一、ToDoList需求
参考链接http://www.todolist.cn/
1.将用户输入添加至待办项
2.可以对todolist进行分类(待办项和已完成组),用户勾选既将待办项分入已完成组
3.todolist的每一项可删除和编辑
4.下方有clear按钮,并清空所有todolist项
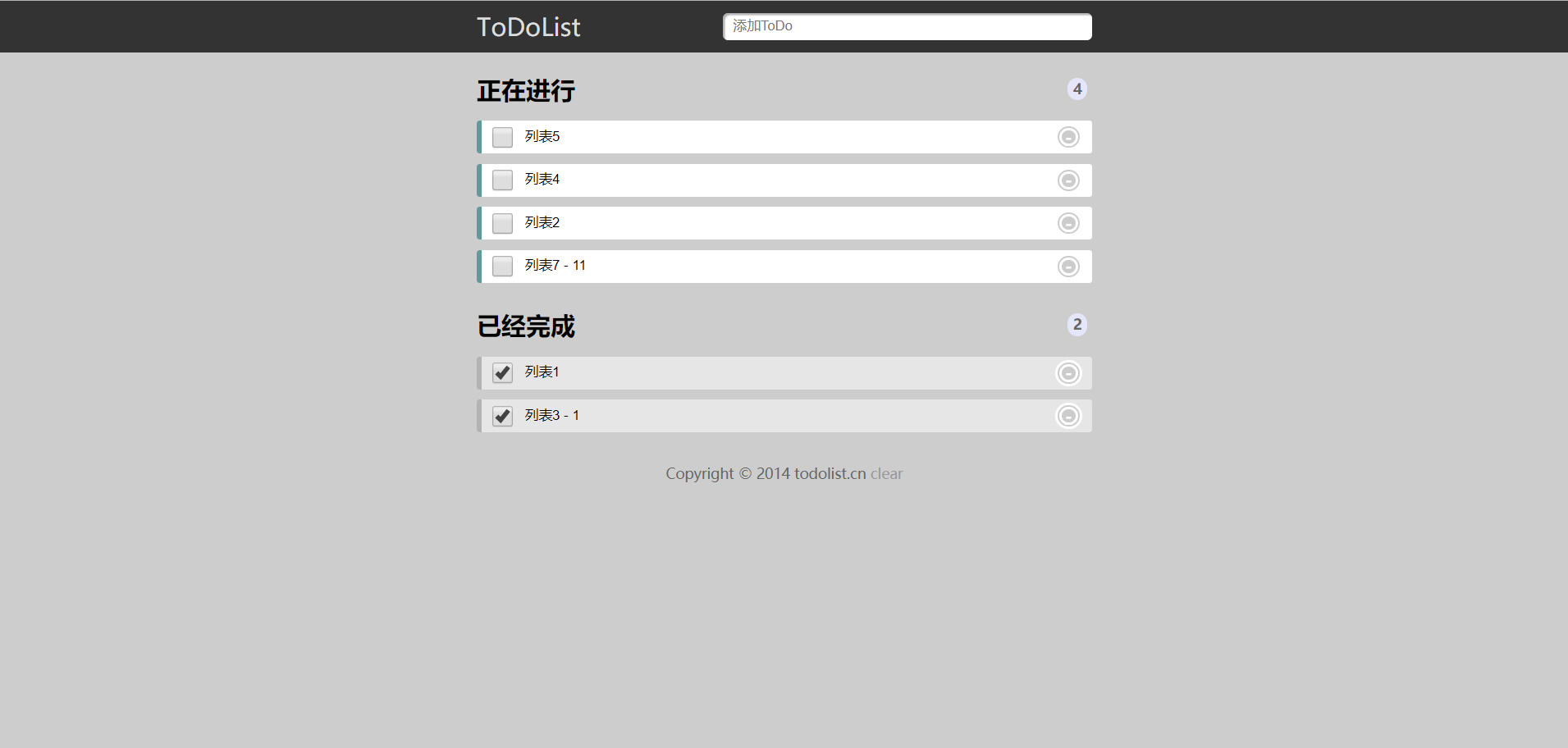
二、html
<!DOCTYPE html>
<html lang="en">
<head>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no">
<title>ToDoList-最简单的待办事项列表</title>
<meta name="description" content="ToDoList无须注册即可使用,数据存储在用户浏览器的html5本地数据库里,是最简单最安全的待办事项列表应用!">
<link rel="stylesheet" href="./css/index.css">
</head>
<body>
<div class="wrap">
<!--顶部-->
<div class="header">
<form action="javascript:postAction()" id="form">
<label for="title">ToDoList</label>
<input type="text" id="title" name="title" placeholder="添加ToDo" required = "required" autocomplete="off">
</form>
</div>
<!--内容区域-->
<div class="content">
<h2>
正在进行
<span id="todocount">0</span>
</h2>
<ul id="todolist"></ul>
<h2>
已经完成
<span id="donecount">0</span>
</h2>
<ul id="donelist"></ul>
</div>
<!--底部-->
<div class="footer">
<span>Copyright © 2014 todolist.cn </span>
<a href="javascript:clear();">clear</a>
</div>
</div>
<script type="text/javascript" src="./js/index.js"></script>
</body>
</html>
三、css
*{ padding: 0; margin: 0;}
body{ background-color: #cdcdcd;}
.header{ background-color:#333; height: 50px; line-height: 50px; }
#form{ width: 600px;margin: 0 auto; overflow: hidden;}
#form label{color: #ddd;font-size: 24px;float: left; cursor: pointer;}
#form input{ float: right; width: 60%;height: 26px;text-indent: 10px; border-radius: 5px; border: none;
margin-top: 12px; outline: none; box-shadow: 2px 2px 0.8px rgba(0,0,0,0.3) inset;}
.content{ width: 600px; margin: 20px auto;}
h2{ position: relative; margin: 15px 0;}
h2 span{ position: absolute; right: 5px; display: inline-block; background-color: #e6e6fa; font-size: 14px;
color: #666;text-align: center;height: 22px; line-height: 22px; top: 5px; padding: 0 5px;border-radius: 20px;}
ul{ list-style: none; overflow: hidden;}
ul li{ overflow: hidden; height: 32px; line-height: 32px; background-color: #fff; border-radius: 3px;
border-left: 5px solid #629a9c; padding: 0 10px; margin-bottom: 10px;}
ul li input[type='checkbox']{ float: left; width: 22px; height: 22px; margin: 6px 5px 0 0;}
ul li input[type='text']{ float: left; width: 80%; text-indent: 5px; height: 26px; line-height: 27px; margin: 2px 0;
outline: none; border: none;}
ul li a{ float: right; width:14px;background-color: #ccc; color: white;border: 6px double #fff;margin-top: 3px;
border-radius: 14px; text-align: center;font-weight: bold; cursor: pointer; line-height: 14px;}
#donelist li{ background-color: #e6e6e6; border-left: 5px solid #b3b3b3;}
#donelist li input[type='text']{ background-color: #e6e6e6;}
.footer{ width: 600px; margin: 0 auto; color: #666; font-size: 14px; text-align: center; margin-top: 20px;}
.footer a{ color: #999; text-decoration: none;}
四、js
var todoC = 0;
var doneC = 0;
var todolist = document.getElementById('todolist');
var donelist = document.getElementById('donelist');
var todoCount = document.getElementById('todocount');
var doneCount = document.getElementById('donecount');
