
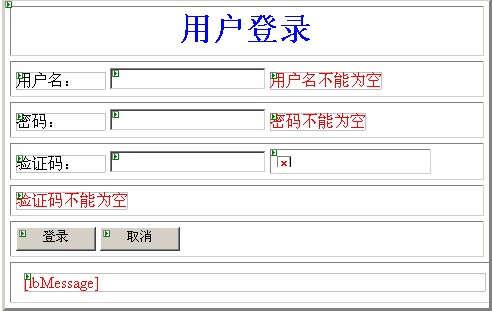
1
2
3 using System;
4 using System.Data;
5 using System.Configuration;
6 using System.Collections;
7 using System.Web;
8 using System.Web.Security;
9 using System.Web.UI;
10 using System.Web.UI.WebControls;
11 using System.Web.UI.WebControls.WebParts;
12 using System.Web.UI.HtmlControls;
13
14
15
16 public partial class _Default : System.Web.UI.Page
17 {
18
19 private static string sValidator = "";
20 private readonly string sValidatorImageUrl = "ValidateImage.aspx?Validator=";
21
22 private static char[] allCharArray = new char[] { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9' };
23
24
25
26 protected void Page_Load(object sender, EventArgs e)
27 {
28 this.tbUserName.Focus();
29
30 if (!Page.IsPostBack)
31 {
32 sValidator=CreateValidateString(6);
33
34 Application["validateString"] = sValidator;
35
36 this.imgValidator.ImageUrl=sValidatorImageUrl+sValidator;
37 }
38 }
39
40 //随机生成验证码,并把验证码保存到Session中
41 private string CreateValidateString(int nLen)
42 {
43 #region 0-9数字的验证码
44
45 string randomCode = "";
46 int t = 0;
47
48 Random rand = new Random();
49
50 for (int i = 0; i < nLen; i++)
51 {
52 t = rand.Next(9);
53
54 randomCode += allCharArray[t];
55 }
56
57 Session["CheckCode"] = randomCode;
58
59 return randomCode;
60
61 #endregion
62 }
63
64 protected void btnCancel_Click(object sender, EventArgs e)
65 {
66 this.tbUserName.Text = "";
67 this.tbPassword.Text = "";
68 this.lbMessage.Text = "";
69
70
71 sValidator = CreateValidateString(6);
72
73 Application["validateString"] = sValidator;
74
75 this.imgValidator.ImageUrl = sValidatorImageUrl + sValidator;
76
77
78
79 this.imgValidator.ImageUrl = sValidatorImageUrl + sValidator;
80
81 }
82
83 protected void btnLogin_Click(object sender, EventArgs e)
84 {
85 if (Page.IsValid == true)
86 {
87 if (this.tbValidator.Text != sValidator)
88 {
89 lbMessage.Text = "验证码输入错误,请重新输入验证码!!!";
90
91 sValidator = CreateValidateString(6);
92
93 Application["validateString"] = sValidator;
94
95 this.imgValidator.ImageUrl = sValidatorImageUrl + sValidator;
96
97 return;
98 }
99 else
100 {
101 Response.Write("congradulations!");
102 }
103 }
104
105 }
106
107 }
2
3 using System;
4 using System.Data;
5 using System.Configuration;
6 using System.Collections;
7 using System.Web;
8 using System.Web.Security;
9 using System.Web.UI;
10 using System.Web.UI.WebControls;
11 using System.Web.UI.WebControls.WebParts;
12 using System.Web.UI.HtmlControls;
13
14
15
16 public partial class _Default : System.Web.UI.Page
17 {
18
19 private static string sValidator = "";
20 private readonly string sValidatorImageUrl = "ValidateImage.aspx?Validator=";
21
22 private static char[] allCharArray = new char[] { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9' };
23
24
25
26 protected void Page_Load(object sender, EventArgs e)
27 {
28 this.tbUserName.Focus();
29
30 if (!Page.IsPostBack)
31 {
32 sValidator=CreateValidateString(6);
33
34 Application["validateString"] = sValidator;
35
36 this.imgValidator.ImageUrl=sValidatorImageUrl+sValidator;
37 }
38 }
39
40 //随机生成验证码,并把验证码保存到Session中
41 private string CreateValidateString(int nLen)
