UVA122 树的层次遍历 Trees on the level
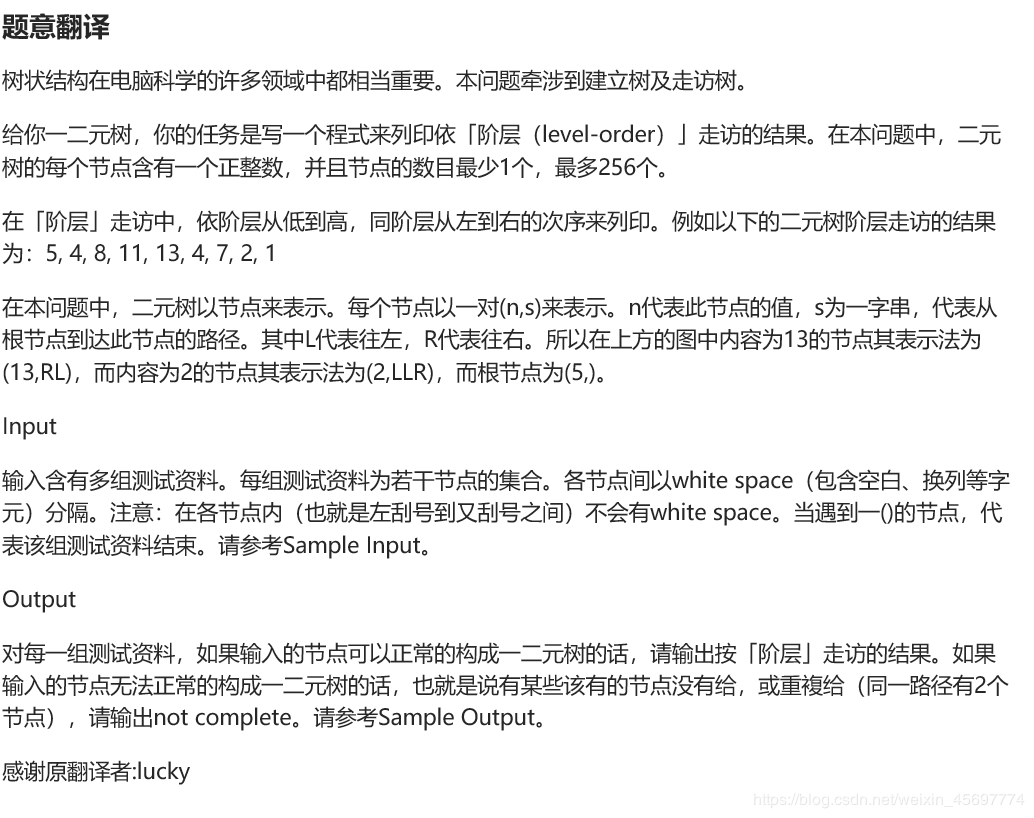
//二叉树 用数组或者动态链表
#include<bits/stdc++>
using namespace std;
const int maxn=100010;
char s[maxn];
bool failed;
vector<int> ans;
struct Node
{
bool have_value; //该节点是否有值
int v; //节点值
Node *left;
Node *right;
//有点像构造函数
Node():have_value(false),left(NULL),right(NULL){};
};
Node *root;
Node *newnode()
{
return new Node(); //开辟新节点
}
void addnode(int v,char *s)
{
int n=strlen(s);
Node *u=root;
for(int i=0;i<n;i++)
{
if(s[i]=='L')
{
if(u->left == NULL)
u->left=newnode(); // 若给的位置没有节点,创造新的
u=u->left; // 找到给的位置节点u
}
else if(s[i]=='R')
{
if(u->right==NULL)
u->right=newnode();
u=u->right;
}
}
if(u->have_value)
{
failed=true;
}
u->v=v; //赋值
u->have_value = true; // 此节点有值
}
bool read_input()
{
failed=false;
root = newnode();
for(;;)
{
if(scanf("%s",s)!=1)
return false;
if(!strcmp(a,"()"))
break;
int v;
//把值记录在v中
sscanf(&s[1],"%d",&v);
addnode(v,strchr(s,',')+1); // 从,后开始调用函数
return true;
}
}
bool bfs(vector<int> &ans) //遍历二叉树,宽度优先遍历
{
queue<Node*> q;
ans.clear();
q.push(root); //先放根节点
while(!q.empty())
{
Node *u=q.front();
q.pop();
if(!u->have_value)
return false;
ans.push_back(u->v);
if(u->left != NULL)
q.push(u->left); // 有左儿子放左儿子入队
if(u->right != NULL)
q.push(u->right); // 有右儿子放右儿子入队
}
return true;
}
int main()
{
while(read_input()) //读入正确
{
if(bfs(ans)&&!failed) //正确
{
for(int i = 0 ; i < ans.size() ; i++) // 输出ans
{
if(i == 0?printf("%d",ans[i]):printf(" %d",ans[i]));
}
cout << endl;
}
else
cout << "not complete" << endl;
}
return 0;
}