队列是一种常见的数据结构,是一种有序列表,可以使用数组或者链表实现,
队列遵循先入先出规则,常见的应用场景有:银行叫号系统,政务大厅排号系统等,使用数组模拟队列示意图如下:
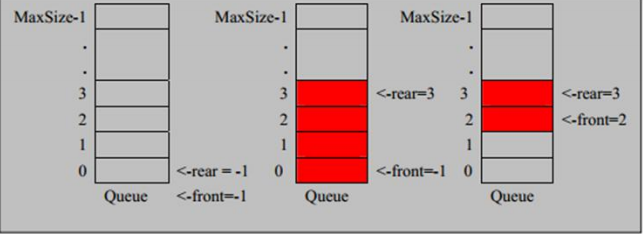
下面是代码:
1 public class CircleArrayQueueDemo {
2 public static void main(String[] args) {
3 // 测试,创建队列 最大值为 3 方便测试
4 CircleArrayQueue aq = new CircleArrayQueue(4);
5 char key = ' ';// 接收用户输入
6 Scanner input = new Scanner(System.in);
7 boolean loop = true;
8 while (loop) {
9 System.out.println("s(show) 显示队列");
10 System.out.println("e(exit) 退出程序");
11 System.out.println("a(add) 添加队列");
12 System.out.println("g(get) 取出队列");
13 System.out.println("h(head) 展示队列");
14 key = input.next().charAt(0);// 接收输入字符
15 switch (key) {
16 case 's':
17 aq.showQueue();
18 break;
19 case 'a':
20 System.out.println("请输入数字:");
21 int v = input.nextInt();
22 aq.addQueue(v);
23 break;
24 case 'g':
25 try {
26 int reslut = aq.getQueue();
27 System.out.println("取出的数据是 -- " + reslut);
28 } catch (Exception e) {
29 System.out.println(e.getMessage());
30 }
31 break;
32 case 'h':// 查看队列头
33 try {
34 int head = aq.headQueue();
35 System.out.println("队列头的数据是 -- " + head);
36 } catch (Exception e) {
37 System.out.println(e.getMessage());
38 }
39 case 'e':// 退出
40 input.close();
41 loop = false;
42 break;
43 default:
44 break;
45 }
46 }
47 System.out.println("------exit------");
48
49 }
50 }
51
52 //使用数组模拟队列
53 class CircleArrayQueue {
54 private int maxSize;// 数组最大容量
55 private int front; // 指向队列头部
56 private int rear; // 队列尾部后一个元素,即 即将被叫号的那个元素
57 private int arr[]; // 该数组用于模拟队列存储数据
58
59 // 构造器
60 public CircleArrayQueue(int arrMaxSize) {
61 maxSize = arrMaxSize;
62 arr = new int[maxSize];
63 }
64 // 编写队列的方法
65 // 判断队列是否满
66 public boolean isFull() {
67 return (rear + 1) % maxSize == front;
68 }
69
70 // 判断队列是否为空
71 public boolean isEmpty() {
72 return rear == front;
73 }
74
75 // 添加队列
76 public void addQueue(int n) {
77 if (isFull()) {
78 System.out.println("队列已满,请稍后!");
79 return;
80 }
81 arr[rear] = n;
82 rear = (rear + 1) % maxSize;
83 }
84
85 // 获取数据
86 public int getQueue() {
87 if (isEmpty()) {
88 // 通过抛出异常处理
89 throw new RuntimeException("队列为空!");
90 }
91 int v = arr[front];
92 front = (front + 1) % maxSize;
93 return v;
94 }
95
96 // 遍历队列数据
97 public void showQueue() {
98 if (isEmpty()) {
99 System.out.println("队列为空!");
100 return;
101 }
102 for (int i = front; i < front + size(); i++) {
103 System.out.printf("arr[%d] = %d
", i % maxSize, arr[i % maxSize]);
104 }
105 }
106
107 // 返回当前数组有效数据数目
108 public int size() {
109 return (rear + maxSize - front) % maxSize;
110 }
111
112 // 展示队列头部信息
113 public int headQueue() {
114 if (isEmpty()) {
115 throw new RuntimeException("队列为空,无数据!");
116 }
117 return arr[front];
118 }
119 }