java.util.Map接口中常用方法:
1 Map和Collection没有继承关系
2 Map集合以key和value的方式存储数据:键值对
key和value都是引用数据类型。
key和value都是存储对象的内存地址。
key起到主导的地位,value是key的一个附属品。
3 Map接口中常用方法:
V put(K key, V value) 向Map集合中添加键值对
V get(Object key) 通过key获取value
void clear() 清空Map集合
boolean containsKey(Object key) 判断Map中是否包含某个key
boolean containsValue(Object value) 判断Map中是否包含某个value
boolean isEmpty() 判断Map集合中的元素哥数是否为0
V remove(Object key) 通过key删除键值对
int size() 获取Map集合中键值对的个数
Collection<V> values() 获取Map集合中所有的value,返回一个Collection
Set<K> keySet() 获取Map集合所有的key
Set<Map.Entry<K,V>> entrySet() ---> Map.Entry(Map中的静态内部类Entry)
将Map集合转换成Set集合
假设现在有一个Map集合,如下所示:
map1集合对象
key values
-----------------------
1 zhangsan
2 lisi
3 wangwu
Set set = map1.entrySet();
set集合对象
1 = zhangsan 【注意:Map集合通过entrySet()方法转换成的这个Set集合,Set集合中元素的类型是Map.entry[K,V]】
2 = lisi 【Map.entry和String一样,都是一种类型的名字,只不过:Map.Entry是静态内部类,是Map中的静态内部类。】
3 = wangwu ---> 这个东西是个啥? 是个这个东西Map.Entry
Map的结构图:
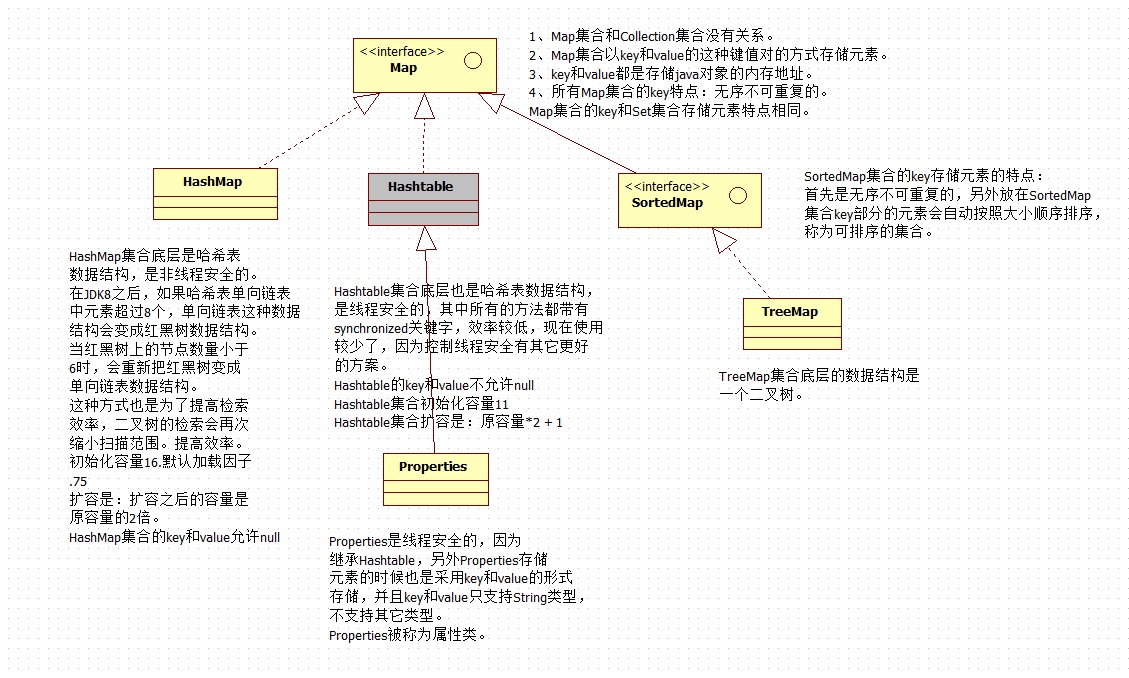
案例1:
package com.javaSe.Map; import java.util.Collection; import java.util.HashMap; import java.util.Map; /* java.util.Map接口中常用方法: 1 Map和Collection没有继承关系 2 Map集合以key和value的方式存储数据:键值对 key和value都是引用数据类型。 key和value都是存储对象的内存地址。 key起到主导的地位,value是key的一个附属品。 3 Map接口中常用方法: V put(K key, V value) 向Map集合中添加键值对 V get(Object key) 通过key获取value void clear() 清空Map集合 boolean containsKey(Object key) 判断Map中是否包含某个key boolean containsValue(Object value) 判断Map中是否包含某个value boolean isEmpty() 判断Map集合中的元素哥数是否为0 V remove(Object key) 通过key删除键值对 int size() 获取Map集合中键值对的个数 Collection<V> values() 获取Map集合中所有的value,返回一个Collection Set<K> keySet() 获取Map集合所有的key Set<Map.Entry<K,V>> entrySet() ---> Map.Entry(Map中的静态内部类Entry) 将Map集合转换成Set集合 假设现在有一个Map集合,如下所示: map1集合对象 key values ----------------------- 1 zhangsan 2 lisi 3 wangwu Set set = map1.entrySet(); set集合对象 1 = zhangsan 【注意:Map集合通过entrySet()方法转换成的这个Set集合,Set集合中元素的类型是Map.entry[K,V]】 2 = lisi 【Map.entry和String一样,都是一种类型的名字,只不过:Map.Entry是静态内部类,是Map中的静态内部类。】 3 = wangwu ---> 这个东西是个啥? 是个这个东西Map.Entry */ public class MapTest01 { public static void main(String[] args) { // 创建Map集合 Map<Integer,String> map = new HashMap<>(); // 向Map中添加数据 map.put(1,"zhangsan"); // 1在这里自动装箱了。 