- 传送门 -
https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem&problem=183
# 247 - Calling Circles
Time limit: 3.000 seconds
| [Root](https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&category=0) | [](https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=submit_problem&problemid=183&category=) [](https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=problem_stats&problemid=183&category=)
[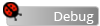](https://www.udebug.com/UVa/247) [](https://uva.onlinejudge.org/external/2/247.pdf) |
###(题面见pdf)
### - 题意 -
给出n, m, 表示 n 个人打了 m 个单向电话, 若两个人互通了电话(直接或间接), 就称他们在一个电话圈里, 问每个电话圈有那些人.
### - 思路 -
floyd: G[i][j]表示 i 向 j 连线, 过一遍 floyd 可以使间接通话变为直接(G[i][j] = G[i][j] || (G[i][k] && G[k][j])), 然后 dfs 查找和一个人互相直接通话的人(G[x][y]&&G[y][x]), 则他们在一个电话圈里.
tarjan: 其实这题我第一反应是强连通分量...强连通分量即是"电话圈".
细节见代码.
### - 代码 -
floyd:
```c++
#include
#include