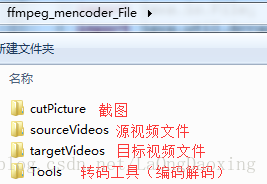
VideoToPicUtil.java
1 package com.zhwy.util;
2
3 import java.io.File;
4 import java.util.ArrayList;
5 import java.util.Calendar;
6 import java.util.List;
7 /**
8 * 视频转码_截图
9 */
10 public class VideoToPicUtil {
11
12 public static void main(String[] args) {
13 String videoPathURL = "D:/ffmpeg_mencoder_File/sourceVideos/短视频.mp4";//源视频文件路径
14 VideoToPic(videoPathURL);
15 }
16 /**
17 * 视频截图工具
18 * @param videoPath 源视频文件路径
19 * @return
20 */
21 public static String VideoToPic(String videoPath){
22 if (!is_File(videoPath)) { //判断路径是不是一个文件
23 System.out.println(videoPath + " is not file");
24 }
25 String VideoToPicResult=executeCodecs(videoPath);
26 return VideoToPicResult;
27 }
28
29 /**
30 * 判断路径是不是一个文件
31 *
32 * @param file
33 * 源视频文件路径
34 */
35 private static boolean is_File(String path) {
36 File file = new File(path);
37 if (!file.isFile()) {
38 return false;
39 }
40 return true;
41 }
42
43 /**
44 * 判断视频格式
45 */
46 private static int is_VideoType(String srcFilePath) {
47 String type = srcFilePath.substring(srcFilePath.lastIndexOf(".") + 1, srcFilePath.length()).toLowerCase();
48 // ffmpeg能解析的格式:(asx,asf,mpg,wmv,3gp,mp4,mov,avi,flv等)
49 if (type.equals("avi")) {
50 return 0;
51 } else if (type.equals("mpg")) {
52 return 0;
53 } else if (type.equals("wmv")) {
54 return 0;
55 } else if (type.equals("3gp")) {
56 return 0;
57 } else if (type.equals("mov")) {
58 return 0;
59 } else if (type.equals("mp4")) {
60 return 0;
61 } else if (type.equals("asf")) {
62 return 0;
63 } else if (type.equals("asx")) {
64 return 0;
65 } else if (type.equals("flv")) {
66 return 0;
67 }
68 // 对ffmpeg.exe无法解析的文件格式(wmv9,rm,rmvb等),可以先用别的工具(mencoder.exe)转换为.avi(ffmpeg能解析的格式).
69 else if (type.equals("wmv9")) {
70 return 1;
71 } else if (type.equals("rm")) {
72 return 1;
73 } else if (type.equals("rmvb")) {
74 return 1;
75 }
76 return 9;
77 }
78 /**
79 * 源视频转换成AVI格式
80 *
81 * @param type
82 * 视频格式
83 */
84 // 对ffmpeg.exe无法解析的文件格式(wmv9,rm,rmvb等), 可以先用别的工具(mencoder.exe)转换为avi(ffmpeg能解析的)格式.
85 private static String convertToAVI(int type,String srcFilePath) {
86 String mencoderPath = "D:\ffmpeg_mencoder_File\Tools\mencoder.exe"; // 转换工具路径
87 String fileNameWithoutSuffix=getFileNameWithoutSuffix(srcFilePath);
88 String codcFilePath ="D:\ffmpeg_mencoder_File\targetVideos\"+fileNameWithoutSuffix+".avi";//【存放转码后视频的路径,记住一定是.avi后缀的文件名】
89 List<String> commend = new ArrayList<String>();
90 commend.add(mencoderPath);
91 commend.add(srcFilePath);
92 commend.add("-oac");
93 commend.add("lavc");
94 commend.add("-lavcopts");
95 commend.add("acodec=mp3:abitrate=64");
96 commend.add("-ovc");
97 commend.add("xvid");
98 commend.add("-xvidencopts");
99 commend.add("bitrate=600");
100 commend.add("-of");
101 commend.add("avi");
102 commend.add("-o");
103 commend.add(codcFilePath);
104 try {
105 //调用线程命令启动转码
106 ProcessBuilder builder = new ProcessBuilder();
107 builder.command(commend);
108 builder.start();
109 return codcFilePath;
110 } catch (Exception e) {
111 e.printStackTrace();
112 return null;
113 }
114 }
115
116 /**
117 * 源视频转换成FLV格式
118 * @param srcFilePathParam
119 * 源:用于指定要转换格式的文件,要截图的源视频文件路径
120 */
121 // ffmpeg能解析的格式:(asx,asf,mpg,wmv,3gp,mp4,mov,avi,flv等)
