环境: win10(10.0.16299.0)+ VS2017
sdk版本:ArcFace v2.0
OPENCV3.43版本
x64平台Debug、Release配置都已通过编译
下载地址:https://download.csdn.net/download/cngwj/10763108
配置过程
->0x01 下载sdk:
虹安sdk https://ai.arcsoft.com.cn
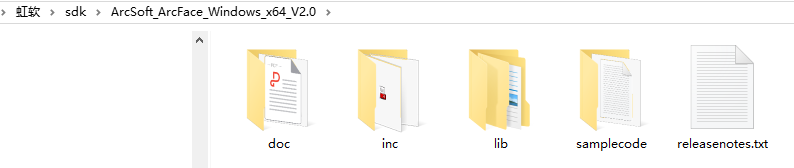
->0x02 工程配置:
1、 添加工程的头文件目录:
a) 右键单击工程名, 选择属性---配置属性---c/c++---常规---附加包含目录
b) 添加头文件存放目录
2、 添加文件引用的 lib 静态库路径:
a) 右键单击工程名,选择属性---配置属性---链接器---常规---附加库目录
b) 添加 lib 文件存放
3、 添加工程引用的 lib 库:
a) 右键单击工程名,选择属性---配置属性---链接器---输入---附加依赖项
b) 添加依赖的 lib 库名称
4、自定义可执行文件输出目录
5、 添加工程引用的 dll 动态库:
a) 把引用的 dll 放到工程的可执行文件所在的目录下(复制到Build目录)
6、添加自己申请的APPID
>0x03 参考代码
/************************************************************************ * Copyright(c) 2018 * All rights reserved. * File: samplecode.cpp * Brief: Powered by ArcSoft 环境: win10(10.0.16299.0)+ VS2017 sdk版本:ArcFace v2.0 x64平台Debug、Release配置都已通过编译 * Version: 0.1 * Author: 一念无明 * Email: cngwj@outlook.com * Date: 2018.11.3 * History: 2018.11.3 建立项目 ************************************************************************/ #include "pch.h" #include "arcsoft_face_sdk.h"//接口文件 #include "amcomdef.h"//平台文件 #include "asvloffscreen.h"//平台文件 #include "merror.h"//错误码文件 #include <direct.h> //目录操作 #include <iostream> #include <stdarg.h> #include <string> #include <opencv.hpp> using namespace std; using namespace cv; #pragma comment(lib, "libarcsoft_face_engine.lib") #define APPID "" #define SDKKey "" #define MERR_ASF_BASE_ALREADY_ACTIVATED 90114 //SDK已激活 #define SafeFree(p) { if ((p)) free(p); (p) = NULL; } #define SafeArrayDelete(p) { if ((p)) delete [] (p); (p) = NULL; } #define SafeDelete(p) { if ((p)) delete (p); (p) = NULL; } int main() { //激活SDK MRESULT res = ASFActivation(APPID, SDKKey); if (MOK != res && MERR_ASF_BASE_ALREADY_ACTIVATED != res) printf("ALActivation fail: %d ", res); else printf("ALActivation sucess: %d ", res); //初始化引擎 MHandle handle = NULL; MInt32 mask = ASF_FACE_DETECT | ASF_FACERECOGNITION | ASF_AGE | ASF_GENDER | ASF_FACE3DANGLE; res = ASFInitEngine(ASF_DETECT_MODE_IMAGE, ASF_OP_0_ONLY, 16, 5, mask, &handle); if (res != MOK) printf("ALInitEngine fail: %d ", res); else printf("ALInitEngine sucess: %d ", res); // 人脸检测 IplImage* img = cvLoadImage("../Build\1.bmp");//图片宽度需符合4的倍数 IplImage* img1 = cvLoadImage("../Build\2.bmp"); if (img && img1) { ASF_MultiFaceInfo detectedFaces1 = { 0 };//多人脸信息; ASF_SingleFaceInfo SingleDetectedFaces1 = { 0 }; ASF_FaceFeature feature1 = { 0 }; ASF_FaceFeature copyfeature1 = { 0 }; res = ASFDetectFaces(handle, img->width, img->height, ASVL_PAF_RGB24_B8G8R8, (MUInt8*)img->imageData, &detectedFaces1); if (MOK == res) { SingleDetectedFaces1.faceRect.left = detectedFaces1.faceRect[0].left; SingleDetectedFaces1.faceRect.top = detectedFaces1.faceRect[0].top; SingleDetectedFaces1.faceRect.right = detectedFaces1.faceRect[0].right; SingleDetectedFaces1.faceRect.bottom = detectedFaces1.faceRect[0].bottom; SingleDetectedFaces1.faceOrient = detectedFaces1.faceOrient[0]; //单人脸特征提取 res = ASFFaceFeatureExtract(handle, img->width, img->height, ASVL_PAF_RGB24_B8G8R8, (MUInt8*)img->imageData, &SingleDetectedFaces1, &feature1); if (res == MOK) { //拷贝feature copyfeature1.featureSize = feature1.featureSize; copyfeature1.feature = (MByte *)malloc(feature1.featureSize); memset(copyfeature1.feature, 0, feature1.featureSize); memcpy(copyfeature1.feature, feature1.feature, feature1.featureSize); } else printf("ASFFaceFeatureExtract 1 fail: %d ", res); } else printf("ASFDetectFaces 1 fail: %d ", res); //第二张人脸提取特征 ASF_MultiFaceInfo detectedFaces2 = { 0 }; ASF_SingleFaceInfo SingleDetectedFaces2 = { 0 }; ASF_FaceFeature feature2 = { 0 }; res = ASFDetectFaces(handle, img1->width, img1->height, ASVL_PAF_RGB24_B8G8R8, (MUInt8*)img1->imageData, &detectedFaces2); if (MOK == res) { SingleDetectedFaces2.faceRect.left = detectedFaces2.faceRect[0].left; SingleDetectedFaces2.faceRect.top = detectedFaces2.faceRect[0].top; SingleDetectedFaces2.faceRect.right = detectedFaces2.faceRect[0].right; SingleDetectedFaces2.faceRect.bottom = detectedFaces2.faceRect[0].bottom; SingleDetectedFaces2.faceOrient = detectedFaces2.faceOrient[0]; res = ASFFaceFeatureExtract(handle, img1->width, img1->height, ASVL_PAF_RGB24_B8G8R8, (MUInt8*)img1->imageData, &SingleDetectedFaces2, &feature2); if (MOK != res) printf("ASFFaceFeatureExtract 2 fail: %d ", res); } else printf("ASFDetectFaces 2 fail: %d ", res); // 单人脸特征比对 MFloat confidenceLevel; res = ASFFaceFeatureCompare(handle, ©feature1, &feature2, &confidenceLevel); if (res != MOK) printf("ASFFaceFeatureCompare fail: %d ", res); else printf("ASFFaceFeatureCompare sucess: %lf ", confidenceLevel); // 人脸信息检测 MInt32 processMask = ASF_AGE | ASF_GENDER | ASF_FACE3DANGLE; res = ASFProcess(handle, img1->width, img1->height, ASVL_PAF_RGB24_B8G8R8, (MUInt8*)img1->imageData, &detectedFaces1, processMask); if (res != MOK) printf("ASFProcess fail: %d ", res); else printf("ASFProcess sucess: %d ", res); // 获取年龄 ASF_AgeInfo ageInfo = { 0 }; res = ASFGetAge(handle, &ageInfo); //printf("年龄: %d ", ageInfo); if (res != MOK) printf("ASFGetAge fail: %d ", res); else printf("ASFGetAge sucess: %d ", res); // 获取性别 ASF_GenderInfo genderInfo = { 0 }; res = ASFGetGender(handle, &genderInfo); if (res != MOK) printf("ASFGetGender fail: %d ", res); else printf("ASFGetGender sucess: %d ", res); // 获取3D角度 ASF_Face3DAngle angleInfo = { 0 }; res = ASFGetFace3DAngle(handle, &angleInfo); if (res != MOK) printf("ASFGetFace3DAngle fail: %d ", res); else printf("ASFGetFace3DAngle sucess: %d ", res); SafeFree(copyfeature1.feature); //释放内存 } //获取版本信息 const ASF_VERSION* pVersionInfo = ASFGetVersion(handle); printf("版本号: %s ", pVersionInfo->Version); //反初始化 res = ASFUninitEngine(handle); if (res != MOK) printf("ALUninitEngine fail: %d ", res); else printf("ALUninitEngine sucess: %d ", res); getchar(); return 0; }
用其它照片测试需要注意图片的宽度
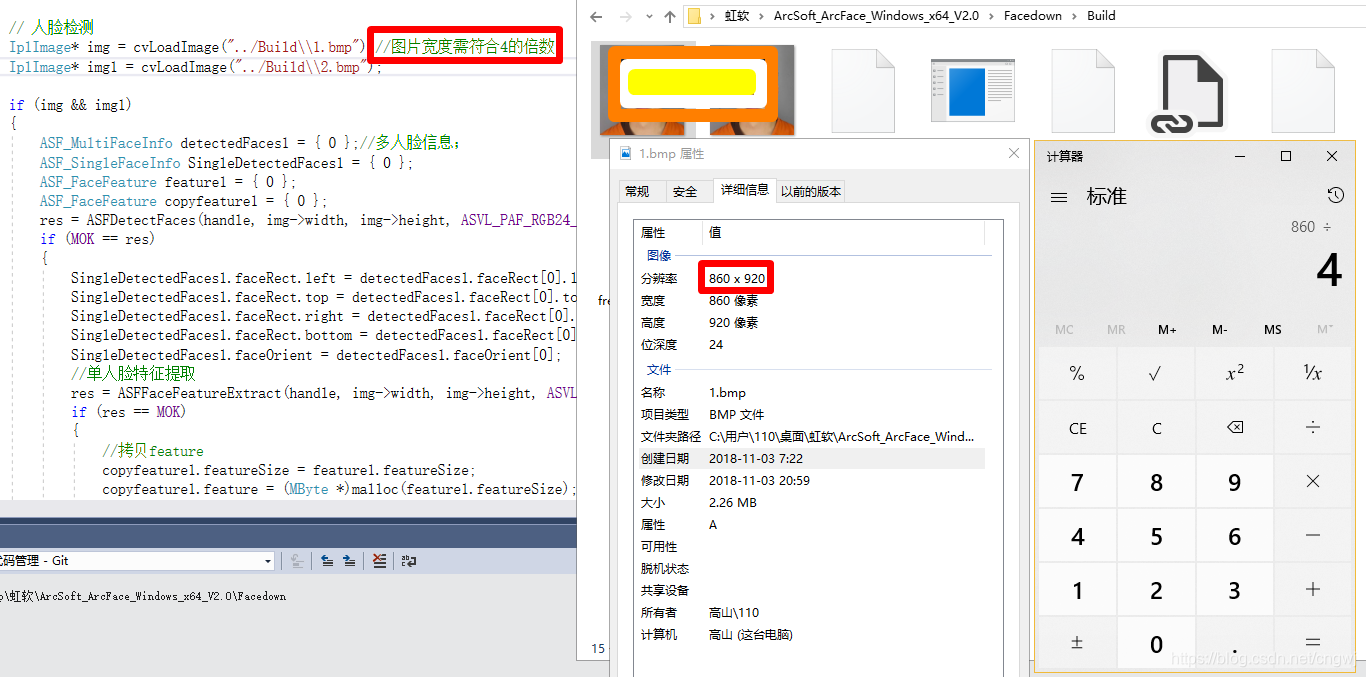