Given an m x n
board
of characters and a list of strings words
, return all words on the board.
Each word must be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once in a word.
Example 1:
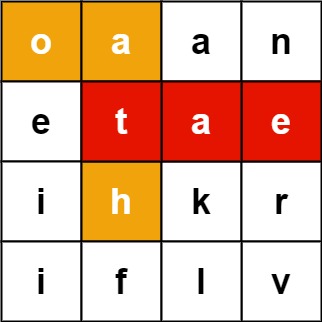
Input: board = [["o","a","a","n"],["e","t","a","e"],["i","h","k","r"],["i","f","l","v"]], words = ["oath","pea","eat","rain"] Output: ["eat","oath"]
Example 2:
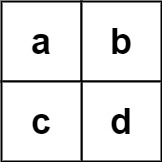
Input: board = [["a","b"],["c","d"]], words = ["abcb"] Output: []
Constraints:
m == board.length
n == board[i].length
1 <= m, n <= 12
board[i][j]
is a lowercase English letter.1 <= words.length <= 3 * 104
1 <= words[i].length <= 10
words[i]
consists of lowercase English letters.- All the strings of
words
are unique.
单词搜索 II。
给定一个 m x n 二维字符网格 board 和一个单词(字符串)列表 words, 返回所有二维网格上的单词 。
单词必须按照字母顺序,通过 相邻的单元格 内的字母构成,其中“相邻”单元格是那些水平相邻或垂直相邻的单元格。同一个单元格内的字母在一个单词中不允许被重复使用。
来源:力扣(LeetCode)
链接:https://leetcode.cn/problems/word-search-ii
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
做这道题之前需要先做79题版本一。79题我们用 backtracking 的方式在矩阵里找一个 word 是否存在。这道题是给你若干个words,请你判断这里面有哪些单词可以在矩阵里被找到。这道题其实也还是可以单纯用 backtracking 的办法做,但是无奈会超时。但是这道题我们可以用字典树对所有的单词做预处理以加快速度。
对于 input 给的每个单词,我们都把他加到字典树里,字典树不熟悉的请先做208和211题。字典树创建好以后,我们就可以做 DFS + backtracking 判断每个单词是否可以在矩阵内被找到。
时间
空间
Java实现
1 class Solution { 2 public List<String> findWords(char[][] board, String[] words) { 3 List<String> res = new ArrayList<>(); 4 int m = board.length; 5 int n = board[0].length; 6 TrieNode root = buildTrie(words); 7 for (int i = 0; i < m; i++) { 8 for (int j = 0; j < n; j++) { 9 dfs(board, i, j, root, res); 10 } 11 } 12 return res; 13 } 14 15 private void dfs(char[][] board, int i, int j, TrieNode node, List<String> res) { 16 char c = board[i][j]; 17 // 如果已经访问过或者字典树node为空,则返回 18 if (c == '#' || node.children[c - 'a'] == null) { 19 return; 20 } 21 node = node.children[c - 'a']; 22 if (node.word != null) { 23 res.add(node.word); 24 node.word = null; 25 } 26 board[i][j] = '#'; // 把访问过的地方标记成# 27 if (i > 0) { 28 dfs(board, i - 1, j, node, res); 29 } 30 if (j > 0) { 31 dfs(board, i, j - 1, node, res); 32 } 33 if (i < board.length - 1) { 34 dfs(board, i + 1, j, node, res); 35 } 36 if (j < board[0].length - 1) { 37 dfs(board, i, j + 1, node, res); 38 } 39 board[i][j] = c; // backtracking 把字母再改回去 40 } 41 42 private TrieNode buildTrie(String[] words) { 43 TrieNode root = new TrieNode(); 44 for (String w : words) { 45 TrieNode node = root; 46 for (int i = 0; i < w.length(); i++) { 47 int j = w.charAt(i) - 'a'; 48 if (node.children[j] == null) { 49 node.children[j] = new TrieNode(); 50 } 51 node = node.children[j]; 52 } 53 node.word = w; 54 } 55 return root; 56 } 57 58 class TrieNode { 59 TrieNode[] children = new TrieNode[26]; 60 String word; 61 } 62 }