1. Description:

2. Examples:
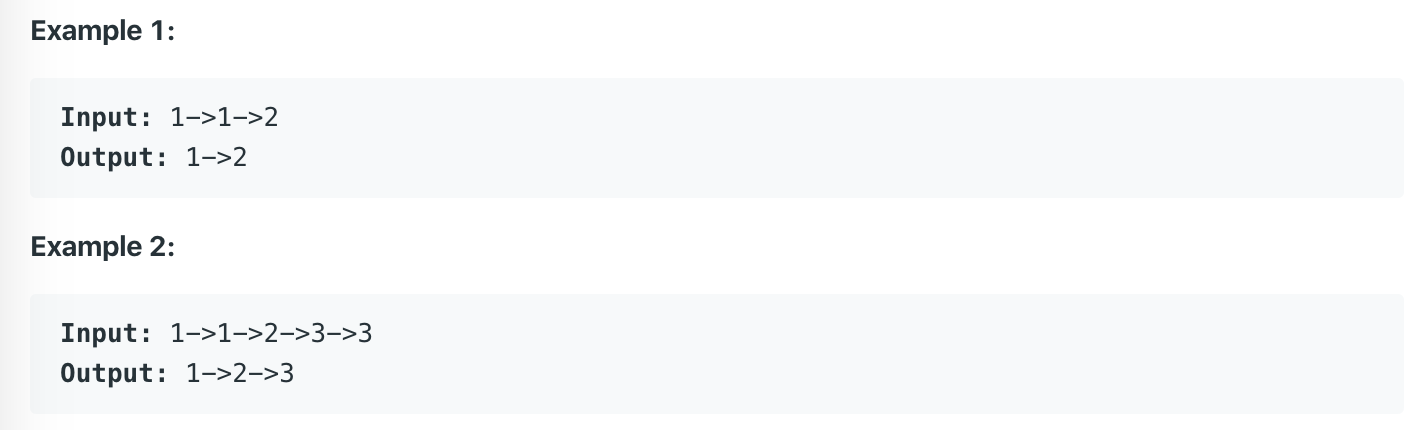
3.Solutions:
1 /**
2 * Created by sheepcore on 2019-05-08
3 * Definition for singly-linked list.
4 * public class ListNode {
5 * int val;
6 * ListNode next;
7 * ListNode(int x) { val = x; }
8 * }
9 */
10 class Solution {
11 public ListNode deleteDuplicates(ListNode head) {
12 if(head == null)
13 return head;
14 else{
15 ListNode pre = head, cur = head.next;
16 while (cur != null){
17 if(cur.val == pre.val){
18 pre.next = cur.next;
19 cur = cur.next;
20 } else{
21 pre = cur;
22 cur = cur.next;
23 }
24 }
25 }
26 return head;
27
28 }
29 }