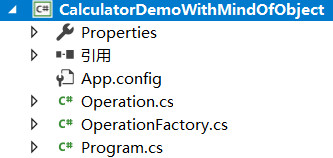
using System;
namespace CalculatorDemoWithMindOfObject
{
public class Operation
{
public double NumberA { get; set; }
public double NumberB { get; set; }
public virtual double GetResult()
{
double result = 0;
return result;
}
}
public class OperationAdd : Operation
{
public override double GetResult()
{
double result = 0;
result = NumberA + NumberB;
return result;
}
}
public class OperationSub : Operation
{
public override double GetResult()
{
double result = 0;
result = NumberA - NumberB;
return result;
}
}
public class OperationMul : Operation
{
public override double GetResult()
{
double result = 0;
result = NumberA * NumberB;
return result;
}
}
public class OperationDiv : Operation
{
public override double GetResult()
{
if (NumberB == 0)
{
throw new Exception("除数不能为0");
}
double result = 0;
result = NumberA / NumberB;
return result;
}
}
}
namespace CalculatorDemoWithMindOfObject
{
/// <summary>
/// 运算类工厂(简单工厂模式)
/// </summary>
public class OperationFactory
{
public static Operation CreateOperation(string operatorInfo)
{
Operation operation = null;
switch (operatorInfo)
{
case "+":
operation = new OperationAdd();
break;
case "-":
operation = new OperationSub();
break;
case "*":
operation = new OperationMul();
break;
case "/":
operation = new OperationDiv();
break;
}
return operation;
}
}
}
using System;
namespace CalculatorDemoWithMindOfObject
{
/// <summary>
/// 使用面向对象三大特征(封装、继承、多态)实现的计算器
/// LDH @ 2019-2-12
/// </summary>
class Program
{
static void Main()
{
Console.Title = "使用面向对象三大特征,实现简单的计算器功能";
Operation op = OperationFactory.CreateOperation("+");
op.NumberA = 10;
op.NumberB = 20;
double result = op.GetResult();
Console.WriteLine("运算结果:" + result);
Console.ReadKey();
}
}
}
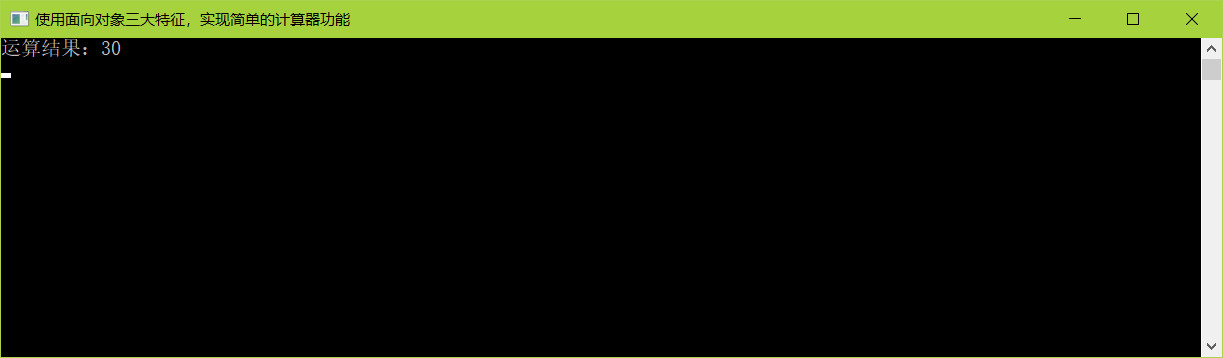