在实际项目应用中会偶尔使用文件的压缩上传以及服务器端的加压处理,故写此文记录以备不时之需.
1.自己编写的ZipHelper类.
1 public static class ZipHelper 2 { 3 private static string pathExe = AppDomain.CurrentDomain.BaseDirectory + @"ResourceWinRAR.exe"; 4 /// <summary> 5 /// 使用Gzip方法压缩文件 6 /// </summary> 7 /// <param name="sourcefilename"></param> 8 /// <param name="zipfilename"></param> 9 /// <returns></returns> 10 public static bool GZipFile(string sourcefilename, string zipfilename) 11 { 12 bool isSucc = false; 13 //拼接压缩命令参数 14 string args = string.Format("a -as -r -afzip -ed -ibck -inul -m5 -mt5 -ep1 {0} {1}", zipfilename, sourcefilename); 15 16 //启动压缩进程 17 isSucc = ProcessHelper.StartProcess(pathExe,args); 18 return isSucc; 19 } 20 21 /// <summary> 22 /// 使用GZIP解压文件的方法 23 /// </summary> 24 /// <param name="zipfilename"></param> 25 /// <param name="unzipfilename"></param> 26 /// <returns></returns> 27 public static bool UnGzipFile(string zipfilename, string unzipfilename) 28 { 29 bool isSucc = false; 30 if (!Directory.Exists(unzipfilename)) 31 { 32 Directory.CreateDirectory(unzipfilename); 33 } 34 //拼接解压命令参数 35 string args = string.Format("x -ibck -inul -y -mt5 {0} {1}", zipfilename, unzipfilename); 36 37 //启动解压进程 38 isSucc = ProcessHelper.StartProcess(pathExe, args); 39 return isSucc; 40 } 41 }
2.用到的ProcessHelper类.
1 public class ProcessHelper 2 { 3 /// <summary> 4 /// 启动进程执行exe 5 /// </summary> 6 /// <param name="exePath">exe路径</param> 7 /// <param name="exeArgs">exe所需参数</param> 8 /// <returns></returns> 9 public static bool StartProcess(string exePath,string exeArgs) 10 { 11 bool isHidden = true; 12 bool isSucc = true; 13 Process process = new Process(); 14 process.StartInfo.FileName = exePath; 15 process.StartInfo.Arguments = exeArgs; 16 if (isHidden) 17 { 18 process.StartInfo.WindowStyle = ProcessWindowStyle.Hidden; 19 process.StartInfo.CreateNoWindow = true; 20 } 21 else 22 { 23 process.StartInfo.WindowStyle = ProcessWindowStyle.Normal; 24 process.StartInfo.CreateNoWindow = false; 25 } 26 process.Start(); 27 int idx = 1; 28 while (!process.HasExited) 29 { 30 idx++; 31 process.WaitForExit(1000); 32 if (idx == 50) 33 { 34 process.Kill(); 35 isSucc = false; 36 } 37 } 38 process.Close(); 39 process.Dispose(); 40 return isSucc; 41 } 42 }
3.WinRar相关命令解释:
/*
* <命令> -<开关1> -<开关N> <压缩文件 > <文件...> <@列表文件...> <解压路径>
*压缩 a a -as -r -afzip -ed -ibck -inul -m5 -mt5 -ep1 e: ext.zip d: ext.jpg
*解压 x x -ibck -inul -y -mt5 e: ext.zip e: ext
*a d:Info.zip D:easyui
*-af 指定格式 -afzip -afrar
*-as 在当前添加的文件列表中不存在的被压缩文件,将会从压缩文件中删除
*-df 压缩后删除源文件
*-dr 删除到回收站
*-ed 不添加空文件夹
*-hp 添加密码 -hp123456
*-ibck 后台运行
*-inul 禁止错误信息
*-loff 压缩完成后 关闭电源
*-m0 存储 添加文件到压缩文件但是不压缩
*-m1 最快 最快速的方法 ( 最低的压缩比)
*-m2 快速 快速压缩方法
*-m3 标准 标准 (默认 ) 压缩方法
*-m4 较好 较好的压缩方法 (较高的压缩比)
*-m5 最优 最优的压缩方法 (最高压缩比但是速度也最慢)
*-mtN 线程 -mt5 1~32
*-or 自动重命名文件
*-r 连同子文件
*-z 压缩后测试文件
*-y 所有弹窗选择"是"
*/
4.官方相关解压缩命令行解释:

5.使用方法,拷贝WinRar.exe到你的工程指定目录即可直接调用.
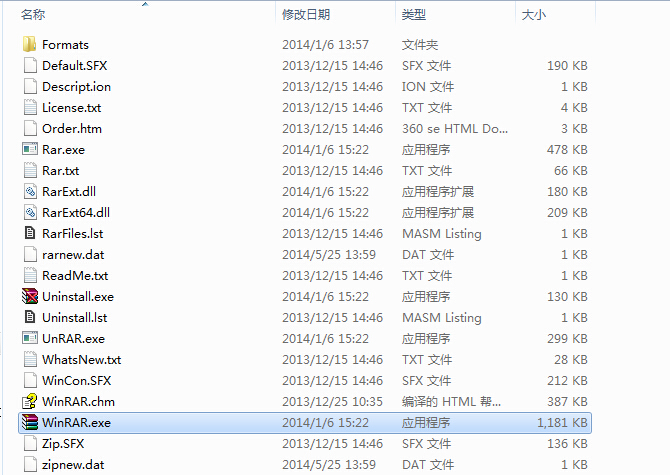