// 添加ToDo
function postAction() {
var title = document.getElementById('title');
if(title.value === ""){
alert('内容不能为空!')
}else{
var li = document.createElement('li');
li.innerHTML = '<input type="checkbox" onchange="update();"><input class="title" type="text" onchange="change();" onclick="edit();"><a href="javascript:remove();">-</a>';
if(todoC === 0){ // 第一次添加元素 appendChild
todolist.appendChild(li);
}else{
todolist.insertBefore(li,todolist.children[0]);
}
var txtTitle = document.getElementsByClassName('title')[0];
txtTitle.value = title.value;
loop('todolist');
todoC++;
todoCount.innerText = todoC;
title.value = "";
}
}
//循环 每次添加不同的 i 值
function loop(str) {
var list = null;
str==='todolist' ? list = todolist : list = donelist;
childs = list.childNodes;
for(var i=0; i<childs.length; i++){
childs[i].children[0].setAttribute('onchange','update("'+i+'","'+str+'")');
childs[i].children[1].setAttribute('onclick','edit("'+i+'","'+str+'")');
childs[i].children[1].setAttribute('onchange','change("'+i+'","'+str+'","'+childs[i].children[1].value+'")');
childs[i].children[2].setAttribute('href','javascript:remove("'+i+'","'+str+'")');
}
}
//update 方法
function update(n,str) {
var list = null;
str==='todolist' ? list = todolist : list = donelist;
var li = null;
childs = list.childNodes;
for(var i=0; i<childs.length; i++){
if(i===Number(n)){
li = childs[i];
}
}
remove(n,str); // 删除原有的,得到li 并刷新了原有的li
if(str==='todolist'){
if(doneC === 0){ // 第一次添加元素 appendChild
donelist.appendChild(li);
}else{
donelist.insertBefore(li,donelist.children[0]);
}
loop('donelist');
doneC++;
doneCount.innerText = doneC;
}else if(str === 'donelist'){
todolist.appendChild(li);
loop('todolist');
todoC++;
todoCount.innerText = todoC;
}
}
// edit 方法 编辑title
function edit(n,str) {
var list = null;
str==='todolist' ? list = todolist : list = donelist;
childs = list.childNodes;
for(var i=0; i<childs.length; i++){
if(i===Number(n)){
childs[i].children[1].style.border = '1px solid #ccc';
}
}
}
// change 方法 失去焦点
function change(n,str,oldValue) {
var list = null;
str==='todolist' ? list = todolist : list = donelist;
childs = list.childNodes;
for(var i=0; i<childs.length; i++){
if(i===Number(n)){
childs[i].children[1].style.border = 'none';
if(childs[i].children[1].value === ""){
alert('内容不能为空');
childs[i].children[1].value = oldValue;
}
}
}
loop(str);
}
//清除单个列表
function remove(n,str) {
var list = null;
if(str==='todolist'){
list = todolist;
todoC--;
todoCount.innerText = todoC;
}else if(str==='donelist'){
list = donelist;
doneC--;
doneCount.innerText = doneC;
}
childs = list.childNodes;
for(var i=childs.length-1; i>=0; i--){
if(i===Number(n)){
list.removeChild(childs[n]);
}
}
loop(str);
}
// 清除所有列表
function clear() {
childs1 = todolist.childNodes;
for(var i= childs1.length-1 ; i >= 0 ; i--){
todolist.removeChild(childs1[i]);
}
childs2 = donelist.childNodes;
for(var j = childs2.length-1 ; j >= 0 ; j-- ){
donelist.removeChild(childs2[j]);
}
todoC = 0;
doneC = 0;
todoCount.innerText = todoC;
doneCount.innerText = doneC;
}