示意图:
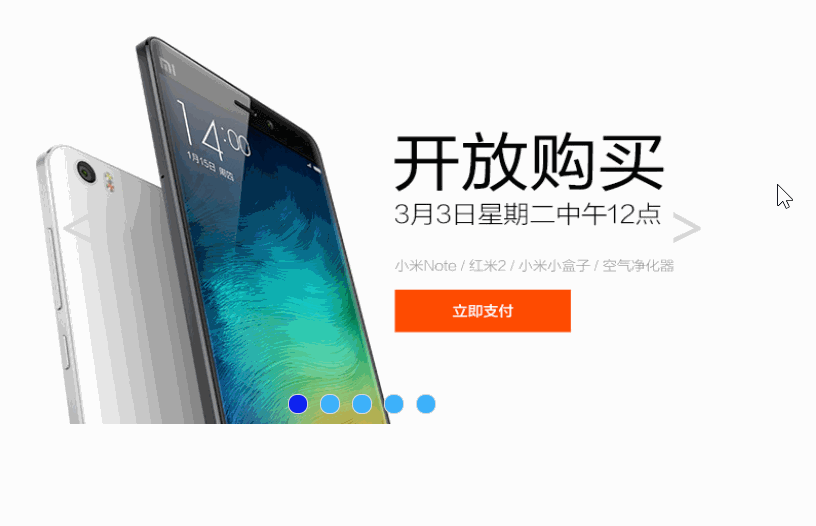
demo
1 <!DOCTYPE html>
2 <html lang="en">
3 <head>
4 <meta charset="UTF-8">
5 <title>首页</title>
6 <style>
7 *{margin:0px;padding:0px;}
8 .wrap{700px;height:400px;margin:50px auto 0;position: relative;}
9 .imgList img{700px;height:400px;position: absolute;left:0px;top:0px;}
10 .btn {200px;height:20px;position: absolute;bottom: 10px;right:250px;}
11 .btn ul li{18px;height:18px;border: 1px solid #ddd;list-style: none;border-radius: 9px;float: left;margin:0 6px;
12 background: #3DB1FA;color: #ddd;text-align: center;cursor: pointer;}
13 .btn ul li:hover{background: #0e23f0}
14 .btn ul li.hov{background: #0e23f0}
15 .btnL{position: absolute;left:20px;top:150px;50px;height:100px;line-height: 100px;font-size: 60px;color: #ddd;text-align: center;cursor: pointer}
16 .btnR{position: absolute;right:20px;top:150px;50px;height:100px;line-height: 100px;font-size: 60px;color: #ddd;text-align: center;cursor: pointer;}
17 .btnL:hover,.btnR:hover{background: rgba(0,0,0,.3)}
18 </style>
19 </head>
20 <body>
21 <div class="wrap">
22 <div class="imgList">
23 <img src="img/1.jpg" alt="" style="display: block">
24 <img src="img/2.jpg" alt="" style="display: none">
25 <img src="img/3.jpg" alt="" style="display: none">
26 <img src="img/4.jpg" alt="" style="display: none">
27 <img src="img/5.jpg" alt="" style="display: none">
28 </div>
29 <div class="btn">
30 <ul>
31 <li class="hov"></li>
32 <li></li>
33 <li></li>
34 <li></li>
35 <li></li>
36 </ul>
37 </div>
38 <div class="btnL"><</div>
39 <div class="btnR">></div>
40 </div>
41 <script src="../lib/jquery-1.12.2.js"></script>
42 <script>
43 var timer = null;
44 var _index = 0;
45
46
47 //小圆点hover切换
48 $('.btn>ul>li').hover(function () {
49 clearInterval(timer);
50 _index = $(this).index();
51 changImg(_index);
52 },function () {
53 autoPlay();
54 });
55
56 //左右切图
57 $('.btnR').click(function () {
58 clearInterval(timer);
59 _index++;
60 if(_index >4) {
61 _index = 0;
62 }
63 changImg(_index);
64 });
65
66 $('.btnL').click(function () {
67 clearInterval(timer);
68 _index--;
69 if(_index < 0) {
70 _index = 4;
71 }
72 changImg(_index);
73
74 });
75 $('.btnR,.btnL').hover(function () {
76 clearInterval(timer);
77 },function () {
78 autoPlay();
79 });
80
81 function changImg(_index) {
82 $('.btn>ul>li').eq(_index).addClass('hov').siblings('li').removeClass('hov');
83 $('.imgList>img').eq(_index).stop().fadeIn().siblings('img').stop().hide();
84 }
85 // 自动轮播
86 function autoPlay() {
87 timer = setInterval(function () {
88 _index++;
89 if(_index >4) {
90 _index = 0;
91 }
92 changImg(_index);
93 },2000);
94 }
95
96 autoPlay();
97
98 </script>
99 </body>
100 </html>