Prime Path
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 13905 | Accepted: 7841 |
Description
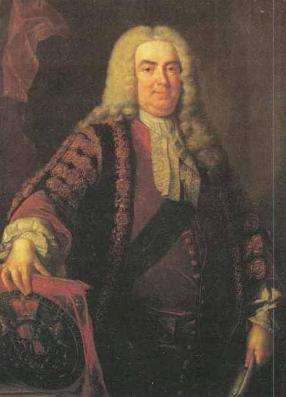
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
1733
3733
3739
3779
8779
8179
Input
One line with a positive number: the number of test cases (at most 100). Then for each test case, one line with two numbers separated by a blank. Both numbers are four-digit primes (without leading zeros).
Output
One line for each case, either with a number stating the minimal cost or containing the word Impossible.
Sample Input
3 1033 8179 1373 8017 1033 1033
Sample Output
6 7 0
这个题一看知道初始状态,知道末状态,准备用双向BFS来做,但是老是WA,也一直苦于找不到WA数据。所以暂时先用普通的单向BFS来做,回头再用双向的做一做。
这个题只需要把可以达到的质数入队就可以了。注意判重。
/************************************************************************* > File Name: Prim_Path_by_bfs.cpp > Author: Zhanghaoran0 > Mail: chiluamnxi@gmail.com > Created Time: 2015年07月29日 星期三 08时37分18秒 ************************************************************************/ #include <iostream> #include <algorithm> #include <cstdio> #include <cstring> #include <math.h> using namespace std; struct node{ int x; int step; }q[10000]; bool flag[10000]; int T; int head, tail; int start, end; bool judge_prim(int x){ if(x == 1 || x == 0) return false; if(x == 2) return true; for(int i = 2; i <= (int)sqrt(x); i ++){ if(x % i == 0) return false; } return true; } int bfs(){ int temp; head = 0; tail = 1; q[head].step = 0; q[head].x = start; memset(flag, 0, sizeof(flag)); while(head < tail){ for(int i = 1; i <= 9; i += 2){ temp = q[head].x / 10 * 10 + i; if(judge_prim(temp) && !flag[temp]){ if(temp == end) return q[head].step + 1; else{ q[tail].x = temp; q[tail ++].step = q[head].step + 1; flag[temp] = true; } } else continue; } for(int i = 0; i <= 9; i ++){ temp = q[head].x /100 * 100 + 10 * i + q[head].x % 10; if(judge_prim(temp) && !flag[temp]){ if(temp == end) return q[head].step + 1; else{ q[tail].x = temp; q[tail ++].step = q[head].step + 1; flag[temp] = true; } } else continue; } for(int i = 0; i <= 9; i ++){ temp = q[head].x / 1000 * 1000 + q[head].x / 10 % 10 * 10 + q[head].x % 10 + i * 100; if(judge_prim(temp) && !flag[temp]){ if(temp == end) return q[head].step + 1; else{ q[tail].x = temp; q[tail ++].step = q[head].step + 1; flag[temp] = true; } } else continue; } for(int i = 1; i <= 9; i ++){ temp = q[head].x % 1000 + i * 1000; if(judge_prim(temp) && !flag[temp]){ if(temp == end) return q[head].step + 1; else{ q[tail].x = temp; q[tail ++].step = q[head].step + 1; flag[temp] = true; } } else continue; } head ++; } return -1; } int main(void){ cin >> T; int steps; while(T --){ cin >> start >> end; if(start == end){ cout << "0" << endl; continue; } steps = bfs(); if(steps != -1){ cout << steps << endl; continue; } else cout << "IMPOSSIBLE" << endl; } return 0; }