介绍
本文介绍如何通过Telnet协议连接到远程Shell,执行脚本,并获取执行结果;
相关文章:
《【Jsch】使用SSH协议连接到远程Shell执行脚本》http://www.cnblogs.com/ssslinppp/p/6244653.html
其他示例:
maven仓库
使用Apache Commons-net通用库;
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.4</version>
</dependency>
《Apache Commons Net示例》http://commons.apache.org/proper/commons-net/
包括:
- FTP/FTPS
- FTP over HTTP (experimental)
- NNTP
- SMTP(S)
- POP3(S)
- IMAP(S)
- Telnet
- TFTP
- Finger
- Whois
- rexec/rcmd/rlogin
- Time (rdate) and Daytime
- Echo
- Discard
- NTP/SNTP
- Backgr
具体步骤
- 步骤1: 使用TelnetClient创建连接:connect();
- 步骤2: 设置Telnet属性:如 回显选项/SUPPRESS GO AHEAD/终端类型等;
- 步骤3: 获取输入/输出流:getInputStream()/getOutputStream();
- 步骤4: 使用username和password进行登录;
- 步骤5: 执行Shell脚本,获取执行结果;
- 步骤6: 关闭资源:输入/输出流,TelnetClient连接等;
程序
步骤1~步骤6

执行具体脚本
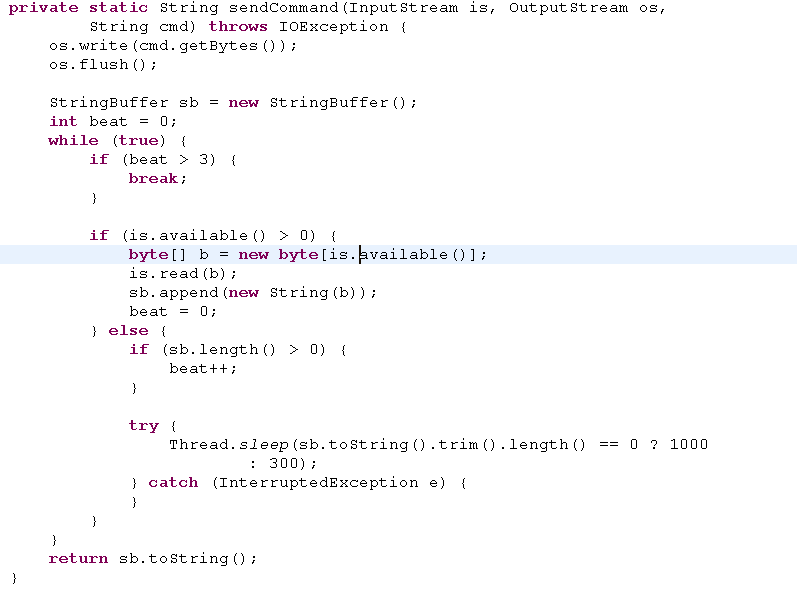
测试程序
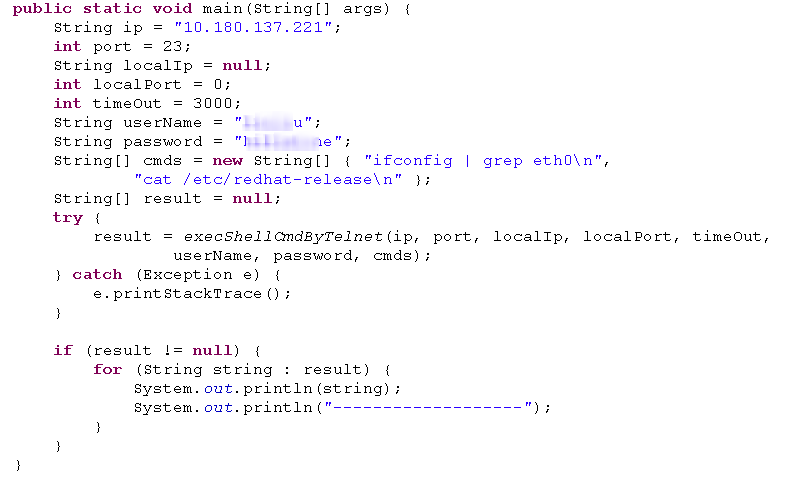
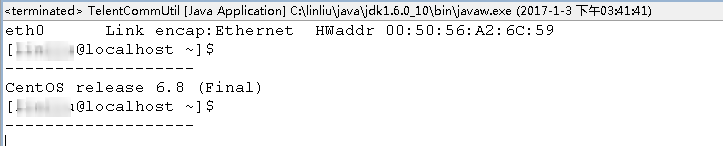
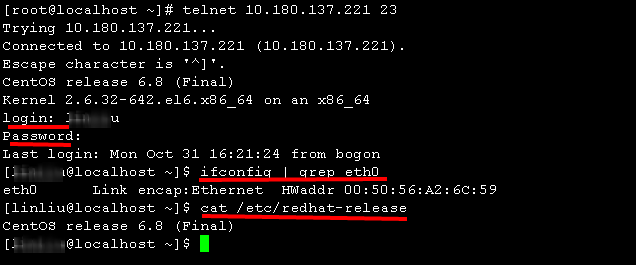
完整程序
package com.sssppp.Communication;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.SocketTimeoutException;
import org.apache.commons.net.telnet.EchoOptionHandler;
import org.apache.commons.net.telnet.SuppressGAOptionHandler;
import org.apache.commons.net.telnet.TelnetClient;
import org.apache.commons.net.telnet.TerminalTypeOptionHandler;
public class TelentCommUtil {
/**
* 测试程序
*
* @param args
*/
public static void main(String[] args) {
String ip = "10.180.137.221";
int port = 23;
String localIp = null;
int localPort = 0;
int timeOut = 3000;
String userName = "xxxxx";
String password = "xxxxx";
String[] cmds = new String[] { "ifconfig | grep eth0 ",
"cat /etc/redhat-release " };
String[] result = null;
try {
result = execShellCmdByTelnet(ip, port, localIp, localPort, timeOut,
userName, password, cmds);
} catch (Exception e) {
e.printStackTrace();
}
if (result != null) {
for (String string : result) {
System.out.println(string);
System.out.println("-------------------");
}
}
}
/**
* 使用Telnet协议,连接到Linux Shell,执行脚本命令,并获取结果
*
* @param dstIp
* @param dstPort
* @param localIp
* @param localPort
* @param timeOut
* @param userName
* @param password
* @param cmds
* @return
