最近好忙呀,一直没有完整的时间来安静的编写OracleMembershipProvider,周末领导都去开会了,终于可以偷点时间出来写这个东东了,因为MembershipProvider接口众多,实现起来代码量大,所以下面列出的代码仅仅是截止发帖时部分完成的代码,后续几天还会继续编写,以下代码并未测试,打算所有实现写完后再测试,为了方便大家读代码,我尽量多多添加注释,自定义函数的注释是自己编写的,MembershipProvider的成员注释全部来源于MSDN。
项目里有两个类,OracleMembershipProvider是继承并实现抽象类MembershipProvider并扩充了一些辅助成员,OracleTools是自己定义的一些与Oracle交互的基础成员。

OracleMembershipProvider
1
using System;
2
using System.Collections.Generic;
3
using System.Text;
4
using System.Web;
5
using System.Web.Security;
6
using System.Data;
7
using System.Data.OleDb;
8
using System.Collections;
9
using System.Configuration;
10
11
namespace BoooLee
12

{
13
public class OracleMembershipProvider : MembershipProvider
14
{
15
private OracleTools OT = null;
16
17
/**//*
18
摘要:
19
初始化提供程序。
20
21
参数:
22
config: 名称/值对的集合,表示在配置中为该提供程序指定的、提供程序特定的属性。
23
name: 该提供程序的友好名称。
24
*/
25
public override void Initialize(string name, System.Collections.Specialized.NameValueCollection config)
26
{
27
base.Initialize(name, config);
28
OT = new OracleTools();
29
OT.ConnectionString = config["ConnectionString"];
30
}
31
32
/**//*
33
摘要:
34
使用自定义成员资格提供程序的应用程序的名称。
35
36
返回值:
37
使用自定义成员资格提供程序的应用程序的名称。
38
*/
39
public override string ApplicationName
40
{
41
get
42
{
43
return "OracleMembershipProvider";
44
}
45
set
46
{
47
;
48
}
49
}
50
51
/**//*
52
摘要:
53
处理更新成员资格用户密码的请求。
54
55
参数:
56
newPassword: 指定的用户的新密码。
57
oldPassword: 指定的用户的当前密码。
58
username: 为其更新密码的用户。
59
60
返回值:
61
如果密码更新成功,则为 true;否则为 false。
62
*/
63
public override bool ChangePassword(string username, string oldPassword, string newPassword)
64
{
65
string SqlString = "";
66
67
//更改用户密码
68
if (UserExist(username,oldPassword)>0)
69
{
70
SqlString = string.Format("update sharepointusers set password={0} where username='{1}' and password='{2}'", newPassword, username, oldPassword);
71
return OT.RunSqlNonQuery(SqlString);
72
}
73
74
return false;
75
}
76
77
/**//*
78
摘要:
79
处理更新成员资格用户的密码提示问题和答案的请求。
80
81
参数:
82
newPasswordQuestion: 指定的用户的新密码提示问题。
83
newPasswordAnswer: 指定的用户的新密码提示问题答案。
84
username: 要为其更改密码提示问题和答案的用户。
85
password: 指定的用户的密码。
86
87
返回值:
88
如果成功更新密码提示问题和答案,则为 true;否则,为 false。
89
*/
90
public override bool ChangePasswordQuestionAndAnswer(string username, string password, string newPasswordQuestion, string newPasswordAnswer)
91
{
92
string SqlString = "";
93
94
//更改用户密码问题和密码答案
95
if (UserExist(username,password)>0)
96
{
97
SqlString = string.Format("update sharepointusers set question={0} , answer={1} where username='{2}' and password='{3}'",newPasswordQuestion,newPasswordAnswer,username,password);
98
return OT.RunSqlNonQuery(SqlString);
99
}
100
101
return false;
102
}
103
104
/**//*
105
摘要:
106
将新的成员资格用户添加到数据源。
107
108
参数:
109
isApproved: 是否允许验证新用户。
110
passwordAnswer: 新用户的密码提示问题答案。
111
username: 新用户的用户名。
112
providerUserKey: 成员资格数据源中该用户的唯一标识符。
113
password: 新用户的密码。
114
passwordQuestion: 新用户的密码提示问题。
115
email: 新用户的电子邮件地址。
116
status: 一个 System.Web.Security.MembershipCreateStatus 枚举值,指示是否已成功创建用户。
117
118
返回值:
119
一个用新创建的用户的信息填充的 System.Web.Security.MembershipUser 对象。
