其他相关
一、isinstance(obj, cls)
检查是否obj是否是类 cls 的对象
1
2
3
4
5
6
|
class Foo( object ): pass obj = Foo() isinstance (obj, Foo) |
二、issubclass(sub, super)
检查sub类是否是 super 类的派生类
1
2
3
4
5
6
7
|
class Foo( object ): pass class Bar(Foo): pass issubclass (Bar, Foo) |
三、异常处理
1、异常基础
在编程过程中为了增加友好性,在程序出现bug时一般不会将错误信息显示给用户,而是现实一个提示的页面,通俗来说就是不让用户看见大黄页!!!
1
2
3
4
|
try : pass except Exception,ex: pass |
需求:将用户输入的两个数字相加

2、异常种类
python中的异常种类非常多,每个异常专门用于处理某一项异常!!!





对于上述实例,异常类只能用来处理指定的异常情况,如果非指定异常则无法处理。
1
2
3
4
5
6
7
|
# 未捕获到异常,程序直接报错 s1 = 'hello' try : int (s1) except IndexError,e: print e |
所以,写程序时需要考虑到try代码块中可能出现的任意异常,可以这样写:
1
2
3
4
5
6
7
8
9
|
s1 = 'hello' try : int (s1) except IndexError,e: print e except KeyError,e: print e except ValueError,e: print e |
万能异常 在python的异常中,有一个万能异常:Exception,他可以捕获任意异常,即:
1
2
3
4
5
|
s1 = 'hello' try : int (s1) except Exception,e: print e |
接下来你可能要问了,既然有这个万能异常,其他异常是不是就可以忽略了!
答:当然不是,对于特殊处理或提醒的异常需要先定义,最后定义Exception来确保程序正常运行。
1
2
3
4
5
6
7
8
9
|
s1 = 'hello' try : int (s1) except KeyError,e: print '键错误' except IndexError,e: print '索引错误' except Exception, e: print '错误' |
3、异常其他结构
1
2
3
4
5
6
7
8
9
10
11
12
|
try : # 主代码块 pass except KeyError,e: # 异常时,执行该块 pass else : # 主代码块执行完,执行该块 pass finally : # 无论异常与否,最终执行该块 pass |
4、主动触发异常
1
2
3
4
|
try : raise Exception( '错误了。。。' ) except Exception,e: print e |
5、自定义异常
1
2
3
4
5
6
7
8
9
10
11
12
|
class WupeiqiException(Exception): def __init__( self , msg): self .message = msg def __str__( self ): return self .message try : raise WupeiqiException( '我的异常' ) except WupeiqiException,e: print e |
