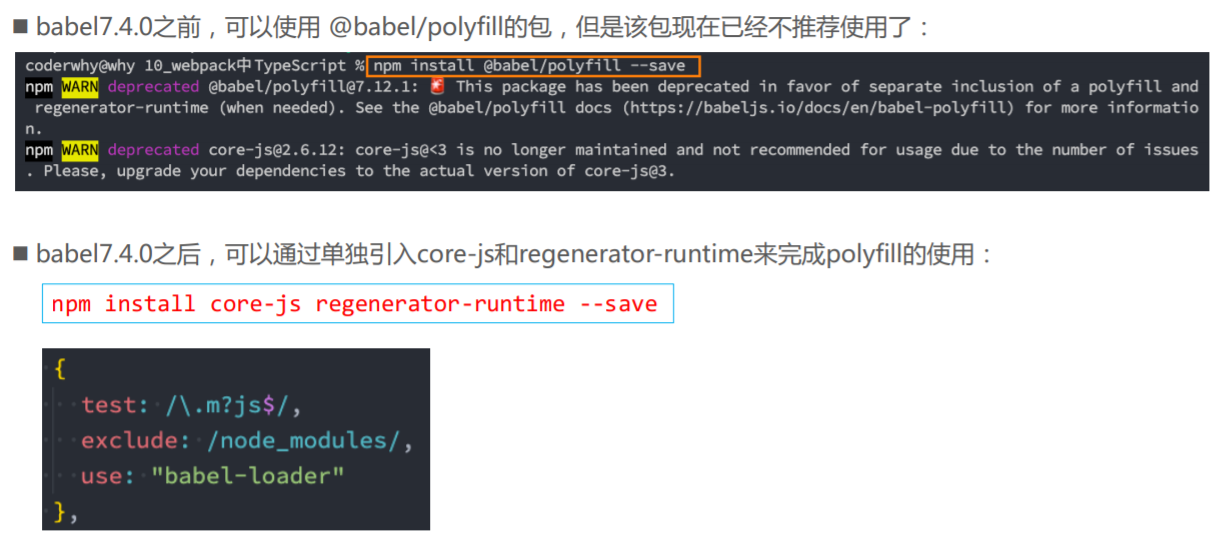
配置babel.config.js
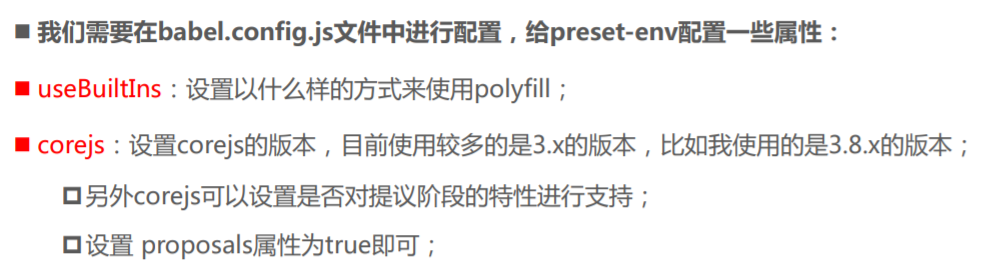
useBuiltIns属性设置
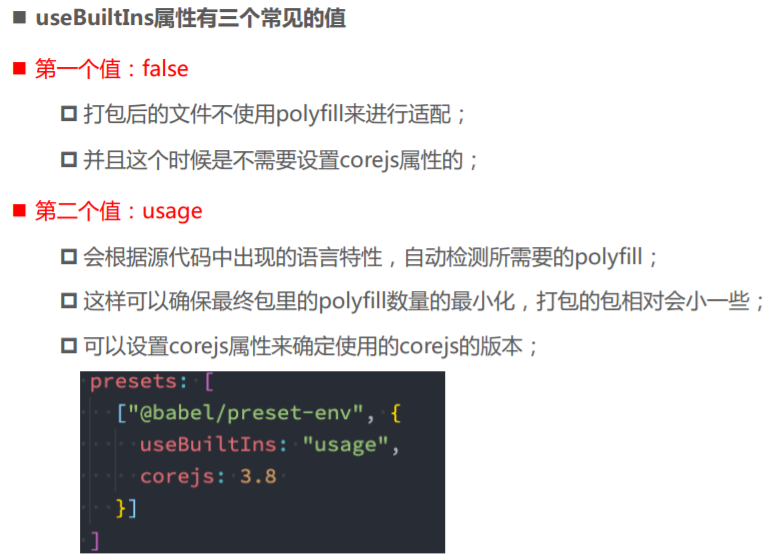
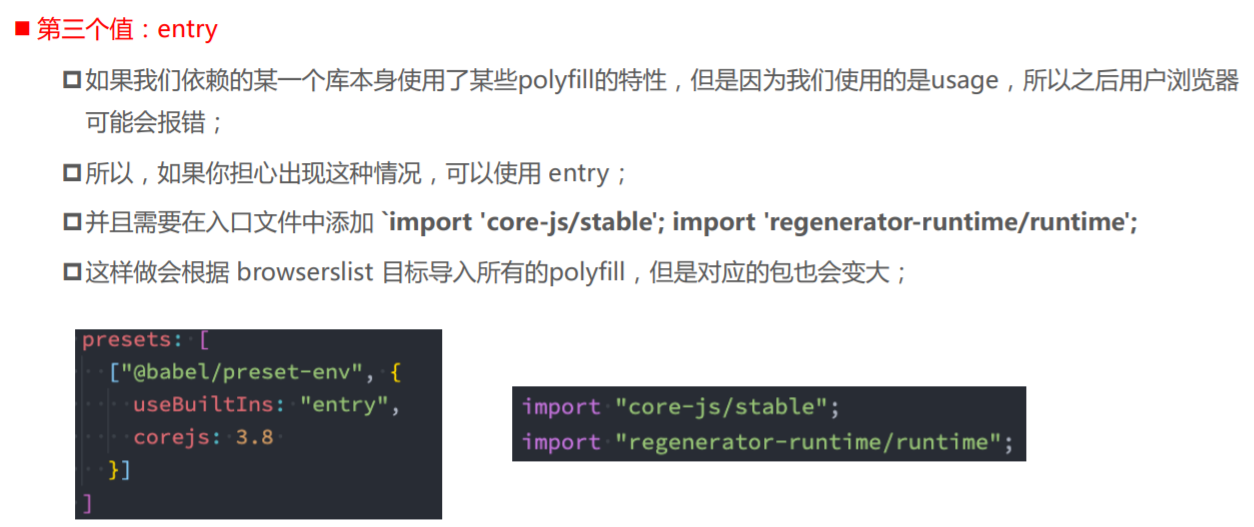
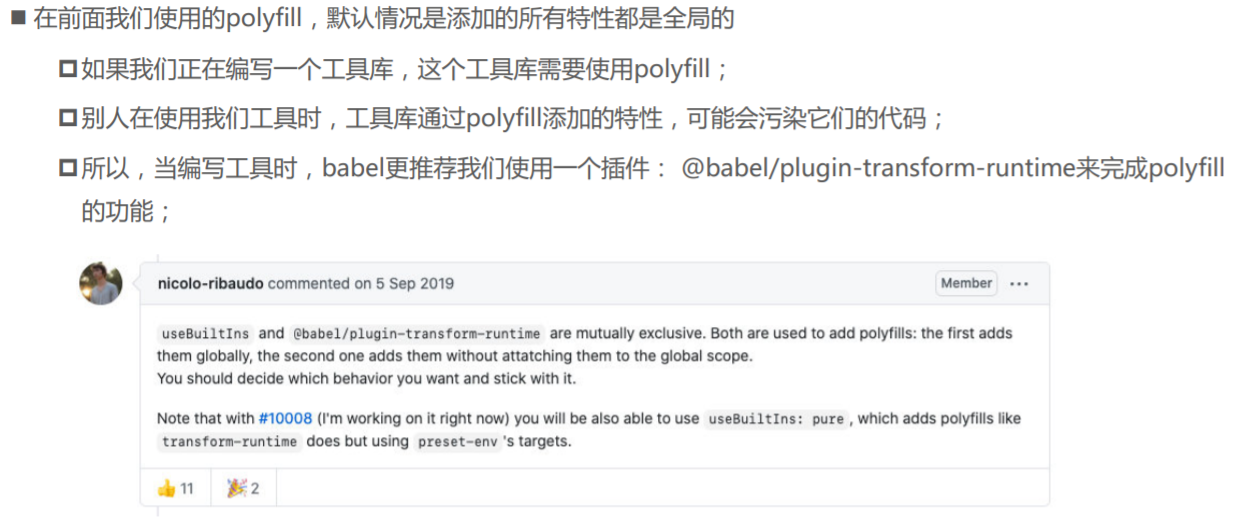
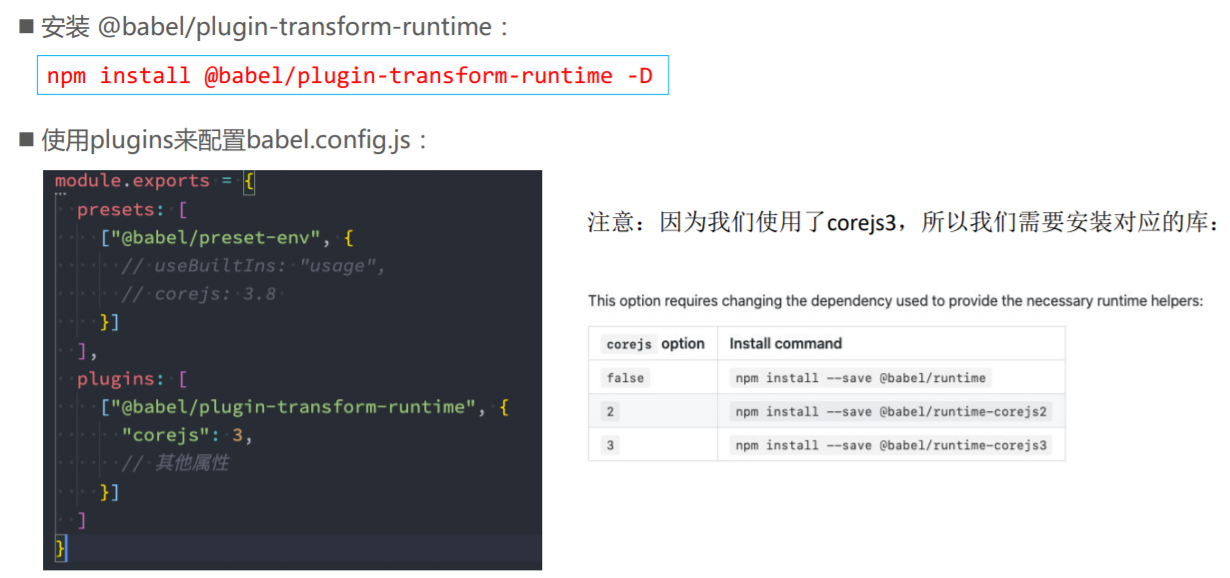
React的jsx支持
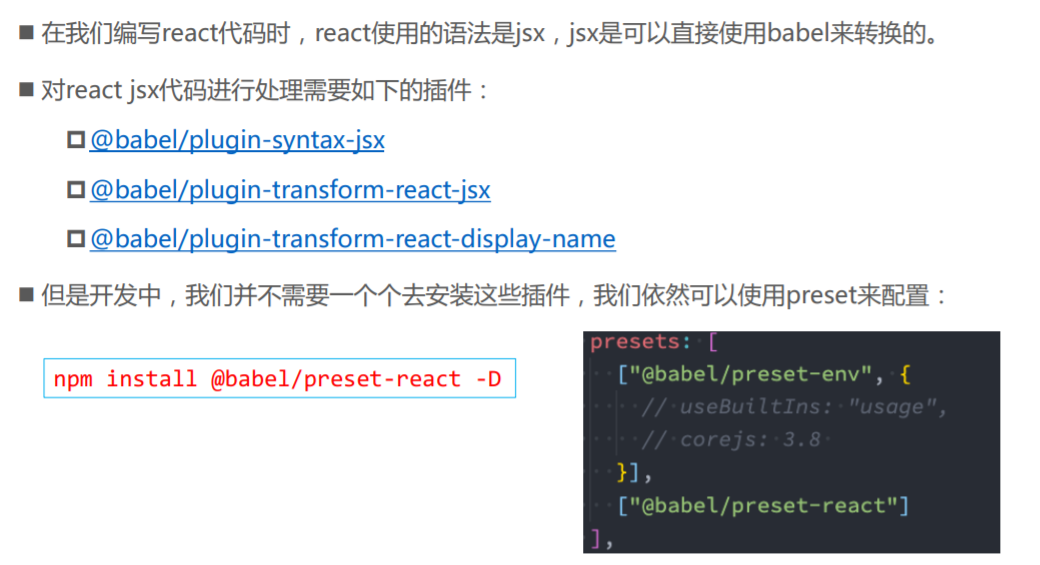
wk.config.js
const path = require('path');
const { CleanWebpackPlugin } = require('clean-webpack-plugin');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
mode: "development",
entry: "./src/react_index.jsx",
devtool: "source-map",
output: {
filename: "js/bundle.js",
// 必须是一个绝对路径
path: path.resolve(__dirname, "./build"),
// assetModuleFilename: "img/[name].[hash:6][ext]"
},
module: {
rules: [
