原题
Description
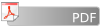
A ring is composed of n (even number) circles as shown in diagram. Put natural numbers into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
Input
n (0 < n <= 16)Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements.
You are to write a program that completes above process.
Sample Input
6 8
Sample Output
Case 1: 1 4 3 2 5 6 1 6 5 2 3 4 Case 2: 1 2 3 8 5 6 7 4 1 2 5 8 3 4 7 6 1 4 7 6 5 8 3 2 1 6 7 4 3 8 5 2
分析:
题目可以使用dfs求解
这一编写一个高效的判断素数的函数 如下
int is_prime(int x) {
for (int i = 2; i*i <= x; i++)
if (x % i == 0) return 0;
return 1;
}
我的代码

#include<cstdio> #include<cstring> #include<algorithm> using namespace std; int is_prime(int x) { for (int i = 2; i*2 <= x; i++) if (x % i == 0) return 0; return 1; } int n, A[50], isp[50], vis[50]; void dfs(int cur) { if (cur == n && isp[A[0] + A[n - 1]]) { for (int i = 0; i < n; i++) { if (i != 0) printf(" "); printf("%d", A[i]); } printf(" "); } else for (int i = 2; i <= n; i++) if (!vis[i] && isp[i + A[cur - 1]]) { A[cur] = i; vis[i] = 1; dfs(cur + 1); vis[i] = 0; } } int main() { int kase = 0; while (scanf("%d", &n) == 1 && n > 0) { if (kase > 0) printf(" "); printf("Case %d: ", ++kase); for (int i = 2; i <= n * 2; i++) isp[i] = is_prime(i); memset(vis, 0, sizeof(vis)); A[0] = 1; dfs(1); } return 0; }