3211: 花神游历各国
Time Limit: 5 Sec Memory Limit: 128 MBSubmit: 2549 Solved: 946
[Submit][Status][Discuss]
Description
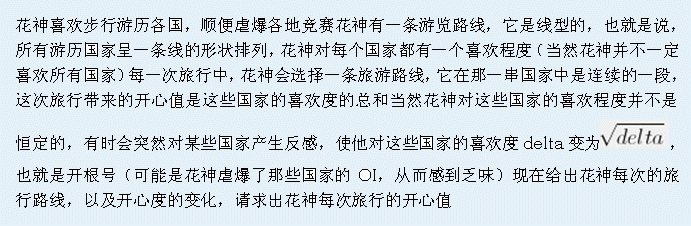
Input
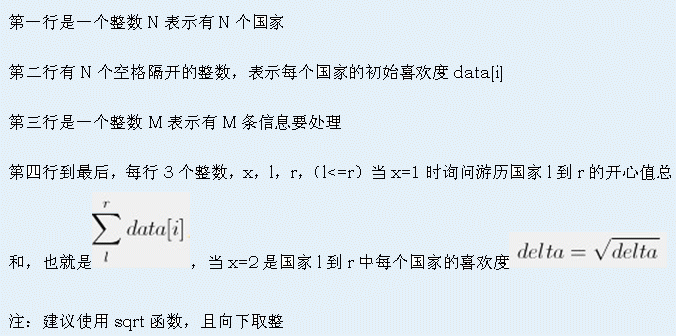
Output
每次x=1时,每行一个整数,表示这次旅行的开心度
Sample Input
4
1 100 5 5
5
1 1 2
2 1 2
1 1 2
2 2 3
1 1 4
1 100 5 5
5
1 1 2
2 1 2
1 1 2
2 2 3
1 1 4
Sample Output
101
11
11
11
11
HINT
对于100%的数据, n ≤ 100000,m≤200000 ,data[i]非负且小于10^9
Solution
区间开根号看似十分难处理,实际不妨找规律。data[ i ] 的值最大为109。
( 109 )½ = 105
( ( 109 )½)½ = 103
( ( ( 109 )½ )½ )½ = 102
( ( ( ( 109 )½ )½ )½ )½ = 101
( ( ( ( ( 109 )½ )½ )½ )½ )½ = 1
一个很大的数,五次根号后全部是1,当某个区间的值被开过5次根号,那么该区间所有值都是1.
根据以上结论,不难写出代码。

1 /************************************************************** 2 Problem: 3211 3 User: shadowland 4 Language: C++ 5 Result: Accepted 6 Time:1568 ms 7 Memory:40372 kb 8 ****************************************************************/ 9 10 #include "bits/stdc++.h" 11 12 using namespace std ; 13 typedef long long QAQ ; 14 struct SegTree { int l , r , sqr ; QAQ sum ; } ; 15 const int maxN = 500100 ; 16 17 SegTree tr[ maxN << 2 ] ; 18 19 void Push_up ( const int i ) { 20 tr[ i ].sum = tr[ i << 1 ].sum + tr[ i << 1 | 1 ].sum ; 21 } 22 23 int INPUT ( ) { 24 int x = 0 , f = 1 ; char ch = getchar ( ) ; 25 while ( ch < '0' || ch > '9' ) { if ( ch == '-' ) f = - 1 ; ch = getchar ( ) ; } 26 while ( ch >= '0' && ch <='9' ) { x = ( x << 1 ) + ( x << 3 ) + ch - '0' ; ch = getchar ( ) ; } 27 return x * f ; 28 } 29 30 void Build_Tree ( const int x , const int y , const int i ) { 31 tr[ i ].l = x ; tr[ i ].r = y ; 32 if ( x == y ) tr[ i ].sum = INPUT ( ) ; 33 else { 34 int mid = ( tr[ i ].l + tr[ i ].r ) >> 1 ; 35 Build_Tree ( x , mid , i << 1 ) ; 36 Build_Tree ( mid + 1 , y , i << 1 | 1 ) ; 37 Push_up ( i ) ; 38 } 39 } 40 void Update_Tree ( const int q , const int w , const int i ) { 41 if ( q <= tr[ i ].l && tr[ i ].r <= w ) { 42 ++ tr[ i ].sqr ; 43 if ( tr[ i ].l == tr[ i ].r ) { 44 tr[ i ].sum = sqrt ( tr[ i ].sum ) ; 45 return ; 46 } 47 } 48 49 int mid = ( tr[ i ].l + tr[ i ].r ) >> 1 ; 50 if ( tr[ i ].sqr <= 5 ) { 51 if ( q > mid ) Update_Tree ( q , w , i << 1 | 1 ) ; 52 else if ( w <= mid ) Update_Tree ( q , w , i << 1 ) ; 53 else { 54 Update_Tree ( q , w , i << 1 | 1 ) ; 55 Update_Tree ( q , w , i << 1 ) ; 56 } 57 Push_up ( i ) ; 58 } 59 60 } 61 62 QAQ Query_Tree ( const int q , const int w , const int i ) { 63 if ( q <= tr[ i ].l && tr[ i ].r <= w ) { 64 return tr[ i ].sum ; 65 } 66 else { 67 int mid = ( tr[ i ].l + tr[ i ].r ) >> 1 ; 68 if ( q > mid ) return Query_Tree ( q , w , i << 1 | 1) ; 69 else if ( w <= mid ) return Query_Tree ( q , w , i << 1 ) ; 70 else return Query_Tree ( q , w , i << 1 | 1 ) + Query_Tree ( q , w , i << 1 ) ; 71 } 72 } 73 74 int main ( ) { 75 int N = INPUT ( ) ; 76 Build_Tree ( 1 , N , 1 ) ; 77 int Q = INPUT( ) ; 78 while ( Q-- ) { 79 int op = INPUT ( ) ; 80 if( op == 1 ) { 81 int l = INPUT( ) , r = INPUT( ) ; 82 printf ( "%lld " , Query_Tree ( l , r , 1 ) ) ; 83 } 84 else if ( op == 2 ) { 85 int l = INPUT ( ) , r = INPUT ( ) ; 86 Update_Tree ( l , r , 1 ) ; 87 } 88 } 89 return 0 ; 90 }
2016-10-13 22:10:52
(完)