{
test: /.jsx?$/,
exclude: /node_modules/, // 排除node_modules文件夹
use: {
loader: "babel-loader",
// options: {
// // 使用预设,就不是plugins,而是presets
// presets: [
// ["@babel/preset-env", {
// // 不推荐在这里写,推荐在.browserslistrc中统一配置
// // targets: ["chrome 88"]
// // enmodules: true
// }]
// ]
// // 使用指定插件,就是plugins
// // plugins: [
// // "@babel/plugin-transform-arrow-functions",
// // "@babel/plugin-transform-block-scoping"
// // ]
// }
}
}
]
},
plugins: [
new CleanWebpackPlugin(),
new HtmlWebpackPlugin({
title: "哈哈哈 webpack",
template: "./index.html"
})
]
}
babel.config.js
module.exports = {
presets: [
["@babel/preset-env", {
// useBuiltIns:哪些东西需要构建
// false: 不用任何的polyfill相关的代码
// usage: 代码中需要哪些polyfill, 就引用相关的api
// entry: 手动在入口文件中导入 core-js/regenerator-runtime, 根据目标浏览器引入所有对应的polyfill
useBuiltIns: "entry",
corejs: 3 // 【默认用版本2,这里安装的是3版本,不指定就报错。】
}],
["@babel/preset-react"]
],
// 【了解】
// plugins: [
// ["@babel/plugin-transform-runtime", {
// corejs: 3
// }]
// ]
}
index.js
import "core-js/stable";
import "regenerator-runtime/runtime";
const message = "Hello World";
const foo = (info) => {
console.log(info)
}
foo(message);
const p = new Promise((resolve, reject) => {})
TypeScript的编译
【tsc --init:这一步必须做,否则报错。】
【npx tsc:是手动执行编译ts。】
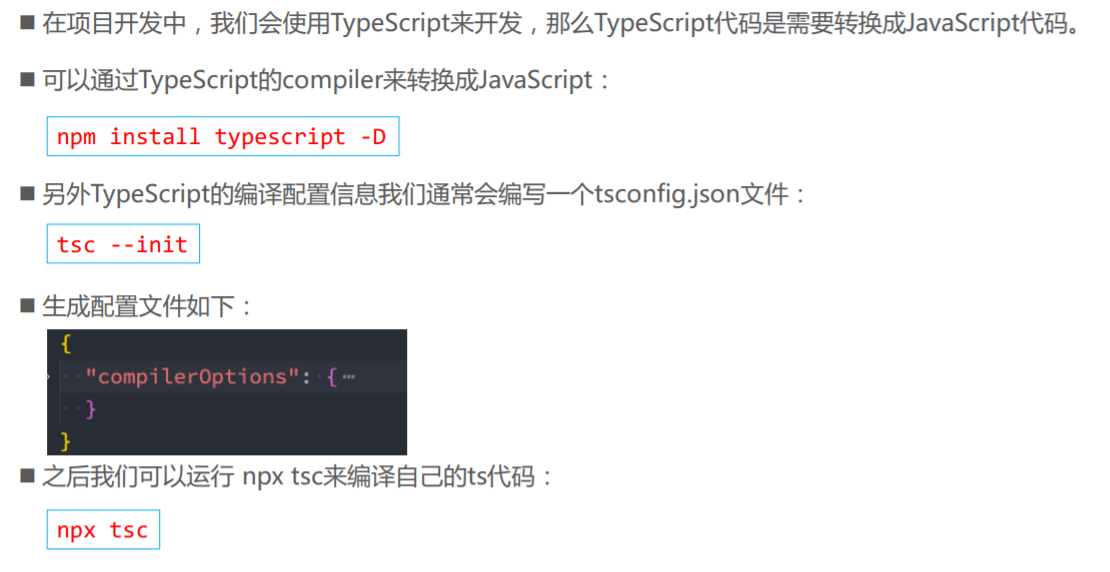
使用ts-loader
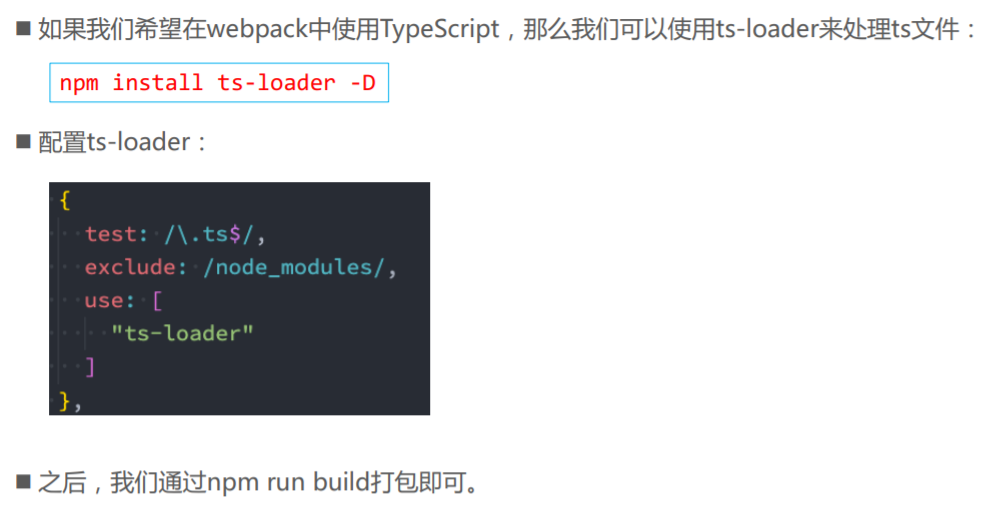
使用babel-loader
- cnpm install @babel/preset-typescript -D
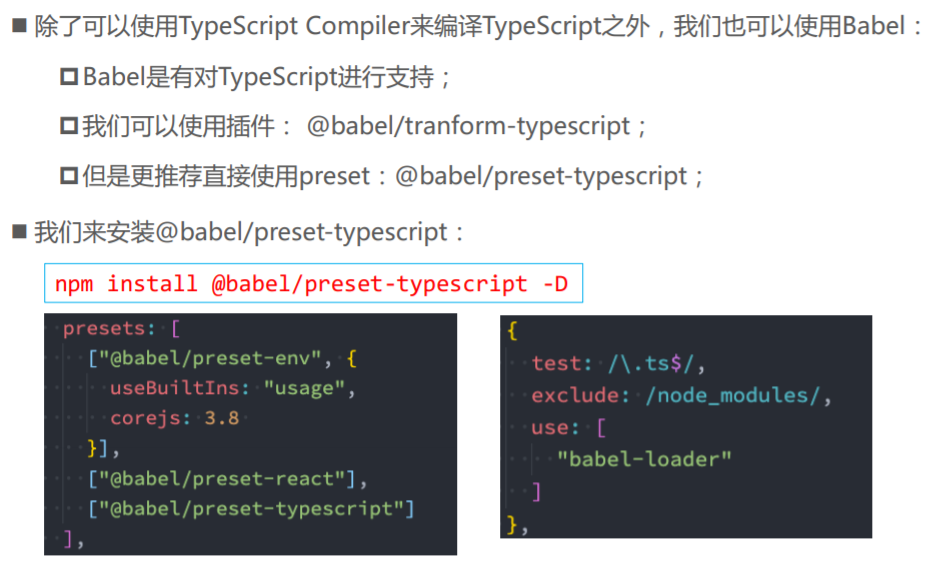
ts-loader和babel-loader选择