map.put(2,"lisi"); map.put(3,"wangwu"); map.put(4,"liuliu"); // 通过key获取value String value = map.get(2); System.out.println(value); // 获取键值对的数量 System.out.println("键值对的数量是:" + map.size()); // 通过key删除key value map.remove(2); System.out.println("键值对的数量是:" + map.size()); // 判断是否包含某个key // contains方法底层调用的都是equals进行比对的,所以自定义的类型需要重写equals方法。 // System.out.println(map.containsKey(3)); // true System.out.println(map.containsKey(new Integer(3))); // true // 判断是否包含某个values // System.out.println(map.containsValue("liuliu")); //true System.out.println(map.containsValue(new String("liuliu"))); //true // 获取所有的value Collection<String> values = map.values(); for(String s : values){ System.out.println(s); } // 清空Map中的元素 map.clear(); System.out.println("键值对的数量是:" + map.size()); // 判断是否为空 System.out.println(map.isEmpty());//true } }
案例2:
package com.javaSe.Map; import java.util.*; /* Map集合的遍历 */ public class MapTest02 { public static void main(String[] args) { // 第一种方式:获取所有的key,通过遍历key,来获取value // 创建Map集合 Map<Integer, String> map = new HashMap<>(); // 向Map中添加数据 map.put(1, "zhangsan"); // 1在这里自动装箱了。 map.put(2, "lisi"); map.put(3, "wangwu"); map.put(4, "liuliu"); // 遍历Map集合 // 获取所有的key,所有的key是一个Set集合 Set<Integer> keys = map.keySet(); // 遍历key,通过key获取value // 迭代器可以 Iterator<Integer> it = keys.iterator(); while (it.hasNext()){ Integer key = it.next(); // 取出其中一个key String value = map.get(key); // 通过key取出value System.out.println(key + "=" + value); } System.out.println("---------------------------------------"); // foreach也可以 for(Integer key : keys){ System.out.println(key + "=" + map.get(key)); } System.out.println("---------------------------------------"); // 第二种方式: Set<Map.Entry<K,V>> entrySet() // 以上这个方法是把Map集合直接全部转换成Set集合。 // Set集合中元素的类型是:Map.Entry Set<Map.Entry<Integer,String>> set = map.entrySet(); // 遍历Set集合,每一次取出一个Node // 迭代器 Iterator<Map.Entry<Integer,String>> it2 = set.iterator(); while (it2.hasNext()){ Map.Entry<Integer,String> node = it2.next(); Integer key = node.getKey(); String value = node.getValue(); System.out.println(key + "=" + value); } System.out.println("---------------------------------------"); // foreach // 这种方式效率比较高,因为获取key和value都是直接从node对象中获取的属性值。 // 这种方式比较适合大数据量。 for(Map.Entry<Integer,String> node : set){ System.out.println(node.getKey() + "=" + node.getValue()); } } }