Introduction
In this post I want to share my work on developing Chatting application that can be used in your regular ASP.Net applications. I am using AJAX and SQL server for backend. This can be used in ASP.Net 2.0 or more, as I am using the UpdatePanel.
Using the code
The application works as follows -
- List of online users are displayed.
- Online users list can be loaded from the backend database, logging an entry when a user logs into the application, the user names will be displayed as hyperlinks with a unique chat id for each.
- The current logged-in user will click on a selected to user name to open a new chat window, and types a message.
- On click of the username OpenChatBox() function is called with the chatid as parameter. When the user types any message and clicks on Send button, the message is sent to server and stored to the database with chat id supplied.
function OpenChatBox(uid, chatid) { var win = window.open("ChatBox.aspx?uid=" + uid + "&cid=" + chatid, "ChatWindow" + chatid + document.getElementById('hidCurrentUser').value, "status=0,toolbar=0, menubar=0, width=450, height=550"); top = top + 50; if (top > screen.availHeight - 550) top = 100; left = left + 50; if (left > screen.availWidth - 450) left = 100; win.moveTo(left, top); chats[chats.length] = win; return false; }
The storing of message is implemented in ASP.Net.Private Sub SendMessage(ByVal msg As String) Dim con As SqlConnection = New SqlConnection( _ "Data Source=.\SQLEXPRESS;AttachDbFilename=D:\Dev\Projects\WebChat\WebChat\App_Data\WebChat.mdf;Integrated Security=True;User Instance=True") con.Open() Dim comm As SqlCommand = New SqlCommand("stp_SendMessage", con) comm.CommandType = CommandType.StoredProcedure Dim paramSender As SqlParameter = New SqlParameter( _ "@Sender", SqlDbType.VarChar, 10) paramSender.Value = HttpContext.Current.User.Identity.Name comm.Parameters.Add(paramSender) Dim paramRec As SqlParameter = New SqlParameter( _ "@Reciever", SqlDbType.VarChar, 10) paramRec.Value = ViewState("uid") comm.Parameters.Add(paramRec) Dim paramMsg As SqlParameter = New SqlParameter( _ "@Msg", SqlDbType.VarChar, 1000) paramMsg.Value = msg comm.Parameters.Add(paramMsg) 'store the chat id supplied from browser Dim paramChatId As SqlParameter = New SqlParameter( _ "@ChatId", SqlDbType.VarChar, 100) paramChatId.Value = ViewState("vwChatId").ToString() comm.Parameters.Add(paramChatId) comm.ExecuteNonQuery() con.Close() End Sub
- On the recipient's screen a new pop-up window will appear.
- One every users home page a script is run for every 5 seconds to ping the server for any messages.
function PingServer() { PingServerForMsg() setTimeout(PingServer, 5000); } function PingServerForMsg() { // Use ajax to call the GetChatMsg.aspx to get any new messages. xmlHttp = GetXmlHttpObject(stateChangeHandler); }
This javascript message will call the GetChatMsg.aspx page for the new messages, and below is the code for GetChatMsg.aspxImports System.Data Imports System.Data.SqlClient Partial Public Class GetChatMsg Inherits System.Web.UI.Page Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load Response.Clear() Response.ClearContent() 'set response to not to cache, to avoid the ajax from displaying old message. Response.AddHeader("Cache-Control", "no-cache, must-revalidate") Dim ds As DataSet = GetMessage(HttpContext.Current.User.Identity.Name) Dim strMsg As String = "" If ds.Tables.Count > 0 Then If ds.Tables(0).Rows.Count > 0 Then For Each dr As DataRow In ds.Tables(0).Rows If strMsg.Length > 0 Then strMsg += "#" End If strMsg += dr("Sender").ToString() + "&" strMsg += dr("ChatId").ToString() + "&" strMsg += dr("Msg").ToString().Replace("&", "_@AMP__").Replace("#", "_@HASH__") Next End If End If Response.Write(strMsg) Response.End() End Sub Private Function GetMessage(ByVal strUid As String) As DataSet Dim dsMsgs As DataSet = New DataSet() Dim con As SqlConnection = New SqlConnection( _ "Data Source=.