122 private static String convertToFLV(String srcFilePathParam) {
123 if (!is_File(srcFilePathParam)) {
124 System.out.println(srcFilePathParam + " is not file");
125 }
126 // 文件命名
127 String fileNameWithoutSuffix=getFileNameWithoutSuffix(srcFilePathParam);
128 String codcFilePath ="D:\ffmpeg_mencoder_File\targetVideos\"+fileNameWithoutSuffix+".flv";//【存放转码后视频的路径,记住一定是.flv后缀的文件名】
129 String mediaPicPath ="d:\ffmpeg_mencoder_File\cutPicture\"+ fileNameWithoutSuffix+".jpg";
130 System.out.println("fileNameWithoutSuffix:"+fileNameWithoutSuffix);
131 Calendar c = Calendar.getInstance();
132 String savename = String.valueOf(c.getTimeInMillis())+ Math.round(Math.random() * 100000);
133 String ffmpegPath = "D:\ffmpeg_mencoder_File\Tools\ffmpeg.exe";
134 List<String> commend = new ArrayList<String>();
135 commend.add(ffmpegPath);
136 commend.add("-i");
137 commend.add(srcFilePathParam);
138 commend.add("-ab");
139 commend.add("56");
140 commend.add("-ar");
141 commend.add("22050");
142 commend.add("-qscale");
143 commend.add("8");
144 commend.add("-r");
145 commend.add("15");
146 commend.add("-s");
147 commend.add("600x500");
148 commend.add(codcFilePath);
149 String cutPicPath =null;
150 try {
151 Runtime runtime = Runtime.getRuntime();
152 Process proce = null;
153 cutPicPath = " D:\ffmpeg_mencoder_File\Tools\ffmpeg.exe -i "
154 + srcFilePathParam
155 + " -y -f image2 -ss 2 -t 0.001 -s 600x500 "+ mediaPicPath; //截图文件的保存路径
156 proce = runtime.exec(cutPicPath);
157 //调用线程命令进行转码
158 ProcessBuilder builder = new ProcessBuilder(commend);
159 builder.command(commend);
160 builder.start();
161 } catch (Exception e) {
162 e.printStackTrace();
163 }
164 String tempFile= codcFilePath;
165 boolean status=deleteAVIFile(tempFile);//删除转码后的目标文件
166 System.out.println("是否删除成功呢"+status);
167 return cutPicPath;
168 }
169 /**
170 * 获取不带后缀名的文件名
171 * @param fileUrl 源视频文件路径
172 */
173 public static String getFileNameWithoutSuffix(String fileUrl){
174 File file=new File(fileUrl);
175 String fileNameWithoutSuffix=file.getName().replaceAll("[.][^.]+$", "");
176 System.out.println("获取不带后缀名的文件名方法中输出:"+fileNameWithoutSuffix);
177 return fileNameWithoutSuffix;
178 }
179 /**
180 * 删除转换后的单个目标视频文件
181 * @param tempFile 转换后的目标视频文件
182 * @return 单个文件删除成功返回true,否则返回false
183 */
184 public static boolean deleteAVIFile(String tempFile) {
185 File file = new File(tempFile);
186 if (file.exists()==true) {
187 if (file.delete()==true) {
188 return true;
189 }else{
190 return false;
191 }
192 }else{
193 return false;
194 }
195 }
196
197 /**
198 * 视频转码(mencoder.exe或ffmpeg.exe执行编码解码)
199 */
200 private static String executeCodecs(String videoPath) {
201 // 判断视频的类型
202 int type = is_VideoType(videoPath);
203 String picResult = null;
204 //如果是ffmpeg可以转换的类型直接转码,否则先用mencoder转码成AVI
205 if (type == 0) {
206 System.out.println("直接将文件转为flv文件");
207 picResult = convertToFLV(videoPath);// 直接将文件转为flv文件 ,并返回截图路径
208 } else if (type == 1) { //
209 String codcFilePath = convertToAVI(type,videoPath); //视频格式转换后的目标视频文件路径
210
211 if (codcFilePath == null){
212 String message="avi文件没有得到";
213 return message;// avi文件没有得到
214 }
215 picResult = convertToFLV(codcFilePath);// 将avi转为flv,并返回截图路径
216 }
217 return picResult;
218 }
219 }