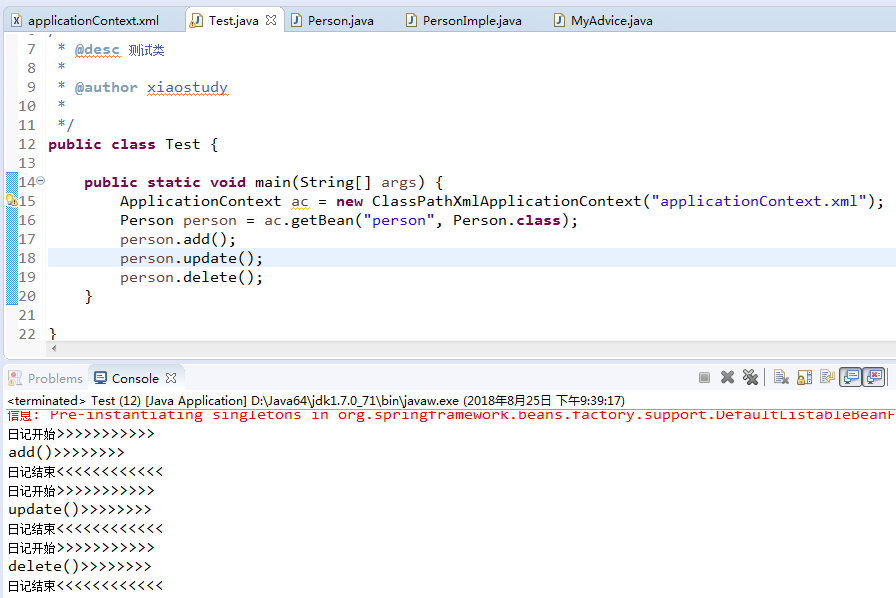
1、被代理类接口Person.java
1 package com.xiaostudy;
2
3 /**
4 * @desc 被代理类接口
5 *
6 * @author xiaostudy
7 *
8 */
9 public interface Person {
10
11 public void add();
12 public void update();
13 public void delete();
14 }
2、被代理类PersonImple.java
1 package com.xiaostudy;
2
3 /**
4 * @desc 被代理类
5 *
6 * @author xiaostudy
7 *
8 */
9 public class PersonImple implements Person {
10
11 /**
12 * @desc 实现接口方法
13 */
14 public void add() {
15 System.out.println("add()>>>>>>>>");
16 }
17
18 @Override
19 public void update() {
20 System.out.println("update()>>>>>>>>");
21 }
22
23 @Override
24 public void delete() {
25 System.out.println("delete()>>>>>>>>");
26 }
27
28 }
3、切面类MyAdvice.java
1 package com.xiaostudy;
2
3 import org.aopalliance.intercept.MethodInterceptor;
4 import org.aopalliance.intercept.MethodInvocation;
5
6 /**
7 * @desc 切面类
8 *
9 * @author xiaostudy
10 *
11 */
12 public class MyAdvice implements MethodInterceptor {
13
14 /**
15 * @desc 环绕通知
16 */
17 @Override
18 public Object invoke(MethodInvocation method) throws Throwable {
19 System.out.println("日记开始>>>>>>>>>>>");
20 method.proceed();
21 System.out.println("日记结束<<<<<<<<<<<<");
22 return null;
23 }
24
25 }
4、spring的配置文件applicationContext.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2 <beans xmlns="http://www.springframework.org/schema/beans"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xmlns:aop="http://www.springframework.org/schema/aop"
5 xsi:schemaLocation="http://www.springframework.org/schema/beans
6 http://www.springframework.org/schema/beans/spring-beans.xsd
7 http://www.springframework.org/schema/aop
8 http://www.springframework.org/schema/aop/spring-aop.xsd">
9 <!-- 创建被代理类 -->
10 <bean id="person" class="com.xiaostudy.PersonImple"></bean>
11 <!-- 创建切面类 -->
12 <bean id="advice" class="com.xiaostudy.MyAdvice"></bean>
13 <!-- spring的AOP编程,proxy-target-class是非必须项,其默认值是false,就是说使用动态代理,这里设置为true,就是说使用cglib代理 -->
14 <aop:config proxy-target-class="true">
15 <!-- 切入点,expression是选择切入方法,id是给这个切入点命名
16 execetion是切入表达式
17 1、第一个*:表示方法的返回值是任意
18 2、第二个*:表示xiaostudy包下的类名是任意,也就是说只要是这个包下的类都可以
19 3、第三个*:表示类方法是任意
20 4、括号里面的两个.:表示方法的参数是任意
21 -->
22 <aop:pointcut expression="execution(* com.xiaostudy.*.*(..))" id="myPointcut"/>
23 <!-- 特殊的切面,advice-ref是切面类id,pointcut-ref是切入点的id -->
24 <aop:advisor advice-ref="advice" pointcut-ref="myPointcut"/>
25 </aop:config>
26
27 </beans>
5、测试类Test.java
1 package com.xiaostudy;
2
3 import org.springframework.context.ApplicationContext;
4 import org.springframework.context.support.ClassPathXmlApplicationContext;
5
6 /**
7 * @desc 测试类
8 *
9 * @author xiaostudy
10 *
11 */
12 public class Test {
13
14 public static void main(String[] args) {
15 ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml");
16 Person person = ac.getBean("person", Person.class);
17 person.add();
18 person.update();
19 person.delete();
20 }
21
22 }