120
*/
121
public override MembershipUser CreateUser(string username, string password, string email, string passwordQuestion, string passwordAnswer, bool isApproved, object providerUserKey, out MembershipCreateStatus status)
122
{
123
//TODO 实现创建用户代码
124
throw new Exception("The method or operation is not implemented.");
125
}
126
127
/**//*
128
摘要:
129
从成员资格数据源删除一个用户。
130
131
参数:
132
username: 要删除的用户的名称。
133
deleteAllRelatedData: 如果为 true,则从数据库中删除与该用户相关的数据;如果为 false,则将与该用户相关的数据保留在数据库。
134
135
返回值:
136
如果用户被成功删除,则为 true;否则为 false。
137
*/
138
public override bool DeleteUser(string username, bool deleteAllRelatedData)
139
{
140
string SqlString = "";
141
142
//删除用户
143
if (UserExist(username)>0)
144
{
145
SqlString = string.Format("delete from sharepointusers where username='{0}'", username);
146
return OT.RunSqlNonQuery(SqlString);
147
}
148
149
return false;
150
}
151
152
/**//*
153
摘要:
154
指示成员资格提供程序是否配置为允许用户重置其密码。
155
156
返回值:
157
如果成员资格提供程序支持密码重置,则为 true;否则为 false。默认为 true。
158
*/
159
public override bool EnablePasswordReset
160
{
161
get
162
{
163
return false;
164
}
165
}
166
167
/**//*
168
摘要:
169
指示成员资格提供程序是否配置为允许用户检索其密码。
170
171
返回值:
172
如果成员资格提供程序配置为支持密码检索,则为 true,否则为 false。默认为 false。
173
*/
174
public override bool EnablePasswordRetrieval
175
{
176
get
177
{
178
return false;
179
}
180
}
181
182
/**//*
183
摘要:
184
获取一个成员资格用户的集合,这些用户的电子邮件地址包含要匹配的指定电子邮件地址。
185
186
参数:
187
totalRecords: 匹配用户的总数。
188
pageIndex: 要返回的结果页的索引。pageIndex 是从零开始的。
189
emailToMatch: 要搜索的电子邮件地址。
190
pageSize: 要返回的结果页的大小。
191
192
返回值:
193
包含一页 pageSizeSystem.Web.Security.MembershipUser 对象的 System.Web.Security.MembershipUserCollection 集合,这些对象从 pageIndex 指定的页开始。
194
*/
195
public override MembershipUserCollection FindUsersByEmail(string emailToMatch, int pageIndex, int pageSize, out int totalRecords)
196
{
197
string SqlString = "";
198
MembershipUserCollection muc = new MembershipUserCollection();
199
totalRecords = UserExistByMail(emailToMatch);
200
if (totalRecords>0)
201
{
202
SqlString = string.Format("select * from sharepointusers where email='{0}'", emailToMatch);
203
muc = ToMembershipUserCollection(OT.RunSqlDataTable(SqlString));
204
}
205
return muc;
206
}
207
208
/**//*
209
摘要:
210
获取一个成员资格用户的集合,这些用户的用户名包含要匹配的指定用户名。
211
212
参数:
213
totalRecords: 匹配用户的总数。
214
pageIndex: 要返回的结果页的索引。pageIndex 是从零开始的。
215
usernameToMatch: 要搜索的用户名。
216
pageSize: 要返回的结果页的大小。
217
218
返回值:
219
包含一页 pageSizeSystem.Web.Security.MembershipUser 对象的 System.Web.Security.MembershipUserCollection 集合,这些对象从 pageIndex 指定的页开始。
220
*/
221
public override MembershipUserCollection FindUsersByName(string usernameToMatch, int pageIndex, int pageSize, out int totalRecords)
222
{
223
string SqlString = "";
224
MembershipUserCollection muc = new MembershipUserCollection();
225
totalRecords = UserExist(usernameToMatch);
226
if (totalRecords > 0)
227
{
228
SqlString = string.Format("select * from sharepointusers where username='{0}'", usernameToMatch);
229