* @throws Exception
*/
public static String[] execShellCmdByTelnet(String dstIp, int dstPort,
String localIp, int localPort, int timeOut, String userName,
String password, String... cmds) throws Exception {
TelnetClient tc = new TelnetClient();
InputStream is = null;
OutputStream os = null;
try {
//设置:RFC 1091 TELNET终端类型选项
tc.addOptionHandler(new TerminalTypeOptionHandler("VT100", false,
false, true, false));
//设置:RFC 857 TELNET ECHO 回显选项
tc.addOptionHandler(new EchoOptionHandler(true, false, true, false));
//设置:RFC 858 TELNET SUPPRESS GO AHEAD(抑制继续进行)选项
tc.addOptionHandler(new SuppressGAOptionHandler(true, true, true,
true));
tc.setConnectTimeout(timeOut);
if (localIp == null) {
tc.connect(dstIp, dstPort);
} else {
tc.connect(InetAddress.getByName(dstIp), dstPort,
InetAddress.getByName(localIp), localPort);
}
is = tc.getInputStream();
os = tc.getOutputStream();
//输入用户名和密码
if (sendCommand(is, os, " ").contains("login:")) {
if (sendCommand(is, os, userName + " ").contains("assword:")) {
if (sendCommand(is, os, password + " ").contains(
"incorrect")) {
throw new Exception("Auth error");
}
}
}
String[] result = new String[cmds.length];
for (int i = 0; i < cmds.length; i++) {
result[i] = sendCommand(is, os, cmds[i]);
}
return result;
} catch (SocketTimeoutException e) {
throw new Exception("SocketTimeoutException error");
} catch (Exception e) {
throw e;
} finally {
try {
is.close();
} catch (Exception e) {
}
try {
os.close();
} catch (Exception e) {
}
try {
tc.disconnect();
} catch (IOException e) {
}
}
}
/**
* 执行Shell命令,并获取执行结果
*
* @param is
* @param os
* @param cmd
* @return
* @throws IOException
*/
private static String sendCommand(InputStream is, OutputStream os,
String cmd) throws IOException {
os.write(cmd.getBytes());
os.flush();
StringBuffer sb = new StringBuffer();
int beat = 0;
while (true) {
if (beat > 3) {
break;
}
if (is.available() > 0) {
byte[] b = new byte[is.available()];
is.read(b);
sb.append(new String(b));
beat = 0;
} else {
if (sb.length() > 0) {
beat++;
}
try {
Thread.sleep(sb.toString().trim().length() == 0 ? 1000
: 300);
} catch (InterruptedException e) {
}
}
}
return sb.toString();
}
}