6、断言
1
2
3
4
5
|
# assert 条件 assert 1 = = 1 assert 1 = = 2 |
四、反射
python中的反射功能是由以下四个内置函数提供:hasattr、getattr、setattr、delattr,改四个函数分别用于对对象内部执行:检查是否含有某成员、获取成员、设置成员、删除成员。
class Foo(object): def __init__(self): self.name = 'wupeiqi' def func(self): return 'func' obj = Foo() # #### 检查是否含有成员 #### hasattr(obj, 'name') hasattr(obj, 'func') # #### 获取成员 #### getattr(obj, 'name') getattr(obj, 'func') # #### 设置成员 #### setattr(obj, 'age', 18) setattr(obj, 'show', lambda num: num + 1) # #### 删除成员 #### delattr(obj, 'name') delattr(obj, 'func')
详细解析:
当我们要访问一个对象的成员时,应该是这样操作:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
class Foo( object ): def __init__( self ): self .name = 'alex' def func( self ): return 'func' obj = Foo() # 访问字段 obj.name # 执行方法 obj.func() |
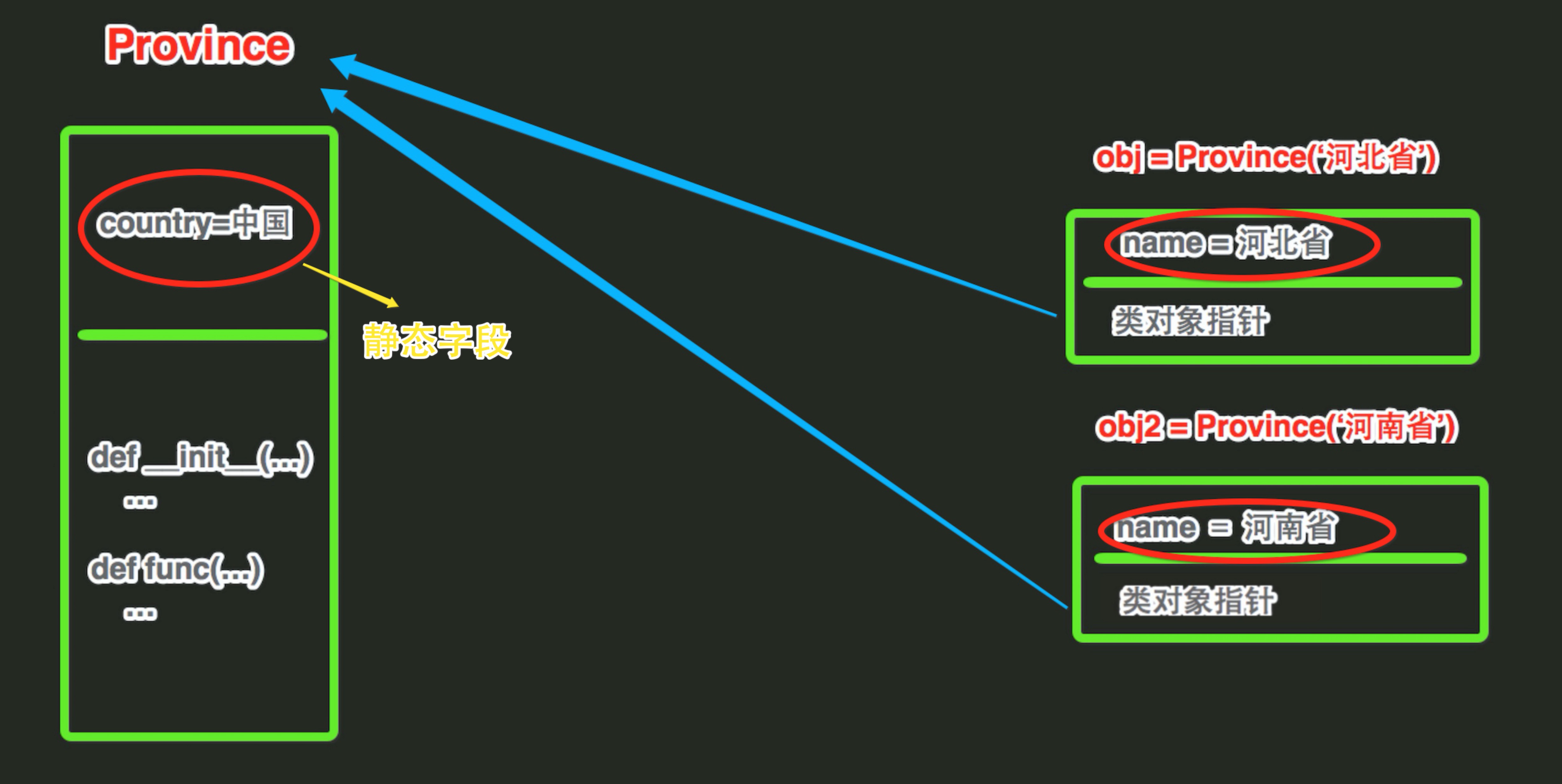



d、比较三种访问方式
- obj.name
- obj.__dict__['name']
- getattr(obj, 'name')
答:第一种和其他种比,...
第二种和第三种比,...

结论:反射是通过字符串的形式操作对象相关的成员。一切事物都是对象!!!

类也是对象
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
class Foo( object ): staticField = "old boy" def __init__( self ): self .name = 'wupeiqi' def func( self ): return 'func' @staticmethod def bar(): return 'bar' print getattr (Foo, 'staticField' ) print getattr (Foo, 'func' ) print getattr (Foo, 'bar' ) |
模块也是对象

1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
#!/usr/bin/env python # -*- coding:utf-8 -*- """ 程序目录: home.py index.py 当前文件: index.py """ import home as obj #obj.dev() func = getattr (obj, 'dev' ) func() |
拓展:
import 模块 (反射实现):
a = __import__("模块名")
a = __import__('lib.test.com', fromlist=True)