textfield截取字符串
ios7 会崩溃
解:
之前的写法是这样的
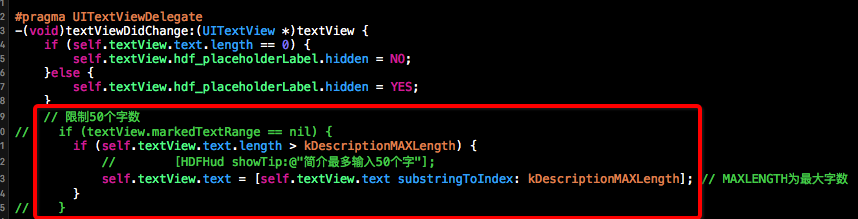
正确的写法:

先判断markedTextRange是否为nil, markedTextRange这个属性是啥意思呢

表示文件中当前选中的文本的长度,参考下面一片文章,详细讲解了这个属性的意思:
在解决上述问题的时候你需要判断markedTextRange是不是为Nil,如果为Nil的话就说明你现在没有未选中的字符,可以计算文字长度。否则此时计算出来的字符长度可能不正确
如果选中的长度为nil,即没有选中的长度,那么就正常计算字符串长度,如果超长就截取字符串
如果选中的长度不为nil,就是有选中的,那不用截取字符串,让他正常输入,超出了,真正往textFild填充的时候,, 选中的字符串的长度为nil了,这样就会走if判断,切割字符串
还写了下面的方法:

这个方法的作用是判断,是否需要修改text in range ,是否需要修改某一段文字, 如果不实现这个代理方法,在中文输入法下面,文字长度超出后,点击键盘,还是会联想字符串
里面的判断表示,如果没有选中的文本, 文本的总长度如果超出了限制,就不让它修改 return no
如果没有选中的文本,文本长度没有超出限制,可以修改 return yes
如果有选中的文本,让他修改 return yes
完整代码:
完整代码:
// // HDFWorkTableAddCheckItemView.h // newDoctor // // Created by songximing on 16/8/20. // Copyright © 2016年 haodf.com. All rights reserved. // #import <UIKit/UIKit.h> /** * @author songximing, 16-08-20 16:08:31 22:12 -2 * * 请输入检查项目View */ @interface HDFBenchAddCheckItemView : UIView - (void)show; @property (nonatomic, copy) HDFStringBlock saveStringBlock; //<!确定按钮保存的字符串 /** * @author songximing, 16-08-20 21:08:01 * * 请输入检查项目View 的初始化方法 * * @param frame 必须给frame(跟屏幕相等) * @param content 带入的文案内容 第一次进入没有传 nil * @param title 标题 "请输入检查项目" 或者 "请输入检验项目" 两个必须填写一个 * @param placeHolder 站位文字 必须传 * * @return self */ - (instancetype)initWithFrame:(CGRect)frame content:(NSString *)content title:(NSString *)title placeHolder:(NSString *)placeHolder; @property (nonatomic, copy) NSString *cancelString; //!<清空 @end
// // HDFWorkTableAddCheckItemView.m // newDoctor // // Created by songximing on 16/8/20. // Copyright © 2016年 haodf.com. All rights reserved. // #import "HDFBenchAddCheckItemView.h" #import "NSString+HDFAdditions.h" #define kDescriptionMAXLength 50 //最多50字 #define kTextViewStringWidth kScreenWidth - 50 - 30 // 50是白色View的左右间距 30是textView距离白色view的左右间距 #define kBenchBottomViewHeight 45.0f @interface HDFBenchAddCheckItemView ()<UITextViewDelegate> @property (nonatomic, strong) UITextView *textView; @property (nonatomic, strong) UIView *whiteContainerView; @property (nonatomic, strong) UIButton *cancelButton; @property (nonatomic, strong) UIControl *bgCoverControl; @property (nonatomic, copy) NSString *title; @property (nonatomic, copy) NSString *content; //!<带入的文本内容 @property (nonatomic, copy) NSString *placeHolder; //!<textView站位文字 @end @implementation HDFBenchAddCheckItemView - (void)dealloc { LOG(@"请输入检查项目View释放了..."); [[NSNotificationCenter defaultCenter] removeObserver:self name:UIKeyboardWillHideNotification object:nil]; [[NSNotificationCenter defaultCenter] removeObserver:self name:UIKeyboardWillShowNotification object:nil]; } - (instancetype)initWithFrame:(CGRect)frame content:(NSString *)content title:(NSString *)title placeHolder:(NSString *)placeHolder{ if (self = [super initWithFrame:frame]) { self.title = title; self.content = content; self.placeHolder = placeHolder; [self configUI]; } return self; } #pragma mark - UI - (void)configUI { [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillShow:) name:UIKeyboardWillShowNotification object:nil]; [[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardWillHide:) name:UIKeyboardWillHideNotification