42 {
43 #region 0-9数字的验证码
44
45 string randomCode = "";
46 int t = 0;
47
48 Random rand = new Random();
49
50 for (int i = 0; i < nLen; i++)
51 {
52 t = rand.Next(9);
53
54 randomCode += allCharArray[t];
55 }
56
57 Session["CheckCode"] = randomCode;
58
59 return randomCode;
60
61 #endregion
62 }
63
64 protected void btnCancel_Click(object sender, EventArgs e)
65 {
66 this.tbUserName.Text = "";
67 this.tbPassword.Text = "";
68 this.lbMessage.Text = "";
69
70
71 sValidator = CreateValidateString(6);
72
73 Application["validateString"] = sValidator;
74
75 this.imgValidator.ImageUrl = sValidatorImageUrl + sValidator;
76
77
78
79 this.imgValidator.ImageUrl = sValidatorImageUrl + sValidator;
80
81 }
82
83 protected void btnLogin_Click(object sender, EventArgs e)
84 {
85 if (Page.IsValid == true)
86 {
87 if (this.tbValidator.Text != sValidator)
88 {
89 lbMessage.Text = "验证码输入错误,请重新输入验证码!!!";
90
91 sValidator = CreateValidateString(6);
92
93 Application["validateString"] = sValidator;
94
95 this.imgValidator.ImageUrl = sValidatorImageUrl + sValidator;
96
97 return;
98 }
99 else
100 {
101 Response.Write("congradulations!");
102 }
103 }
104
105 }
106
107 }
108
109 //***************************************************************************
110 using System;
111 using System.Data;
112 using System.Configuration;
113 using System.Collections;
114 using System.Web;
115 using System.Web.Security;
116 using System.Web.UI;
117 using System.Web.UI.WebControls;
118 using System.Web.UI.WebControls.WebParts;
119 using System.Web.UI.HtmlControls;
120
121 using System.Drawing;
122 using System.Drawing.Imaging;
123
124
125 public partial class ValidateImage : System.Web.UI.Page
126 {
127
128 #region 设置验证码
129 private int codeLen = 6;//验证码长度
130
131 private int fineness = 90;//图片清晰度
132
133 private int imgWidth = 60;//图片宽度
134
135 private int imgHeight = 18;//图片高度
136
137 private string fontFamily = "Arial";//字体家庭名称
138
139 private int fontSize = 11;//字体大小
140
141 private int fontStyle = 1;//字体样式
142
143 private int posX = 5;//绘制起始坐标X
144
145 private int posY = 2;//绘制起始坐标Y
146
147 //private static char[] allCharArray = new char[] { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9' };
148 #endregion
149
150 protected void Page_Load(object sender, EventArgs e)
151 {
152 #region 读取Request 传递参数
153 //获取代码长度设置
154 if (Request["CodeLen"] != null)
155 {
156 try
157 {
158 codeLen = Int32.Parse(Request["CodeLen"]);
159 //规定验证码长度
160 if (codeLen < 4 || codeLen > 16)
161 {
162 throw new Exception("验证码长度必须在4到16之间");
163 }
164
165 }
166 catch (Exception Ex)
167 {
168 throw Ex;
169 }
170 }
171
172 //获取图片清晰度设置
173 if (Request["Fineness"] != null)
174 {
175 try
176 {
177 fineness = Int32.Parse(Request["Fineness"]);
178
179 //验证清晰度
180 if (fineness < 0 || fineness > 100)
181 throw new Exception("图片清晰度必须在0到100之间");
182 }
183 catch (Exception Ex)
184 {
185 throw Ex;
186 }
187 }
188
189 //获取图片宽度
190 if (Request["ImgWidth"] != null)
191 {
192 try
193 {
194 imgWidth = Int32.Parse(Request["ImgWidth"]);
195
196 if (imgWidth < 16 || imgWidth > 480)
197 throw new Exception("图片宽度必须在16到480之间");
198 }
199 catch (Exception Ex)
