1.Application.java
package com.niugang; import org.apache.catalina.connector.Connector; import org.apache.coyote.http11.AbstractHttp11Protocol; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.context.embedded.tomcat.TomcatConnectorCustomizer; import org.springframework.boot.context.embedded.tomcat.TomcatEmbeddedServletContainerFactory; import org.springframework.boot.web.support.SpringBootServletInitializer; import org.springframework.context.annotation.Bean; //解决包tomcat冲突 //@EnableAutoConfiguration(exclude={WebMvcAutoConfiguration.class}) //组件扫描,会自动扫描springboot启动类包及其子包下的注解文件 //@ComponentScan("com.niugang.controller") //springboot注解 //springboot1.2+之后用@SpringBootApplication替代了三个注解 @SpringBootApplication //mapper 接口类扫描包配置 @MapperScan(value={"com.niugang.dao"}) public class Application extends SpringBootServletInitializer{ public static void main(String[] args) { SpringApplication.run(Application.class,args); } //Tomcat large file upload connection reset @Bean public TomcatEmbeddedServletContainerFactory tomcatEmbedded() { TomcatEmbeddedServletContainerFactory tomcat = new TomcatEmbeddedServletContainerFactory(); tomcat.addConnectorCustomizers(new MyTomcatConnectorCustomizer()); return tomcat; } class MyTomcatConnectorCustomizer implements TomcatConnectorCustomizer{ @SuppressWarnings("rawtypes") @Override public void customize(Connector connector) { AbstractHttp11Protocol protocolHandler = (AbstractHttp11Protocol)connector.getProtocolHandler(); protocolHandler.setMaxSwallowSize(-1); } } }
上面代码事为了解决
tomcatEmbedded这段代码是为了解决上传文件大于10M出现连接重置的问题。此异常内容GlobalException也捕获不到
2.UploadController.java
package com.niugang.controller; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.util.FileCopyUtils; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.multipart.MultipartFile; /** * 文件上传 * * @author niugang * */ @Controller public class UploadController { @RequestMapping("toupload") public String toUpload() { return "upload"; } @RequestMapping("upload") public String upload(MultipartFile file, ModelMap map, HttpServletRequest request) { if (file.isEmpty()) { map.put("uploadResult", "文件不能为空"); return "upload"; } File filePath = new File("d:/myweb/upload"); if (!filePath.exists()) { filePath.mkdirs(); } File realPath = new File(filePath + "/" + file.getOriginalFilename()); try { /* * 或者这样 * File name = new File(file.getOriginalFilename()); *file.transferTo(name); */ FileCopyUtils.copy(file.getInputStream(), new FileOutputStream(realPath)); map.put("uploadResult", "文件上传成功"); } catch (IllegalStateException | IOException