muc=ToMembershipUserCollection( OT.RunSqlDataTable(SqlString));
230
}
231
return muc;
232
}
233
234
/**//*
235
摘要:
236
获取数据源中的所有用户的集合,并显示在数据页中。
237
238
参数:
239
totalRecords: 匹配用户的总数。
240
pageIndex: 要返回的结果页的索引。pageIndex 是从零开始的。
241
pageSize: 要返回的结果页的大小。
242
243
返回值:
244
包含一页 pageSizeSystem.Web.Security.MembershipUser 对象的 System.Web.Security.MembershipUserCollection 集合,这些对象从 pageIndex 指定的页开始。
245
*/
246
public override MembershipUserCollection GetAllUsers(int pageIndex, int pageSize, out int totalRecords)
247
{
248
string SqlString = "";
249
MembershipUserCollection muc = new MembershipUserCollection();
250
totalRecords = UsersTotalRecords();
251
if (totalRecords > 0)
252
{
253
SqlString = "select * from sharepointusers";
254
muc = ToMembershipUserCollection(OT.RunSqlDataTable(SqlString));
255
}
256
return muc;
257
}
258
259
public override int GetNumberOfUsersOnline()
260
{
261
throw new Exception("The method or operation is not implemented.");
262
}
263
264
public override string GetPassword(string username, string answer)
265
{
266
throw new Exception("The method or operation is not implemented.");
267
}
268
269
public override MembershipUser GetUser(string username, bool userIsOnline)
270
{
271
throw new Exception("The method or operation is not implemented.");
272
}
273
274
public override MembershipUser GetUser(object providerUserKey, bool userIsOnline)
275
{
276
throw new Exception("The method or operation is not implemented.");
277
}
278
279
public override string GetUserNameByEmail(string email)
280
{
281
throw new Exception("The method or operation is not implemented.");
282
}
283
284
public override int MaxInvalidPasswordAttempts
285
{
286
get
{ throw new Exception("The method or operation is not implemented."); }
287
}
288
289
public override int MinRequiredNonAlphanumericCharacters
290
{
291
get
{ throw new Exception("The method or operation is not implemented."); }
292
}
293
294
public override int MinRequiredPasswordLength
295
{
296
get
{ throw new Exception("The method or operation is not implemented."); }
297
}
298
299
public override int PasswordAttemptWindow
300
{
301
get
{ throw new Exception("The method or operation is not implemented."); }
302
}
303
304
public override MembershipPasswordFormat PasswordFormat
305
{
306
get
{ throw new Exception("The method or operation is not implemented."); }
307
}
308
309
public override string PasswordStrengthRegularExpression
310
{
311
get
{ throw new Exception("The method or operation is not implemented."); }
312
}
313
314
public override bool RequiresQuestionAndAnswer
315
{
316
get
{ throw new Exception("The method or operation is not implemented."); }
317
}
318
319
public override bool RequiresUniqueEmail
320
{
321
get
{ throw new Exception("The method or operation is not implemented."); }
322
}
323
324
public override string ResetPassword(string username, string answer)
325
{
326
throw new Exception("The method or operation is not implemented.");
327
}
328
329
public override bool UnlockUser(string userName)