200 {
201 throw Ex;
202 }
203 }
204
205
206 //获取图片高度
207 if (Request["ImgHeight"] != null)
208 {
209 try
210 {
211 imgWidth = Int32.Parse(Request["ImgHeight"]);
212
213 if (imgWidth < 16 || imgWidth > 320)
214 throw new Exception("图片高度必须在16到320之间");
215 }
216 catch (Exception Ex)
217 {
218 throw Ex;
219 }
220 }
221
222
223 //获取验证码字体家族名称
224 if (Request["FontFamily"] != null)
225 {
226 fontFamily = Request["FontFamily"];
227 }
228
229
230
231 //获取验证码字体大小
232 if (Request["FontSize"] != null)
233 {
234 try
235 {
236 fontSize = Int32.Parse(Request["FontSize"]);
237
238 if (fontSize < 8 || fontSize > 72)
239 {
240 throw new Exception("字体大小必须在8到72之间");
241 }
242 }
243 catch (Exception Ex)
244 {
245 throw Ex;
246 }
247 }
248
249
250 //获取字体样式
251 if (Request["FontStyle"] != null)
252 {
253 try
254 {
255 fontStyle = Int32.Parse(Request["FontStyle"]);
256 }
257 catch (Exception Ex)
258 {
259 throw Ex;
260 }
261 }
262
263 //验证码绘制起始位置X
264 if (Request["PosX"] != null)
265 {
266 try
267 {
268 posX = Int32.Parse(Request["PosX"]);
269 }
270 catch (Exception Ex)
271 {
272 throw Ex;
273 }
274 }
275
276 //验证码绘制起始位置Y
277 if (Request["PosY"] != null)
278 {
279 try
280 {
281 posY = Int32.Parse(Request["PosY"]);
282 }
283 catch (Exception Ex)
284 {
285 throw Ex;
286 }
287 }
288 #endregion 读取Request 传递参数
289
290 //string validateCode = CreateValidateCode();
291
292 string validateCode = Application["validateString"].ToString();
293
294
295 //生成Bitmap图像
296 Bitmap bitmap = new Bitmap(imgWidth, imgHeight);
297
298 //给图像设置干扰
299 DisturbBitmap(bitmap);
300
301 //绘制验证码
302 DrawValidateCode(bitmap, validateCode);
303
304 //保存验证码图像,等待输出
305 bitmap.Save(Response.OutputStream, ImageFormat.Gif);
306
307 bitmap.Dispose();
308 }
309
310 ////随机生成验证码,并把验证码保存到Session中
311 //private string CreateValidateCode()
312 //{
313 // #region 0-9数字的验证码
314
315 // string randomCode = "";
316 // int t = 0;
317
318 // Random rand = new Random();
319
320 // for (int i = 0; i < codeLen; i++)
321 // {
322 // t = rand.Next(9);
323
324 // randomCode += allCharArray[t];
325 // }
326
327 // Session["CheckCode"] = randomCode;
328
329 // return randomCode;
330
331 // #endregion
332 //}
333
334 //为图片设置干扰
335 private void DisturbBitmap(Bitmap bitmap)
336 {
337 //通过随机数生成
338 Random random = new Random();
339
340 for (int i = 0; i < bitmap.Width; i++)
341 {
342 for (int j = 0; j < bitmap.Height; j++)
343 {
344 if (random.Next(100) <= this.fineness)
345 bitmap.SetPixel(i, j, Color.White);
346 }
347 }
348 }
349
350
351 //绘制验证码图片
352 private void DrawValidateCode(Bitmap bitmap, string validateCode)
353 {
354 //获取绘制器对象
355
356 Graphics g = Graphics.FromImage(bitmap);
357
358 //绘制字体
359 Font font = new Font(fontFamily, fontSize, GetFontStyle());
360
361 //绘制验证码图像
362 g.DrawString(validateCode, font, Brushes.Black, posX, posY);
363
364 font.Dispose();
365 g.Dispose();
366 }
367
368
369 //换算验证码字体样式:1粗体 2斜体 3粗斜体,默认为普通字体
370
371 private FontStyle GetFontStyle()
372 {
373 if (fontStyle == 1)
374 {
375 return FontStyle.Bold;
376 }
377 else if (fontStyle == 2)
378 {
379 return FontStyle.Italic;
380 }
381 else
382 {
383 return FontStyle.Regular;
384 }
385 }
386 }
387