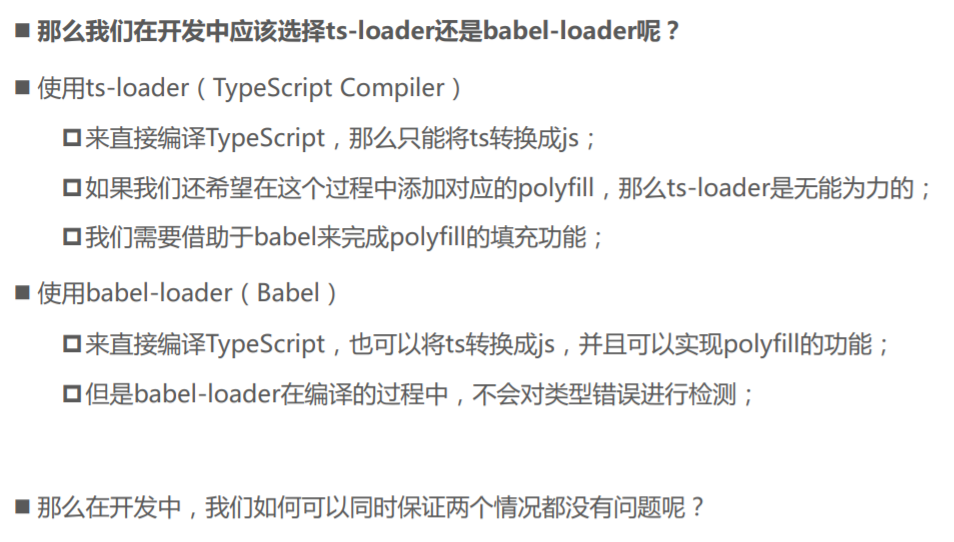
编译TypeScript最佳实践
- 【使用tsc来进行类型的检查后,再使用Babel来完成代码的转换】
"scripts": {
"build": "webpack --config wk.config.js",
"type-check": "tsc --noEmit",
"type-check-watch": "tsc --noEmit --watch"
}
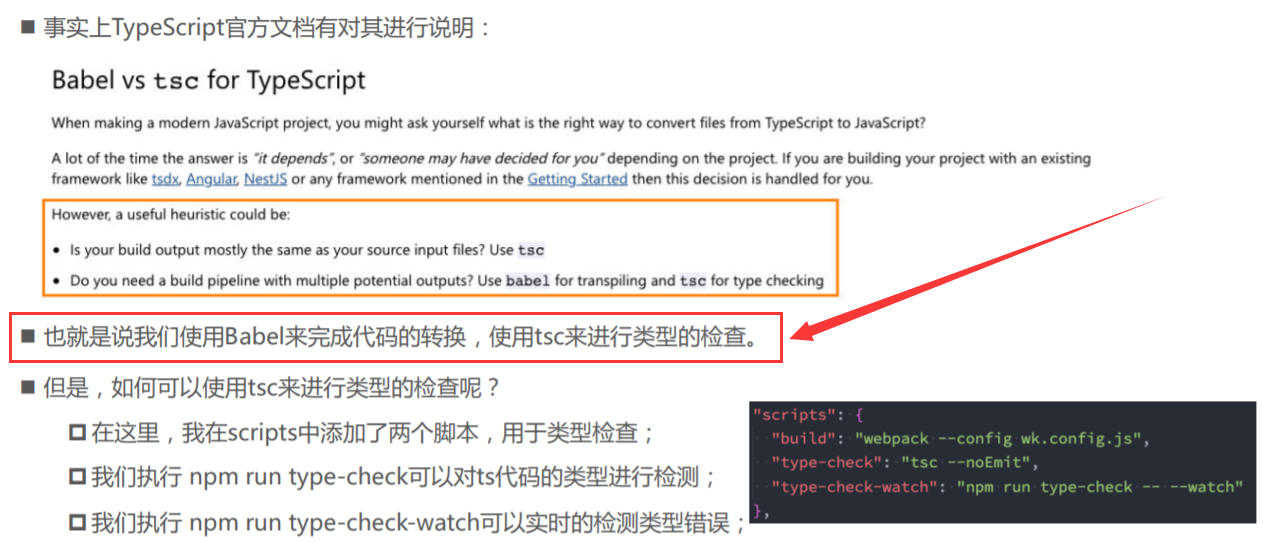
wk.config.js
const path = require('path');
const { CleanWebpackPlugin } = require('clean-webpack-plugin');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
mode: "development",
entry: "./src/index.ts",
devtool: "source-map",
output: {
filename: "js/bundle.js",
// 必须是一个绝对路径
path: path.resolve(__dirname, "./build"),
// assetModuleFilename: "img/[name].[hash:6][ext]"
},
module: {
rules: [
{
test: /.jsx?$/,
exclude: /node_modules/,
use: {
loader: "babel-loader",
// options: {