object:nil]; // 灰色遮罩 UIControl *bgCoverControl = [UIControl hdf_viewWithSuperView:self constraints:^(MASConstraintMaker *make) { make.edges.mas_equalTo(0); } tap:^(UIGestureRecognizer *sender) { // [self dismiss]; }]; bgCoverControl.backgroundColor = [UIColor colorWithRed:0 green:0 blue:0 alpha:0]; self.bgCoverControl = bgCoverControl; // 白色背景 UIView *whiteContainerView = [UIView hdf_viewWithSuperView:self constraints:^(MASConstraintMaker *make) { make.centerY.mas_equalTo(0); make.centerX.mas_equalTo(0); make.width.mas_equalTo(270); }]; whiteContainerView.backgroundColor = kWColor; whiteContainerView.alpha = 0; whiteContainerView.layer.cornerRadius = 8; whiteContainerView.clipsToBounds = YES; self.whiteContainerView = whiteContainerView; // title UILabel *titleLabel = [UILabel hdf_labelWithText:@"请输入检查项目" font:kFontWithSize(17) superView:whiteContainerView constraints:^(MASConstraintMaker *make) { make.top.mas_equalTo(25); make.centerX.mas_equalTo(0); }]; titleLabel.textAlignment = NSTextAlignmentCenter; if (self.title.length > 0) { // 设置标题 titleLabel.text = self.title; } // 文本输入框 self.textView = [UITextView hdf_textViewWithPlaceholder:@" " delegate:self superView:whiteContainerView constraints:^(MASConstraintMaker *make) { make.top.mas_equalTo(titleLabel.mas_bottom).offset(15); make.left.mas_equalTo(15); make.right.mas_equalTo(-15); make.height.mas_equalTo(kFontWithSize(19).lineHeight * 4); }]; self.textView.layer.cornerRadius = 3; self.textView.clipsToBounds = YES; self.textView.layer.borderWidth = 0.5; self.textView.layer.borderColor = kColorWith16RGB(0xcccccc).CGColor; self.textView.hdf_placeholder = @"请输入检查项目"; self.textView.backgroundColor = kColorWith16RGB(0xf8f8f8); self.textView.font = kFontWithSize(16); self.textView.hdf_placeholderFont = kFontWithSize(16); self.textView.hdf_placeholderLabel.numberOfLines = 0; self.textView.hdf_placeholderColor = [UIColor redColor]; [self.textView.hdf_placeholderLabel mas_updateConstraints:^(MASConstraintMaker *make) { make.top.mas_equalTo(self.textView).offset(7.5); make.left.mas_equalTo(self.textView).offset(5); make.width.mas_equalTo(self.textView.mas_width).offset(-5); // 让站位文字换行 }]; if (self.content.length > 0) { // 带入的文本内容 self.textView.text = self.content; self.textView.hdf_placeholder = self.placeHolder; self.textView.hdf_placeholderLabel.hidden = YES; }else { // 无内容,展示站位文字 self.textView.hdf_placeholder = self.placeHolder; self.textView.hdf_placeholderLabel.hidden = NO; } // // textView高度自适应内容 // CGSize textSize = CGSizeZero; // if (isIOS(7.0)) { // textSize = [self.textView sizeThatFits:CGSizeMake(kTextViewStringWidth, MAXFLOAT)]; // } // else{ // textSize = self.textView.contentSize; // } // if (kTextViewHeight < textSize.height) { // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) { // make.height.mas_equalTo(textSize.height); // }]; // }else { // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) { // make.height.mas_equalTo(kTextViewHeight); // }]; // } [self createBottomView]; } - (void)createBottomView { UIView *bottomBgView = [[UIView alloc]init]; [self.whiteContainerView addSubview:bottomBgView]; [bottomBgView mas_makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(self.textView.mas_bottom).offset(15); make.left.right.equalTo(self.whiteContainerView); make.height.mas_equalTo(kBenchBottomViewHeight); }]; // 水平分割线 UIView *horizontalLineView = [[UIView alloc]init]; [bottomBgView addSubview:horizontalLineView]; horizontalLineView.backgroundColor = kG5Color; [horizontalLineView mas_makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(bottomBgView); make.left.right.equalTo(bottomBgView); make.height.mas_equalTo(kLineHeight); }]; // 垂直分割线 UIView *verticalLineView = [[UIView alloc]init]; [bottomBgView addSubview:verticalLineView]; verticalLineView.backgroundColor = kG5Color; [verticalLineView mas_makeConstraints:^(MASConstraintMaker *make) { make.top.bottom.equalTo(bottomBgView); make.centerX.equalTo(bottomBgView); make.width.mas_equalTo(kLineHeight); }]; kWeakObject(self) // 取消 按钮 UIButton *cancelButton = [UIButton hdf_buttonWithTitle:@"取消" superView:bottomBgView constraints:^(MASConstraintMaker *make) { make.top.equalTo(horizontalLineView.mas_bottom); make.left.bottom.equalTo(bottomBgView); make.right.equalTo(verticalLineView.mas_left); } touchup:^(UIButton *sender) { [weakObject cancelButtonClick]; }]; [cancelButton setTitleColor:kNavColer forState:UIControlStateNormal]; self.cancelButton = cancelButton; UIButton *confirmButton = [UIButton hdf_buttonWithTitle:@"确定" superView:bottomBgView constraints:^(MASConstraintMaker *make) { make.top.equalTo(horizontalLineView.mas_bottom); make.right.bottom.equalTo(bottomBgView); make.left.equalTo(verticalLineView.mas_right); } touchup:^(UIButton *sender) { [weakObject confirmButtonClick]; }]; [confirmButton setTitleColor:kNavColer forState:UIControlStateNormal]; [self.whiteContainerView mas_makeConstraints:^(MASConstraintMaker *make) { make.bottom.equalTo(bottomBgView); }]; } #pragma mark - 点击事件 - (void)confirmButtonClick { // 去掉首尾的空格 self.textView.text = [self.textView.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceCharacterSet]]; // if (self.textView.text.length < 1) { // [HDFHud showTip:@"请先输入检查项目"]; // return; // } [self dismiss]; if (self.saveStringBlock) { self.saveStringBlock(self.textView.text); } } - (void)cancelButtonClick { // self.textView.text = nil; // 点击取消,清空输入的文字 [self dismiss]; // if (self.saveStringBlock) { // self.saveStringBlock(self.textView.text); // } } #pragma UITextViewDelegate -(void)textViewDidChange:(UITextView *)textView { if (self.textView.text.length == 0) { self.textView.hdf_placeholderLabel.hidden = NO; }else { self.textView.hdf_placeholderLabel.hidden = YES; } // 限制50个字数 if (textView.markedTextRange == nil) { if (self.textView.text.length > kDescriptionMAXLength) { // [HDFHud showTip:@"简介最多输入50个字"]; self.textView.text = [self.textView.text substringToIndex: kDescriptionMAXLength]; // MAXLENGTH为最大字数 } } // // textView高度自适应内容 // CGSize textSize = CGSizeZero; // if (isIOS(7.0)) { // textSize = [self.textView