一维数组
冒泡排序
二维数组
Length:取数组元素的总个数
GetLength:取不同维度的个数
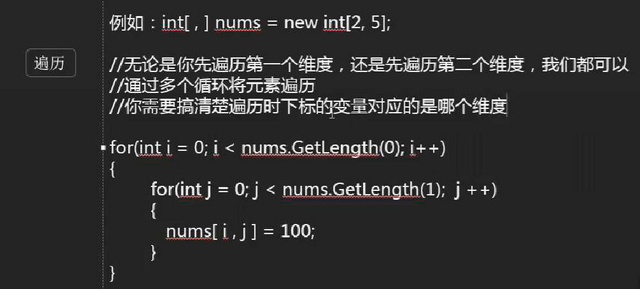
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace m1w2d5_array_rank { class Program { static void Main(string[] args) { #region 冒泡排序 //数组 冒泡排序 Random roll = new Random(); int[] array = { 10, 9, 7, 5, 3, 1, 2, 4, 8, 6 }; for (int i = 0; i < array.Length; i++) { array[i] = roll.Next(0, 100); } foreach (var item in array) { Console.Write(item + ","); } //子问题:指定一个区间,我可以把最大的放后面 //子问题的子问题:从头到尾遍历(除最后一个位置),相邻位置对比,如果大,就排后面 for (int i = 0; i < array.Length - 1; i++) { for (int j = 0; j < array.Length - 1 - i; j++) { if (array[j] > array[j + 1]) { int temp = array[j]; array[j] = array[j + 1]; array[j + 1] = temp; } } } //枚举器:可以帮我们快速遍历,使用这样的遍历方式,我们不用关心遍历的算法 //有一定的内存消耗 //如果他的成员是值类型不可以改,但是可以访问,但是可以改成员的成员,前提是成员是引用类型 Console.WriteLine(); foreach (var item in array) { Console.Write(item + ","); } //1、先写内循环,循环次数为:数组长度-1-外循环当前次数 //2、内循环每次对比当前位置与下一位置,如果逻辑(当前位大于对比位)达成 则交换位置 //3、外循环,循环次数为 数组长度-1 #endregion #region 二维数组 二维数组也是解决重复数据的重复逻辑,不同的是他允许我们通过两(多)个下标 为什么用多个下标,人们在生活,经常需要处理二维的数据,地图,表单(纯三维数据特别的少) 本质上,内存中一维和二维数组是一样的 使用二维数组 定义二维数组 不指定元素 数据类型[,] 变量名; 指定元素 数据类型[,] 变量名 = new 数据类型[标号为0维度的元素个数, 标号为1维度的元素个数] 数据类型[,] 变量名 = new 数据类型[行数, 列数] 数据类型[,] 变量名 = new 数据类型[x, y] 遍历 遍历二维数组我们需要有两个循环,每个循环处理不同维度 #endregion #region 二维数组的练习 //将1到24赋值给一个二维数组(4行6列) int[,] array = new int[4, 6]; int num = 1; //赋值正确 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { array[i, j] = num++; } } //显示正确 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { Console.Write(array[i, j] + " "); //Console.Write(array[i, j] + " "); } Console.WriteLine(); } //将二维数组(5行5列)的右上半部分置零. int[,] array = new int[5, 5]; int num = 1; //赋值正确 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { array[i, j] = num++; if (i < j) { array[i, j] = 0; } } } //显示正确 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { Console.Write(array[i, j] + " "); } Console.WriteLine(); } ////求二维数组(3行3列)的对角线元素的和. //00 01 02 //10 11 12 //20 21 22 int[,] array = new int[3, 3]; int num = 0; int sum = 0; //赋值正确 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { array[i, j] = num++; } } //i = j //i + j =array.GetLength(0)-1 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { if (i == j || i + j == 2) { sum += array[i, j]; } } } for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { Console.Write(array[i, j] + " "); } Console.WriteLine(); } Console.WriteLine(sum); ////求二维数组(5行5列)中最大元素值及其行列号。(随机1~500) Random roll = new Random(); int[,] array = new int[5, 5]; int max = 0; int x = 0; int y = 0; //赋值正确 for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { array[i, j] = roll.Next(1, 101); if (array[i, j] > max) { max = array[i, j]; x = i; y = j; } } } for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { Console.Write(array[i, j] + " "); } Console.WriteLine(); } Console.WriteLine("最大数为{0},行号为{1},列号位{2}", max, x + 1, y + 1); //从键盘上输入9个整数,保存在二维数组中按方阵的形式输出 