// presets: [
// ["@babel/preset-env", {
// // targets: ["chrome 88"]
// // enmodules: true
// }]
// ]
// // plugins: [
// // "@babel/plugin-transform-arrow-functions",
// // "@babel/plugin-transform-block-scoping"
// // ]
// }
}
},
{
test: /.ts$/,
exclude: /node_modules/,
// 本质上是依赖于typescript(typescript compiler)
use: "babel-loader"
}
]
},
plugins: [
new CleanWebpackPlugin(),
new HtmlWebpackPlugin({
title: "coderwhy webpack",
template: "./index.html"
})
]
}
babel.config.js
module.exports = {
presets: [
["@babel/preset-env", {
// false: 不用任何的polyfill相关的代码
// usage: 代码中需要哪些polyfill, 就引用相关的api
// entry: 手动在入口文件中导入 core-js/regenerator-runtime, 根据目标浏览器引入所有对应的polyfill
useBuiltIns: "usage",
corejs: 3
}],
["@babel/preset-react"],
["@babel/preset-typescript"]
],
// plugins: [
// ["@babel/plugin-transform-runtime", {
// corejs: 3
// }]
// ]
}
package.json
{
"name": "01_learn_webpack",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"build": "webpack --config wk.config.js",
"type-check": "tsc --noEmit",
"type-check-watch": "tsc --noEmit --watch"
},
"author": "",
"license": "ISC",
"devDependencies": {
"@babel/cli": "^7.12.10",
"@babel/core": "^7.12.10",
"@babel/plugin-transform-arrow-functions": "^7.12.1",
"@babel/plugin-transform-block-scoping": "^7.12.12",
"@babel/plugin-transform-runtime": "^7.12.10",
"@babel/preset-env": "^7.12.11",
"@babel/preset-react": "^7.12.10",
"@babel/preset-typescript": "^7.12.13",
"autoprefixer": "^10.2.3",
"babel-loader": "^8.2.2",
"clean-webpack-plugin": "^3.0.0",
"copy-webpack-plugin": "^7.0.0",
"css-loader": "^5.0.1",
"html-webpack-plugin": "^5.0.0-beta.6",
"less": "^4.1.0",
"less-loader": "^7.3.0",
"postcss": "^8.2.4",
"postcss-cli": "^8.3.1",
"postcss-loader": "^4.1.0",
"postcss-preset-env": "^6.7.0",
"style-loader": "^2.0.0",
"ts-loader": "^8.0.14",
"webpack": "^5.14.0",
"webpack-cli": "^4.3.1"
},
"dependencies": {
"@babel/runtime-corejs3": "^7.12.5",
"core-js": "^3.8.3",
"react": "^17.0.1",
"react-dom": "^17.0.1",
"regenerator-runtime": "^0.13.7"
}
}
index.ts
const message: string = "Hello TypeScript";
const foo = (info: string) => {
console.log(info);
}
foo('111');
// const p = new Promise((resolve, reject) => {})
export {}