//装饰者模式:就是在保证不改变原有对象的基础上,去扩展一些想要的方法或去求
var CarInterface = new BH.Interface('CarInterface' , ['getPrice' , 'assemble']);
var Car = function(car){ //也可以这样写类。
//让子类都有这个属性
this.car = car ;
//检查接口
BH.Interface.ensureImplements(this , CarInterface);
};
Car.prototype = {
constructor :Car,
getPrice:function(){
return 200000 ;
},
assemble:function(){
document.write('组装汽车...');
}
};
var LightDecorator = function(o){ //函数调用的时候执行
//继承属性并传值,this.car = car,此时传进来的car是上面的Car对象。
LightDecorator.superClass.constructor.call(this , o);
/*
相当于复制代码:this.car = car ;
BH.Interface.ensureImplements(this , CarInterface);
*/
};
BH.extend(LightDecorator , Car); //立即执行
LightDecorator.prototype = {
constructor:LightDecorator ,
getPrice:function(){
return this.car.getPrice() + 10000;
},
assemble:function(){
document.write('组装车灯...');
}
};
var IceBoxDecorator = function(o){
//继承属性并传值,this.car = car,此时传进来的car是上面的LightDecorator对象。js没有多态概念,
IceBoxDecorator.superClass.constructor.call(this , o);
};
BH.extend(IceBoxDecorator , Car); //原型继承
IceBoxDecorator.prototype = {
constructor:IceBoxDecorator ,
getPrice:function(){
return this.car.getPrice() + 20000;
},
assemble:function(){
document.write('组装车载冰箱...');
}
};
var car = new Car();
alert(car.getPrice());
car.assemble();
var L = new LightDecorator(car);
alert(L.getPrice());
L.assemble();
var I = new IceBoxDecorator(L);
alert(I.getPrice());
I.assemble();
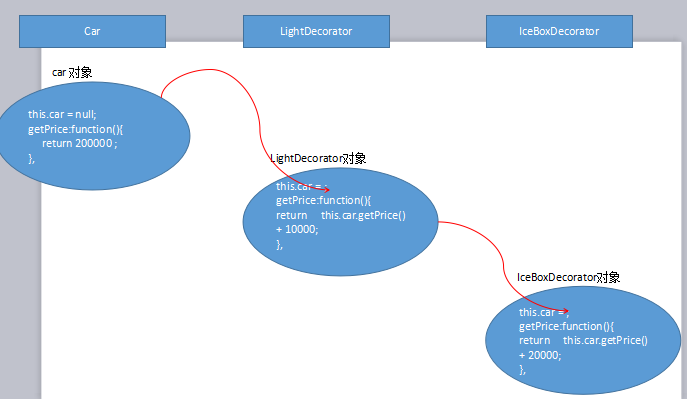
//装饰者 不仅可以用在类上, 还可以用在函数上
//返回一个当前时间的字符串表示形式
function getDate(){
return (new Date()).toString();
};
// 包装函数 (装饰者函数)
function upperCaseDecorator(fn){
return function(){
return fn.apply(this, arguments).toUpperCase();
}
};
alert(getDate());
var getDecoratorDate = upperCaseDecorator(getDate);
alert(getDecoratorDate());