e) { e.printStackTrace(); } return "upload"; } /** * * @return 第二种方式 * @throws IOException */ @RequestMapping(value = "/upload1", method = RequestMethod.POST) @ResponseBody public String upload1(MultipartFile file) throws IOException { byte[] bytes = file.getBytes(); //这样默认上传文件就放在当前 项目路径下 File name = new File(file.getOriginalFilename()); FileCopyUtils.copy(bytes, name); return name.getAbsolutePath(); } }
3.application.properties
#文件上传相关配置 #org.springframework.boot.autoconfigure.web.MultipartProperties 具体配置类 #spring.http.multipart.enabled=true #默认支持文件上传. #spring.http.multipart.file-size-threshold=0 #支持文件写入磁盘. # 上传文件的临时目录 #spring.http.multipart.location= # 最大支持文件大小 spring.http.multipart.max-file-size=2Mb #默认为1m #spring.http.multipart.max-request-size=10Mb # 最大支持请求大小 默认为10m
4.FileUploadException.java 文件上传错误提示
package com.niugang.exception; import org.springframework.boot.autoconfigure.web.MultipartProperties; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.multipart.MultipartException; import org.springframework.web.servlet.ModelAndView; /** * @ControllerAdvice * 它通常用于定义{@link ExceptionHandler @ExceptionHandler}、 * {@link InitBinder @InitBinder}和{@link ModelAttribute @ModelAttribute} * * 方法适用于所有{@link RequestMapping @RequestMapping}方法。 * * @author niugang 文件上传异常处理类 * */ @ControllerAdvice /** * 从配置文件根据前缀读取设置 处理需要字段外还需要,set方法,否则值注入不进来 * * @author niugang * */ @ConfigurationProperties(prefix = "spring.http.multipart") public class FileUploadException { private String maxFileSize; public String getMaxFileSize() { return maxFileSize; } public void setMaxFileSize(String maxFileSize) { this.maxFileSize = maxFileSize; } @ExceptionHandler(MultipartException.class) public ModelAndView handleError1(MultipartException e) { ModelAndView modelAndView = new ModelAndView("upload"); // 如果application.properties里面没有设置,则读取系统默认的文件大小 if (this.getMaxFileSize() == null) { MultipartProperties multipartProperties = new MultipartProperties(); modelAndView.addObject("uploadResult", "最大上传为:" + multipartProperties.getMaxFileSize()); } else { modelAndView.addObject("uploadResult", "最大上传为:" + this.getMaxFileSize()); } return modelAndView; } }
5.upload.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> <h1>Spring Boot文件上传</h1> <#if uploadResult??> ${uploadResult} </#if> <form method="POST" action="upload" enctype="multipart/form-data"> <input type="file" name="file" /><br /> <br /> <input type="submit" value="提交" /> </form> </body> </html>
6.启动项目
http://localhost:8080/myweb/toupload
文件上传成功:
文件上传失败:
微信公众号
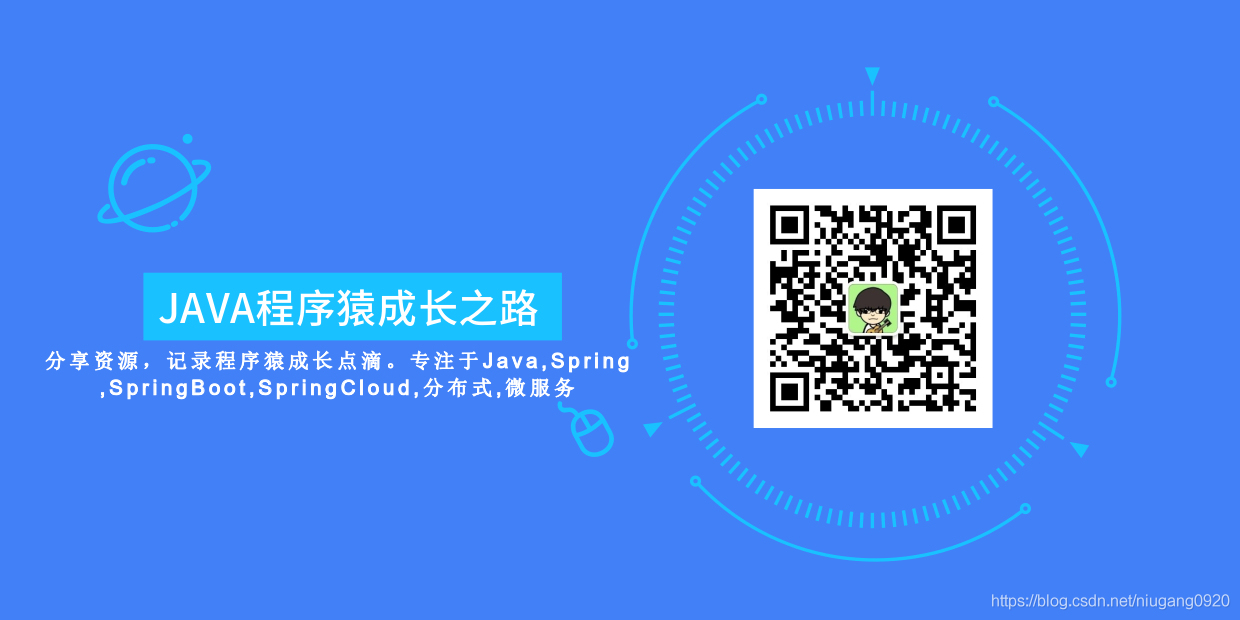