#include <stdio.h> #include <iostream> #include <malloc.h> #define Max 10 using namespace std; typedef struct{ char data[Max]; int length; }sqlist; void initlist(sqlist *&l) //线性表初始化*&代表可以对l的地址改变 { l=(sqlist *)malloc(sizeof(sqlist)); l->length=0; } void easyinsert(sqlist *l) { char c=getchar(); if(l->length>Max) { return ; } l->data[l->length++]=c; } void display(sqlist l) { for(int i=0;i<l.length;i++) { cout<<l.data[i]; } cout<<endl; } int leng(sqlist l) { cout<<l.length<<endl; return l.length; } void getelem(sqlist l,int n) { if(n<1||n>l.length) { return ; } char e; e=l.data[n-1]; cout<<e<<endl; } void weizhi(sqlist l,char c) { for(int i=0;i<l.length;i++) { if(c==l.data[i]) { cout<<i+1<<" "; } } } void charu(sqlist *l,char c,int n) { if(n<1||n>l->length) { return ; } l->length++; int i; for(i=l->length;i>n;i--) { l->data[i-1]=l->data[i-2]; } l->data[i-1]=c; } void shanchu(sqlist *l,int i) { if(i<1||i>l->length) { return ; } l->length--; for(int j=i-1;j<l->length;j++) { l->data[j]=l->data[j+1]; } } int main() { sqlist *l; initlist(l); easyinsert(l); easyinsert(l); easyinsert(l); easyinsert(l); easyinsert(l); display(*l); leng(*l); getelem(*l,4); weizhi(*l,'c'); charu(l,'g',5); display(*l); shanchu(l,3); display(*l); }
初始化必须用*&l,其余如果对于线性表有改变则调用指针
