
RMI远程调用步骤:
1,客户对象调用客户端辅助对象上的方法
2,客户端辅助对象打包调用信息(变量,方法名),通过网络发送给服务端辅助对象
3,服务端辅助对象将客户端辅助对象发送来的信息解包,找出真正被调用的方法以及该方法所在对象
4,调用真正服务对象上的真正方法,并将结果返回给服务端辅助对象
5,服务端辅助对象将结果打包,发送给客户端辅助对象
6,客户端辅助对象将返回值解包,返回给客户对象
7,客户对象获得返回值
对于客户对象来说,步骤2-6是完全透明的

IService接口:
import java.rmi.Remote; import java.rmi.RemoteException;
public interface IService extends Remote { //声明服务器端必须提供的服务 String service(String content) throws RemoteException; }
创建远程方法接口,该接口必须继承自Remote接口
Remote 接口是一个标识接口,用于标识所包含的方法可以从非本地虚拟机上调用的接口,Remote接口本身不包含任何方法
由于远程方法调用的本质依然是网络通信,只不过隐藏了底层实现,网络通信是经常会出现异常的,所以接口的所有方法都必须抛出RemoteException以说明该方法是有风险的。
ServiceImpl实现类:
import java.rmi.RemoteException; //UnicastRemoteObject用于导出的远程对象和获得与该远程对象通信的存根。 import java.rmi.server.UnicastRemoteObject; public class ServiceImpl extends UnicastRemoteObject implements IService { private String name; /** * 必须定义构造方法,即使是默认构造方法,也必须把它明确地写出来,因为它必须抛出出RemoteException异常 */ public ServiceImpl(String name) throws RemoteException { this.name = name; } @Override public String service(String content) { return "server >> " + content; } }
创建远程方法接口实现类:
UnicastRemoteObject类的构造函数抛出了RemoteException,故其继承类不能使用默认构造函数,继承类的构造函数必须也抛出RemoteException
由于方法参数与返回值最终都将在网络上传输,故必须是可序列化的
Server类:
/** * Context接口表示一个命名上下文,它由一组名称到对象的绑定组成。 * 它包含检查和更新这些绑定的一些方法。 */ import javax.naming.Context; /* * InitialContext类是执行命名操作的初始上下文。 * 该初始上下文实现 Context 接口并提供解析名称的起始点。 */ import javax.naming.InitialContext; public class Server { public static void main(String[] args) { try { // 实例化实现了IService接口的远程服务ServiceImpl对象 IService service02 = new ServiceImpl("service02"); // 初始化命名空间 Context namingContext = new InitialContext(); /** * Naming 类提供在对象注册表中存储和获得远程对远程对象引用的方法 Naming 类的每个方法都可将某个名称作为其一个参数, * 该名称是使用以下形式的 URL 格式(没有 scheme 组件)的 java.lang.String: * //host:port/name host:注册表所在的主机(远程或本地),省略则默认为本地主机 * port:是注册表接受调用的端口号,省略则默认为1099,RMI注册表registry使用的著名端口 * name:是未经注册表解释的简单字符串 */ // 将名称绑定到对象,即向命名空间注册已经实例化的远程服务对象 namingContext.rebind("rmi://localhost/service02", service02); } catch (Exception e) { e.printStackTrace(); } System.out.println("服务器向命名表注册了1个远程服务对象!"); } }
Client类:
import javax.naming.Context; import javax.naming.InitialContext; public class Client { public static void main(String[] args) { String url = "rmi://localhost/"; try { Context namingContext = new InitialContext(); // 检索指定的对象。 即找到服务器端相对应的服务对象存根 IService service02 = (IService) namingContext.lookup(url + "service02"); Class stubClass = service02.getClass(); System.out.println(service02 + " 是 " + stubClass.getName() + " 的实例!"); // 获得本底存根已实现的接口类型 Class[] interfaces = stubClass.getInterfaces(); for (Class c : interfaces) { System.out.println("存根类实现了 " + c.getName() + " 接口!"); } System.out.println(service02.service("你好!")); } catch (Exception e) { e.printStackTrace(); } } }
执行步骤:
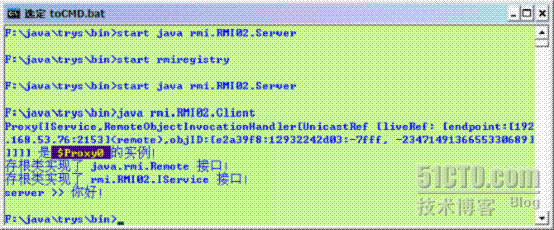
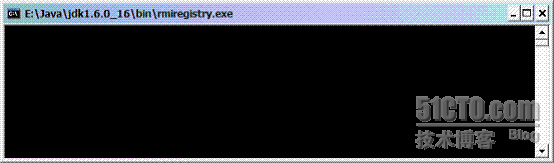

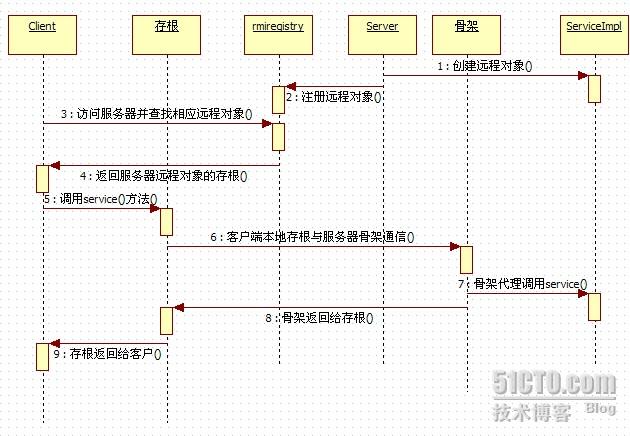
参考复制来源:
http://haolloyin.blog.51cto.com/1177454/332426
http://blog.csdn.net/a19881029/article/details/9465663
http://damies.iteye.com/blog/51778
http://www.blogjava.net/zhenyu33154/articles/320245.html