本文转载自:http://blog.csdn.net/luoshengyang/article/details/6580267
我们在Android系统增加硬件服务的目的是为了让应用层的APP能够通过Java接口来访问硬件服务。那么, APP如何通过Java接口来访问Application Frameworks层提供的硬件服务呢?在这一篇文章中,我们将在Android系统的应用层增加一个内置的应用程序,这个内置的应用程序通过ServiceManager接口获取指定的服务,然后通过这个服务来获得硬件服务。
一. 参照在Ubuntu上为Android系统的Application Frameworks层增加硬件访问服务一文,在Application Frameworks层定义好自己的硬件服务HelloService,并提供IHelloService接口提供访问服务。
二. 为了方便开发,我们可以在IDE环境下使用Android SDK来开发Android应用程序。开发完成后,再把程序源代码移植到Android源代码工程目录中。使用Eclipse的Android插件ADT创建Android工程很方便,这里不述,可以参考网上其它资料。工程名称为Hello,下面主例出主要文件:
主程序是src/shy/luo/hello/Hello.java:
1 package shy.luo.hello; 2 3 import shy.luo.hello.R; 4 import android.app.Activity; 5 import android.os.ServiceManager; 6 import android.os.Bundle; 7 import android.os.IHelloService; 8 import android.os.RemoteException; 9 import android.util.Log; 10 import android.view.View; 11 import android.view.View.OnClickListener; 12 import android.widget.Button; 13 import android.widget.EditText; 14 15 public class Hello extends Activity implements OnClickListener { 16 private final static String LOG_TAG = "shy.luo.renju.Hello"; 17 18 private IHelloService helloService = null; 19 20 private EditText valueText = null; 21 private Button readButton = null; 22 private Button writeButton = null; 23 private Button clearButton = null; 24 25 /** Called when the activity is first created. */ 26 @Override 27 public void onCreate(Bundle savedInstanceState) { 28 super.onCreate(savedInstanceState); 29 setContentView(R.layout.main); 30 31 helloService = IHelloService.Stub.asInterface( 32 ServiceManager.getService("hello")); 33 34 valueText = (EditText)findViewById(R.id.edit_value); 35 readButton = (Button)findViewById(R.id.button_read); 36 writeButton = (Button)findViewById(R.id.button_write); 37 clearButton = (Button)findViewById(R.id.button_clear); 38 39 readButton.setOnClickListener(this); 40 writeButton.setOnClickListener(this); 41 clearButton.setOnClickListener(this); 42 43 Log.i(LOG_TAG, "Hello Activity Created"); 44 } 45 46 @Override 47 public void onClick(View v) { 48 if(v.equals(readButton)) { 49 try { 50 int val = helloService.getVal(); 51 String text = String.valueOf(val); 52 valueText.setText(text); 53 } catch (RemoteException e) { 54 Log.e(LOG_TAG, "Remote Exception while reading value from device."); 55 } 56 } 57 else if(v.equals(writeButton)) { 58 try { 59 String text = valueText.getText().toString(); 60 int val = Integer.parseInt(text); 61 helloService.setVal(val); 62 } catch (RemoteException e) { 63 Log.e(LOG_TAG, "Remote Exception while writing value to device."); 64 } 65 } 66 else if(v.equals(clearButton)) { 67 String text = ""; 68 valueText.setText(text); 69 } 70 } 71 }