原因:https://www.cnblogs.com/xiang-little/p/5840809.html
void initlist(sqlist *&l) //线性表初始化*&代表可以对l的地址改变
{
l=(sqlist *)malloc(sizeof(sqlist));
l->length=0;
}
链式存储结构:
union的知识点,慕课数据结构 第二讲2.1第六个视频
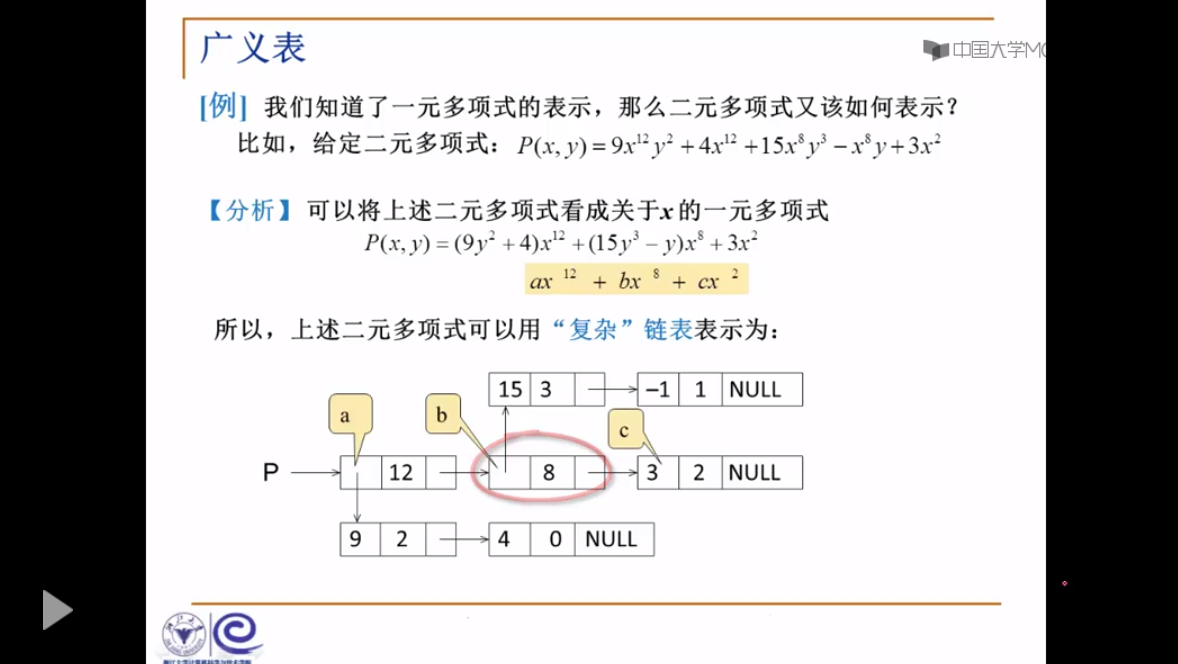
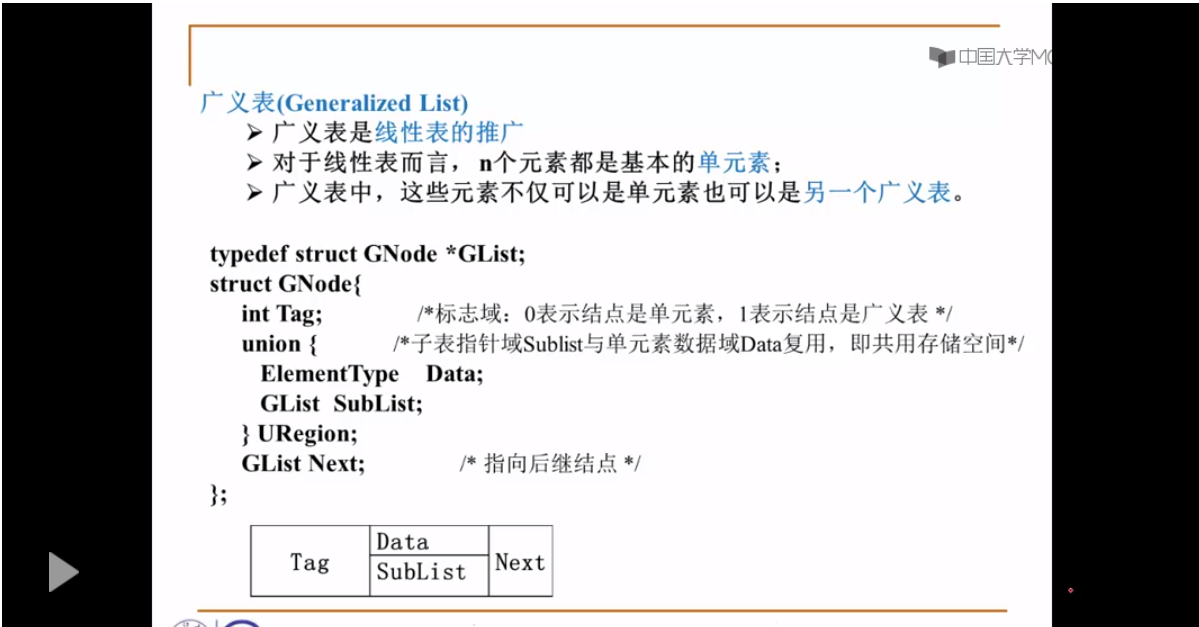
链式结构基础(建立两个链表,连接)
这样//的部分需要注意,别的很简单。
#include <stdio.h> #include <iostream> #include <malloc.h> #define Max 10 using namespace std; typedef struct node{ int data; struct node *next; //因为此处要使用自己所以 //第一行一定要加上node这个名字; }node;//这里也可以写成node,*linklist这样的话 //node *==linklist; void creat(node *&l,int m) { l=new node; //依然很重要分配空间也可malloc
//l=(node *)malloc(sizeof(node)) l->next=NULL; node *r; r=l; for(int i=0;i<m;i++) { node *p; p=new node; ///这一行很重要一定要有 cin>>p->data; p->next=NULL; r->next=p; r=p; } } void display(node *l) { node *p; // p->next=NULL; p=l->next; while(p) { cout<<p->data<<" "; p=p->next; } cout<<endl; } void lianjie(node *l,node *m,node *n) { node *p; p=new node; p=m->next; node *q; q=new node; q=l->next; node *r; r=new node; r=n; while(p) { r->next=p; r=r->next; p=p->next; } while(q) { r->next=q; r=r->next; q=q->next; } } int main() { int n; cin>>n; node *l1; creat(l1,n); display(l1); int m; cin>>m; node *l2; creat(l2,m); display(l2); node *l3; creat(l3,0); lianjie(l1,l2,l3); display(l3); }