330
{
331
throw new Exception("The method or operation is not implemented.");
332
}
333
334
public override void UpdateUser(MembershipUser user)
335
{
336
throw new Exception("The method or operation is not implemented.");
337
}
338
339
public override bool ValidateUser(string username, string password)
340
{
341
throw new Exception("The method or operation is not implemented.");
342
}
343
344
非MembershipProvider成员#region 非MembershipProvider成员
345
346
/**//*
347
摘要:
348
判断用户是否存在
349
350
参数:
351
username:用户名
352
353
返回值:
354
找到用户返回true,没有找到用户false
355
*/
356
private int UserExist(string username)
357
{
358
string SqlString = "";
359
string r = "";
360
int Count = 0;
361
362
SqlString = string.Format("select count(*) from sharepointusers where username='{0}'", username);
363
r = OT.RunSqlScalar(SqlString);
364
try
365
{
366
Count = int.Parse(r);
367
}
368
catch (Exception)
369
{
370
Count = 0;
371
}
372
373
return Count;
374
}
375
376
/**//*
377
摘要:
378
判断用户是否存在
379
380
参数:
381
username:用户名
382
password:用户密码
383
384
返回值:
385
找到用户的用户数。
386
*/
387
private int UserExist(string username, string password)
388
{
389
string SqlString = "";
390
string r = "";
391
int Count = 0;
392
393
SqlString = string.Format("select count(*) from sharepointusers where username='{0}' and password='{1}'", username,password);
394
r = OT.RunSqlScalar(SqlString);
395
try
396
{
397
Count = int.Parse(r);
398
}
399
catch (Exception)
400
{
401
Count = 0;
402
}
403
404
return Count;
405
}
406
407
/**//*
408
摘要:
409
判断用户是否存在
410
411
参数:
412
email:用户名
413
414
返回值:
415
找到用户的用户数。
416
*/
417
private int UserExistByMail(string email)
418
{
419
string SqlString = "";
420
string r = "";
421
int Count = 0;
422
423
SqlString = string.Format("select count(*) from sharepointusers where email='{0}'",email);
424
r = OT.RunSqlScalar(SqlString);
425
try
426
{
427
Count = int.Parse(r);
428
}
429
catch (Exception)
430
{
431
Count = 0;
432
}
433
434
return Count;
435
}
436
437
/**//*
438
摘要:
439
转换用户数据表为MembershipUserCollection
440
441
参数:
442
userstable:用户表
443
444
返回值:
445
返回包含用户数据的MembershipUserCollection。
446
*/
447
private MembershipUserCollection ToMembershipUserCollection(DataTable userstable)
448
{
449
MembershipUserCollection muc = new MembershipUserCollection();
450
451
foreach (DataRow dr in userstable.Rows)
452
{
453
MembershipUser mu = new MembershipUser();
454
mu.UserName = dr["username"];
455
mu.Email = dr["email"];
456
mu.PasswordQuestion = dr["question"];
457
458
muc.Add(mu);
459
}
460
461
return muc;
462
}
463
464
private int UsersTotalRecords()
465
{
466
string SqlString = "";
467
string r = "";
468
int Count = 0;
469
470
SqlString = "select count(*) from sharepointusers";
471
r = OT.RunSqlScalar(SqlString);
472
try
473
{
474
Count = int.Parse(r);
475
}
476
catch (Exception)
477
{
478
Count = 0;
479
}
480
481
return Count;
482
}
483
#endregion
484
485
}
486
}
487
using System;
using System.Collections.Generic;
using System.Text;
using System.Data;
using System.Data.OleDb;