\SQLEXPRESS;AttachDbFilename=D:\Dev\Projects\WebChat\WebChat\App_Data\WebChat.mdf;Integrated Security=True;User Instance=True") con.Open() Dim comm As SqlCommand = New SqlCommand("stp_GetMessage", con) comm.CommandType = CommandType.StoredProcedure Dim paramSender As SqlParameter = New SqlParameter( _ "@Uid", SqlDbType.VarChar, 10) paramSender.Value = HttpContext.Current.User.Identity.Name comm.Parameters.Add(paramSender) If (Request.QueryString("cid") <> Nothing) Then Dim paramChatId As SqlParameter = New SqlParameter( _ "@ChatId", SqlDbType.VarChar, 100) paramChatId.Value = Request.QueryString("cid") comm.Parameters.Add(paramChatId) End If Dim da As SqlDataAdapter = New SqlDataAdapter(comm) da.Fill(dsMsgs) con.Close() Return dsMsgs End Function End Class
On the browser side on getting new message, the browser will open the pop-up if the chatid is new, or will show the message if the pop-up is already open for the chatid associated with the new message.function stateChangeHandler() { //readyState of 4 or 'complete' represents that data has been returned if (xmlHttp.readyState == 4 || xmlHttp.readyState == 'complete') { //Gather the results from the callback var str = xmlHttp.responseText; if (str != "" ) { //document.getElementById('txtMsg').value = str; //eval(str); var msgs = str.split('#'); for (ind = 0; ind < msgs.length; ind++) { msgs[ind] = msgs[ind].replace("_@HASH__", "#"); var msg = msgs[ind].split("&"); msg[0] = msg[0].replace("_@AMP__", "&"); msg[1] = msg[1].replace("_@AMP__", "&"); msg[2] = msg[2].replace("_@AMP__", "&"); var blnExisting = false; for (iind = 0; iind < chats.length; iind++) { try { if (chats[iind] != null && chats[iind].name == "ChatWindow" + msg[1] + document.getElementById('hidCurrentUser').value) blnExisting = true; } catch(e){} } if( blnExisting == false) OpenChatBox(msg[0], msg[1]); } } } }
- The users can communicate through the current window.
- The recipient can type in the message to reply back using the same window. The pop-up window has the screen ChatBox.aspx, which has the ChatBox.js reference of javascript code to ping the server again for every 1 sec. But this time it uses the Chat id assigned to the that window. And thus every chat has a unique chat id, and chat messages are maintained at the backend by the chatid and pop-up windows communicate through the chat id. An update panel is used here to avoid the flickering of the chat window when user clicks send message. And when the message is recieved by the recipient the message is deleted by the server.
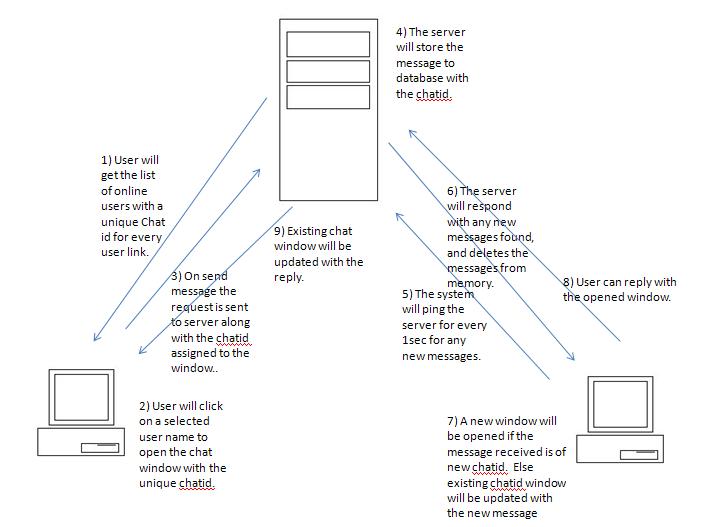
- A single user can communicate with multiple users at a time.
- The user can chat with any number of users as the pop-up windows are used for each chat with the unique chat id for the chat. The chat id is generated as 'Timestamp + Senderid + recipient id'.
On click of the send button the typed message will be saved by the code behind to a table in SQL Server (here any other database can be used). On recipient side on ajax call the message reader will read the messages related to the recipient and send to recipient. Please check the attached source code for ASP.Net code which is for Visual Studio 2008, but same code can be used for VS 2005 (ASP.Net 2.0) with out changes.
When the First user sends a message, the message is saved to backend for the selected user. When the application running on the recipient (implemented in default.aspx in sample) uses the Ajax to ping the server for messages for each 5 seconds.
Enhancements
- Developers can try to implement saving Chat History.
- The list of online users can be implemented in a user control to make it reusable.
- Chat window can be changed to include time of message and to include images.