sizeThatFits:CGSizeMake(kTextViewStringWidth, MAXFLOAT)]; // } // else{ // textSize = self.textView.contentSize; // } // // if (kTextViewHeight < textSize.height) { // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) { // make.height.mas_equalTo(textSize.height); // }]; // [UIView animateWithDuration:0.25 animations:^{ // [self layoutIfNeeded]; // }]; // }else { // [self.textView mas_updateConstraints:^(MASConstraintMaker *make) { // make.height.mas_equalTo(kTextViewHeight); // }]; // } } - (BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text{ if (textView.markedTextRange == nil) { if (range.location >= kDescriptionMAXLength) { return NO; } } return YES; } #pragma mark - 键盘相关 // Handle keyboard show/hide changes - (void)keyboardWillShow: (NSNotification *)notification { CGSize keyboardSize = [[[notification userInfo] objectForKey:UIKeyboardFrameBeginUserInfoKey] CGRectValue].size; UIInterfaceOrientation interfaceOrientation = [[UIApplication sharedApplication] statusBarOrientation]; if (UIInterfaceOrientationIsLandscape(interfaceOrientation) && NSFoundationVersionNumber <= NSFoundationVersionNumber_iOS_7_1) { CGFloat tmp = keyboardSize.height; keyboardSize.height = keyboardSize.width; keyboardSize.width = tmp; } [self.whiteContainerView mas_updateConstraints:^(MASConstraintMaker *make) { make.centerY.mas_equalTo(-keyboardSize.height * 0.5); }]; [UIView animateWithDuration:0.2f delay:0.0 options:UIViewAnimationOptionTransitionNone animations:^{ [self layoutIfNeeded]; } completion:nil ]; } - (void)keyboardWillHide: (NSNotification *)notification { [self.whiteContainerView mas_updateConstraints:^(MASConstraintMaker *make) { make.centerY.mas_equalTo(0); }]; [UIView animateWithDuration:0.2f delay:0.0 options:UIViewAnimationOptionTransitionNone animations:^{ [self layoutIfNeeded]; } completion:nil ]; } - (void)show { [[UIApplication sharedApplication].keyWindow addSubview:self]; //动画 CGAffineTransform transform = CGAffineTransformMakeScale(1.2, 1.2); self.whiteContainerView.transform = transform; [UIView animateWithDuration:0.2 animations:^{ self.bgCoverControl.backgroundColor = [UIColor colorWithRed:0 green:0 blue:0 alpha:0.5]; self.whiteContainerView.alpha = 1; // 子控件的透明度跟着变化 CGAffineTransform transform = CGAffineTransformMakeScale(1, 1); self.whiteContainerView.transform = transform; }]; } - (void)dismiss { [UIView animateWithDuration:0.2 animations:^{ self.bgCoverControl.backgroundColor = [UIColor colorWithRed:0 green:0 blue:0 alpha:0]; self.whiteContainerView.alpha = 0; } completion:^(BOOL finished) { [self removeFromSuperview]; }]; } -(void)setCancelString:(NSString *)cancelString { _cancelString = cancelString; [self.cancelButton setTitle:cancelString forState:UIControlStateNormal]; } //****以下为解决第一次进入页面,textview不可滚动问题 - (UIView *)hitTest:(CGPoint)point withEvent:(UIEvent *)event { NSString * strText = self.textView.text; CGFloat h = [strText heightWithFont:[UIFont systemFontOfSize:16] withLineWidth:kTextViewStringWidth]; self.textView.contentSize = CGSizeMake(0, h+10); return [super hitTest:point withEvent:event]; } @end