namespace BoooLee


{
class OracleTools

{

/**//// <summary>
/// 私有变量
/// </summary>
private string _ConnectionString = ""; //数据库连接串



/**//// <summary>
/// 数据库连接串
/// </summary>
public string ConnectionString

{
get

{
return _ConnectionString;
}

set

{
_ConnectionString=value;
}
}


/**//// <summary>
/// 运行无返回值的Sql语句。
/// </summary>
/// <param name="SqlString">指定要运行的Sql语句。</param>
/// <param name="Conn">指定数据库连接。</param>
/// <returns>成功返回true,失败返回false。</returns>
public bool RunSqlNonQuery(string SqlString)

{
OleDbConnection Conn=null;

try

{
using (OleDbCommand Command = new OleDbCommand())

{
Command.CommandText = SqlString;
Conn = GetConnection();
Conn.Open();
Command.Connection = Conn;
Command.ExecuteNonQuery();
}
return true;
}
catch (Exception)

{
return false;
}
finally

{
//关闭数据库连接
if (Conn != null && Conn.State==ConnectionState.Open)
Conn.Close();
}
}


/**//// <summary>
/// 运行返回一个值的Select语句。
/// </summary>
/// <param name="SqlString">指定要运行的Sql语句。</param>
/// <param name="Conn">指定数据库连接。</param>
/// <returns>成功返回Select语句结果,失败返回空字符串。</returns>
public string RunSqlScalar(string SqlString)

{
OleDbConnection Conn = null;

try

{
Conn = GetConnection();
Conn.Open();

using (OleDbCommand Command = new OleDbCommand())

{
Command.CommandText = SqlString;
Command.Connection = Conn;
return Command.ExecuteScalar().ToString();
}
}
catch (Exception)

{
return "";
}
finally

{
if (Conn != null && Conn.State == ConnectionState.Open)
Conn.Close();
}
}


/**//// <summary>
/// 运行返回DataTable的Sql语句。
/// </summary>
/// <param name="SqlString">指定要运行的Sql语句。</param>
/// <param name="Conn">指定数据库连接。</param>
/// <returns>成功返回Select语句结果DataTable。</returns>
public DataTable RunSqlDataTable(string SqlString)

{
OleDbConnection Conn = null;

try

{
using (OleDbDataAdapter DataAdapter = new OleDbDataAdapter())

{
Conn = GetConnection();
Conn.Open();
OleDbCommand SC = new OleDbCommand();
SC.CommandText = SqlString;
SC.Connection = Conn;
DataAdapter.SelectCommand = SC;
DataTable dt = new DataTable();
DataAdapter.Fill(dt);
return dt;
}
}
catch (Exception)

{
return new DataTable();
}
finally

{
if (Conn != null && Conn.State == ConnectionState.Open)
Conn.Close();
}
}


/**//// <summary>
/// 返回一个OleDbConnection对象。
/// </summary>
/// <returns>成功返回的OleDbConnection对象,失败返回null。</returns>
public OleDbConnection GetConnection()

{
try

{
OleDbConnection result = new OleDbConnection();
result.ConnectionString = _ConnectionString;
return result;
}
catch (Exception)

{
return null;
}
}
}
}

废话时间:
昨天跟伙计去了一个傣家菜馆,虽然经常去,但昨天有些特别,特别到哪里呢,俺终于鼓足勇气点了一盘竹虫,
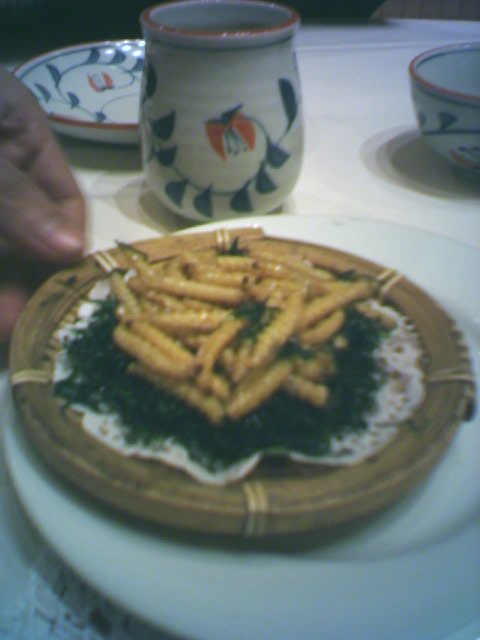
看着蛮XX的,把在座的其他几个伙计看的好难受,俺也有点怕了,吃还是不吃呢???我让服务员先吃一个给我看,没想到女服务还真大方,抓起一个就吃,好象很享受的样子,俺心想,人家女子都吃列,大老爷们还怕啥,吃!!!

哈哈,还真香,有点蚕蛹的味道,都是高蛋白嘛,这么一小碟不便宜呢,除了给老婆硬吃了一条,其他全被我吃光了,边喝小酒边吃虫子,乐哉!乐哉!