前言:
SpringBoot的项目已经对有一定的异常处理了,但是对于我们开发者而言可能就不太合适了,因此我们需要对这些异常进行统一的捕获并处理。SpringBoot中有一个ControllerAdvice
的注解,使用该注解表示开启了全局异常的捕获,我们只需在自定义一个方法使用ExceptionHandler
注解然后定义捕获异常的类型即可对这些捕获的异常进行统一的处理。
最终结果:
自定义基础接口类
定义一个基础的接口类,自定义的错误描述枚举类需实现该接口。
代码如下:
public interface BaseErrorInfoInterface {
/** 错误码
*/
String getCode();
/** 错误描述*/
String getMsg();
}
自定义枚举类
然后自定义一个枚举类,并实现该接口。
代码如下:
import lombok.Getter;
@Getter
public enum ErrorCodeAndMsg implements BaseErrorInfoInterface{
SUCCESS("200", "成功!"),
BODY_NOT_MATCH("400","请求的数据格式不符!"),
SIGNATURE_NOT_MATCH("401","请求的数字签名不匹配!"),
NOT_FOUND("404", "未找到该资源!"),
INTERNAL_SERVER_ERROR("500", "服务器内部错误!"),
SERVER_BUSY("503","服务器正忙,请稍后再试!"),
STUDENG_NULL("0003", "学号不能为空");
private String code;
private String msg;
ErrorCodeAndMsg(String code, String msg) {
this.code = code;
this.msg = msg;
}
public String getCode() {
return code;
}
public String getMsg() {
return msg;
}
}
自定义异常类
定义一个异常类,用于处理我们发生的业务异常。
代码如下:
import lombok.Getter;
/**
* 统一异常捕获类
* Created by Tiger on 2018/10/9.
*/
@Getter
public class StudentException extends RuntimeException{
private String code;
private String msg;
public StudentException() {
super();
}
public StudentException(BaseErrorInfoInterface errorInfoInterface) {
super(errorInfoInterface.getCode());
this.code = errorInfoInterface.getCode();
this.msg = errorInfoInterface.getMsg();
}
public StudentException(BaseErrorInfoInterface errorInfoInterface, Throwable cause) {
super(errorInfoInterface.getCode(), cause);
this.code = errorInfoInterface.getCode();
this.msg = errorInfoInterface.getMsg();
}
public StudentException(String errorMsg) {
super(errorMsg);
this.msg = errorMsg;
}
public StudentException(String errorCode, String errorMsg) {
super(errorCode);
this.code = errorCode;
this.msg = errorMsg;
}
public StudentException(String errorCode, String errorMsg, Throwable cause) {
super(errorCode, cause);
this.code = errorCode;
this.msg = errorMsg;
}
public String getErrorCode() {
return code;
}
public void setErrorCode(String errorCode) {
this.code = errorCode;
}
public String getErrorMsg() {
return msg;
}
public void setErrorMsg(String errorMsg) {
this.msg = errorMsg;
}
public String getMessage() {
return msg;
}
@Override
public Throwable fillInStackTrace() {
return this;
}
}
自定义数据格式
顺便这里定义一下数据的传输格式。
代码如下:
public class ResultBody {
/**
* 响应代码
*/
private String code;
/**
* 响应消息
*/
private String message;
/**
* 响应结果
*/
private Object result;
public ResultBody() {
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public Object getResult() {
return result;
}
public void setResult(Object result) {
this.result = result;
}
/**
* 成功
*
* @return
*/
public static ResultBody success() {
return success(null);
}
/**
* 成功
* @param data
* @return
*/
public static ResultBody success(Object data) {
ResultBody rb = new ResultBody();
rb.setCode(ErrorCodeAndMsg.SUCCESS.getCode());
rb.setMessage(ErrorCodeAndMsg.SUCCESS.getMsg());
rb.setResult(data);
return rb;
}
/**
* 失败
*/
public static ResultBody error(BaseErrorInfoInterface errorInfo) {
ResultBody rb = new ResultBody();
rb.setCode(errorInfo.getCode());
rb.setMessage(errorInfo.getMsg());
rb.setResult(null);
return rb;
}
/**
* 失败
*/
public static ResultBody error(String code, String message) {
ResultBody rb = new ResultBody();
rb.setCode(code);
rb.setMessage(message);
rb.setResult(null);
return rb;
}
/**
* 失败
*/
public static ResultBody error( String message) {
ResultBody rb = new ResultBody();
rb.setCode("-1");
rb.setMessage(message);
rb.setResult(null);
return rb;
}
/*@Override
public String toString() {
return JSONObject.toJSONString(this);
}*/
}
自定义全局异常处理类
最后我们在来编写一个自定义全局异常处理的类。
代码如下:
@ControllerAdvice
public class GlobalExceptionHandler {
private static final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
/**
* 处理自定义的业务异常
* @param req
* @param e
* @return
*/
@ExceptionHandler(value = BizException.class)
@ResponseBody
public ResultBody bizExceptionHandler(HttpServletRequest req, BizException e){
logger.error("发生业务异常!原因是:{}",e.getErrorMsg());
return ResultBody.error(e.getErrorCode(),e.getErrorMsg());
}
/**
* 处理空指针的异常
* @param req
* @param e
* @return
*/
@ExceptionHandler(value =NullPointerException.class)
@ResponseBody
public ResultBody exceptionHandler(HttpServletRequest req, NullPointerException e){
logger.error("发生空指针异常!原因是:",e);
return ResultBody.error(CommonEnum.BODY_NOT_MATCH);
}
/**
* 处理其他异常
* @param req
* @param e
* @return
*/
@ExceptionHandler(value =Exception.class)
@ResponseBody
public ResultBody exceptionHandler(HttpServletRequest req, Exception e){
logger.error("未知异常!原因是:",e);
return ResultBody.error(CommonEnum.INTERNAL_SERVER_ERROR);
}
}
控制层
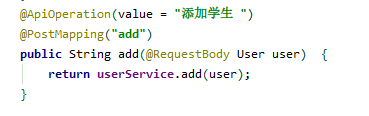
业务层
完成
原文地址:https://www.cnblogs.com/xuwujing/p/10933082.html