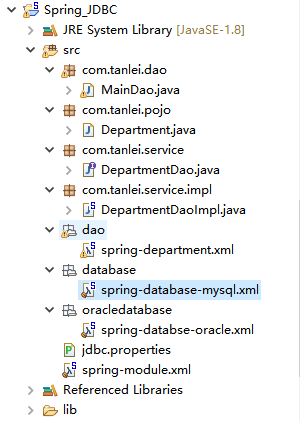
1.导入jarbao
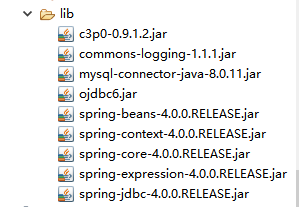
2.创建pojo,dao,Impl

package com.tanlei.pojo;
public class Department {
private Long deptId;
private String deptNo;
private String deptName;
public Department() {
super();
}
public Department(Long deptId, String deptNo, String deptName) {
super();
this.deptId = deptId;
this.deptNo = deptNo;
this.deptName = deptName;
}
public Long getDeptId() {
return deptId;
}
public void setDeptId(Long deptId) {
this.deptId = deptId;
}
public String getDeptNo() {
return deptNo;
}
public void setDeptNo(String deptNo) {
this.deptNo = deptNo;
}
public String getDeptName() {
return deptName;
}
public void setDeptName(String deptName) {
this.deptName = deptName;
}
@Override
public String toString() {
return "Department [deptId=" + deptId + ", deptNo=" + deptNo + ", deptName=" + deptName + "]";
}
}
View Code

package com.tanlei.service;
import java.util.List;
import com.tanlei.pojo.Department;
public interface DepartmentDao {
public List<Department> queryDepartment() throws Exception;
}
View Code

package com.tanlei.service.impl;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import javax.sql.DataSource;
import com.tanlei.pojo.Department;
import com.tanlei.service.DepartmentDao;
public class DepartmentDaoImpl implements DepartmentDao {
public DataSource datasource;
public void setDatasource(DataSource datasource) {
this.datasource = datasource;
}
@Override
public List<Department> queryDepartment() throws Exception {
//从数据源获取连接
Connection connection=datasource.getConnection();
//执行数据库操作
Statement statement=connection.createStatement();
//根据sql语句返回结果集
String sql="Select d.dept_id, d.dept_no, d.dept_name from department d";
ResultSet rSet=statement.executeQuery(sql);
List<Department>list =new ArrayList<Department>();
while(rSet.next()) {
Long deptId=rSet.getLong("dept_id");
String deptNo=rSet.getString("dept_no");
String deptName=rSet.getString("dept_name");
Department department=new Department(deptId, deptNo, deptName);
list.add(department);
}
return list;
}
}
View Code
3.创建配置文件及bean文件及数据源文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="departmentDAO" class="com.tanlei.service.impl.DepartmentDaoImpl">
<property name="datasource" ref="dataSource"></property>
</bean>
</beans>
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<!-- 导入属性文件 -->
<context:property-placeholder location="classpath:jdbc.properties"/>
<!-- Spring配置数据源 -->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="user" value="${user}"></property>
<property name="password" value="${password}"></property>
<property name="driverClass" value="${driverClass}"></property>
<property name="jdbcUrl" value="${jdbcUrl}"></property>
</bean>
</beans>
#mysql
user=root
password=password
driverClass=com.mysql.jdbc.Driver
jdbcUrl=jdbc:mysql://localhost:3306/mysql?serverTimezone=GMT%2B8
#oracle
#user=scott
#password=tiger
#driverClass=oracle.jdbc.OracleDriver
#jdbcUrl=jdbc:oracle:thin:@localhost:1521:ORCL
4.配置主配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--调用数据源集bean的配置文件xml -->
<import resource="dao/spring-department.xml" />
<!-- mysql -->
<import resource="database/spring-database-mysql.xml"/>
<!-- oracle -->
<!-- <import resource="oracledatabase/spring-databse-oracle.xml" /> -->
</beans>
5.创建运行类
package com.tanlei.dao;
import java.util.List;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.tanlei.pojo.Department;
import com.tanlei.service.DepartmentDao;
public class MainDao {
public static void main(String[] args) throws Exception {
ApplicationContext context=new ClassPathXmlApplicationContext("spring-module.xml");
DepartmentDao departmentDao=(DepartmentDao) context.getBean("departmentDAO");
List<Department> departments=departmentDao.queryDepartment();
for (Department department : departments) {
System.out.println(department.toString());
System.out.println(department.getDeptId());
}
}
}
6.运行结果
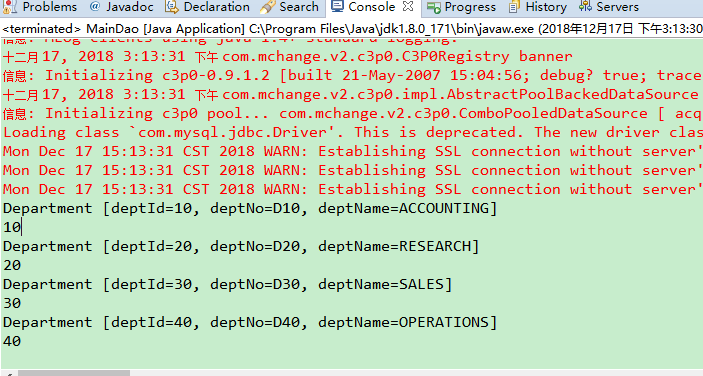