int[,] array = new int[3, 3]; int num = 1; //赋值正确 Console.WriteLine("请输入10个整数"); for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { Console.WriteLine("请输入第{0}整数", num); num++; array[i, j] = int.Parse(Console.ReadLine()); } } for (int i = 0; i < array.GetLength(0); i++) { for (int j = 0; j < array.GetLength(1); j++) { Console.Write(array[i, j] + " "); } Console.WriteLine(); } #endregion #region 交错数组 //交错数组与多维数组的区别 //交错数组理论上才是数组的数组 //1、多维数组必须是每一个维度的元素个数相等,多维数组只能表示矩形数据块 //交错数组可以表示锯齿数据块 //2、多维数组必须是指定每一个维度的元素个数 //交错数组只能指定最外层的数组个数 //3、多维本质上是一块连续内存空间,为什么是多维表示,为了人们方便去理解,多维和一维只是索引器不一样 //交错数组理论上才是数组的数组 //定义一个交错数组 //数据类型[][]变量名; //数据类型[][]变量名 = new 数据类型[4][]{new int[10],new int[5],new int[10],new int[5],} //数据类型[][]变量名 = new 数据类型[4][]; int[][] array = new int[2][] { new int[4] { 1, 2, 3, 4 }, new int[2] { 8, 9 } }; //foreach (var item in array) //{ // for (int i = 0; i < item.Length; i++) // { // Console.Write(item[i] + " "); // } // Console.WriteLine(); //} foreach (var item in array) { foreach (var subitem in item) { Console.Write(subitem + " "); } Console.WriteLine(); } #endregion } } }
复习
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { //&&和&的区别 int a = 10; int b = 0; bool c = a > 15 && a / b > 0; Console.WriteLine(c);//结果false,只判断一遍 bool d = a > 15 & a / b > 0; Console.WriteLine(d);//结果报错,位运算符两边都会判断 float cost = 500; float _cost = cost < 300 ? 500 : 500 * 0.8f; string name = "老王"; name = name == "老王" ? "老王" : "流氓"; //90 A 90-100 //80 B //70 C //60 D //E Random r = new Random(); int score = r.Next(101); switch (score / 10) { case 10: //常量标号 字面值 case 9: Console.WriteLine("A"); break; case 8: Console.WriteLine("B"); break; case 7: Console.WriteLine("C"); break; case 6: Console.WriteLine("D"); break; default: Console.WriteLine("E"); break; } //0-10之间的偶数 int num = 0; while (true) { if (num == 0) { num++; continue; } if (num % 2 == 0) { Console.WriteLine(num); } num++; if (num > 10) { break; } } //数组 int[] num1 = { 1, 2, 3 };//静态数组,内存连续,大量读写效率高,长度不能动态增加,线性,内存连续 int[,] num2 = { { 1, 2, 3 }, { 4, 5, 6 } }; num2[1, 0] = 100; for (int i = 0; i < num2.GetLength(0); i++) { for (int j = 0; j < num2.GetLength(1); j++) { Console.WriteLine(num2[i,j] +" "); } Console.WriteLine(); } //标志枚举 EGameState status = EGameState.菜单状态; //0菜单状态,1战斗状态,2结束 switch (status) { case EGameState.菜单状态: Console.WriteLine("1.开始游戏 2.结束游戏"); char input = Console.ReadKey().KeyChar; if (input == 1) { } else if (input == 2) { } else { } break; case EGameState.游戏状态: break; case EGameState.结束状态: break; default: break; } ERollBuff buff = ERollBuff.None; buff = ERollBuff.中毒 | ERollBuff.减速 | ERollBuff.燃烧; if ((int)(buff & ERollBuff.中毒) != 0) { }//角色中毒 if ((int)(buff & ERollBuff.减速) != 0) { }//角色减速 if ((int)(buff & ERollBuff.燃烧) != 0) { } } } enum EGameState { 菜单状态,//0 游戏状态,//1 结束状态,//2 游戏简介 //3 } enum ERollBuff { None = 0, //0000 减速 = 1, //0001 衰弱 = 2, //0010 1<<1 枚举项多,可以用位左移 中毒 = 4, //0100 1<<2 燃烧 = 8 //1000 1<<3 } }