程序通过ServiceManager.getService("hello")来获得HelloService,接着通过IHelloService.Stub.asInterface函数转换为IHelloService接口。其中,服务名字“hello”是系统启动时加载HelloService时指定的,而IHelloService接口定义在android.os.IHelloService中,具体可以参考在Ubuntu上为Android系统的Application Frameworks层增加硬件访问服务一文。这个程序提供了简单的读定自定义硬件有寄存器val的值的功能,通过IHelloService.getVal和IHelloService.setVal两个接口实现。
1 <?xml version="1.0" encoding="utf-8"?> 2 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" 3 android:orientation="vertical" 4 android:layout_width="fill_parent" 5 android:layout_height="fill_parent"> 6 <LinearLayout 7 android:layout_width="fill_parent" 8 android:layout_height="wrap_content" 9 android:orientation="vertical" 10 android:gravity="center"> 11 <TextView 12 android:layout_width="wrap_content" 13 android:layout_height="wrap_content" 14 android:text="@string/value"> 15 </TextView> 16 <EditText 17 android:layout_width="fill_parent" 18 android:layout_height="wrap_content" 19 android:id="@+id/edit_value" 20 android:hint="@string/hint"> 21 </EditText> 22 </LinearLayout> 23 <LinearLayout 24 android:layout_width="fill_parent" 25 android:layout_height="wrap_content" 26 android:orientation="horizontal" 27 android:gravity="center"> 28 <Button 29 android:id="@+id/button_read" 30 android:layout_width="wrap_content" 31 android:layout_height="wrap_content" 32 android:text="@string/read"> 33 </Button> 34 <Button 35 android:id="@+id/button_write" 36 android:layout_width="wrap_content" 37 android:layout_height="wrap_content" 38 android:text="@string/write"> 39 </Button> 40 <Button 41 android:id="@+id/button_clear" 42 android:layout_width="wrap_content" 43 android:layout_height="wrap_content" 44 android:text="@string/clear"> 45 </Button> 46 </LinearLayout> 47 </LinearLayout>
字符串文件res/values/strings.xml:
1 <?xml version="1.0" encoding="utf-8"?> 2 <resources> 3 <string name="app_name">Hello</string> 4 <string name="value">Value</string> 5 <string name="hint">Please input a value...</string> 6 <string name="read">Read</string> 7 <string name="write">Write</string> 8 <string name="clear">Clear</string> 9 </resources>
程序描述文件AndroidManifest.xml:
1 <?xml version="1.0" encoding="utf-8"?> 2 <manifest xmlns:android="http://schemas.android.com/apk/res/android" 3 package="shy.luo.hello" 4 android:versionCode="1" 5 android:versionName="1.0"> 6 <application android:icon="@drawable/icon" android:label="@string/app_name"> 7 <activity android:name=".Hello" 8 android:label="@string/app_name"> 9 <intent-filter> 10 <action android:name="android.intent.action.MAIN" /> 11 <category android:name="android.intent.category.LAUNCHER" /> 12 </intent-filter> 13 </activity> 14 </application> 15 </manifest>
USER-NAME@MACHINE-NAME:~/Android/packages/experimental$ vi Android.mk
五. 重新打包系统镜像文件system.img:
重新打包后的system.img文件就内置了Hello.apk文件了。
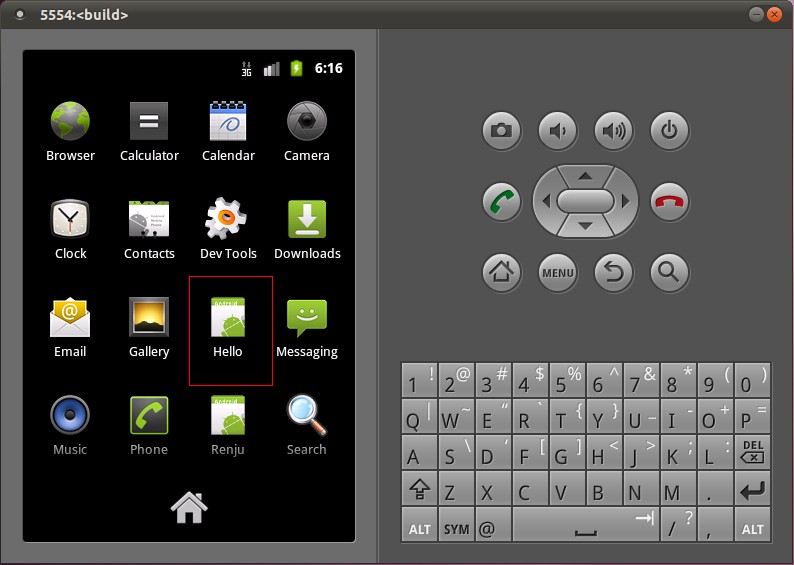
